JavaScript Spread Operator

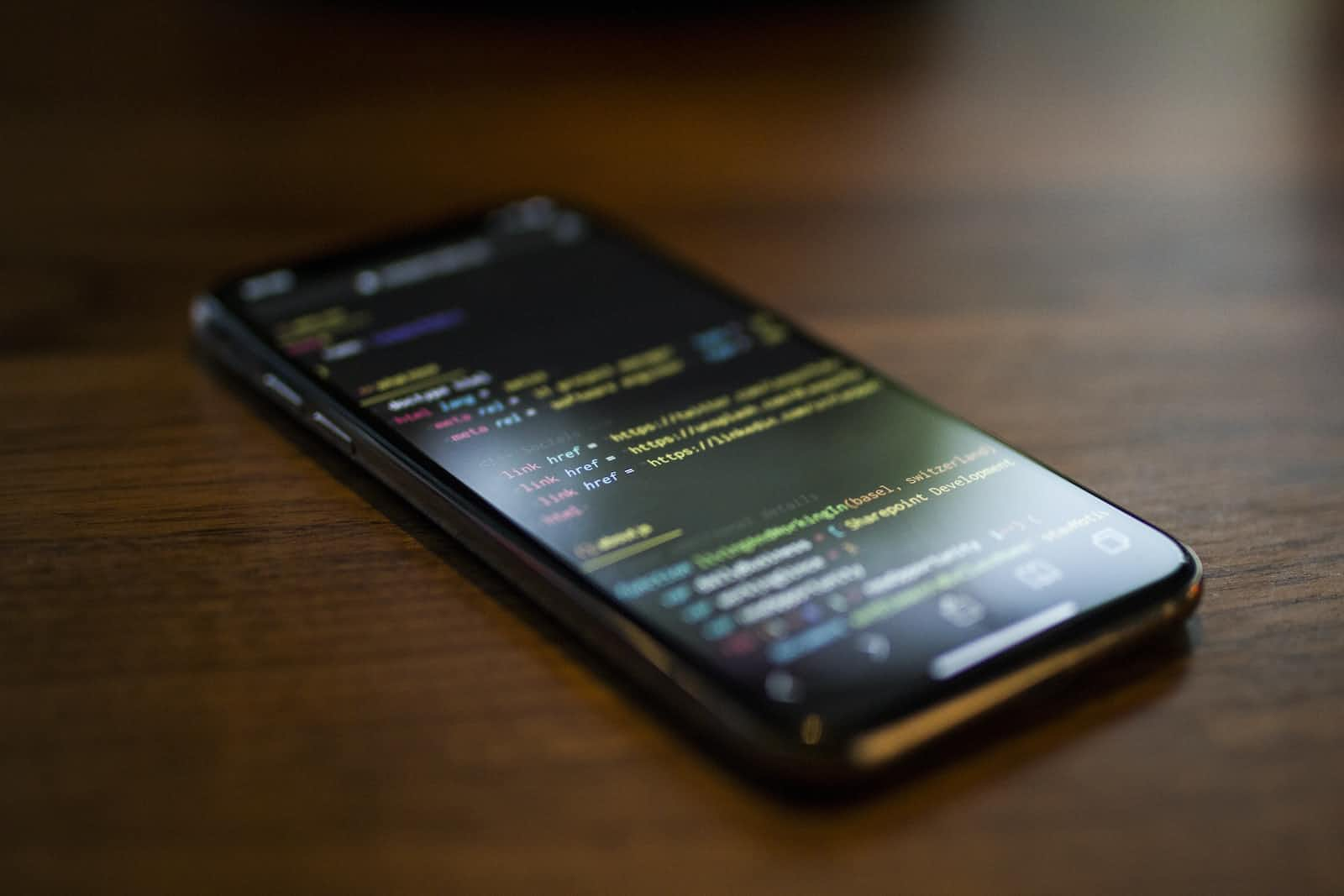
For understanding the spread operator and rest operator ,lets us understand first Destructing in JavaScript.
Destructuring assignment
In JavaScript, "destructuring" is a way to extract values from arrays or objects and assign them to variables in a more concise way. It's a shorthand for writing multiple assignments in one line.
The destructuring assignment syntax is a JavaScript expression that makes it possible to unpack values from arrays, or properties from objects, into distinct variables.
For example, let's say we have an array with three elements:
javascriptCopy codeconst myArray = [1, 2, 3];
Instead of assigning each element to a separate variable like this:
javascriptCopy codeconst firstElement = myArray[0];
const secondElement = myArray[1];
const thirdElement = myArray[2];
We can use destructuring to assign all three elements to variables at once like this:
javascriptCopy codeconst [firstElement, secondElement, thirdElement] = myArray;
Similarly, we can also destructure objects. Let's say we have an object with two properties:
javascriptCopy codeconst myObject = { name: "John", age: 30 };
Instead of accessing each property using dot notation like this:
javascriptCopy codeconst name = myObject.name;
const age = myObject.age;
We can use destructuring to assign both properties to variables at once like this:
javascriptCopy codeconst { name, age } = myObject;
Spread Operator (...)
In JavaScript, the spread operator is denoted by three dots (...
) and it is used to spread the elements of an iterable object (like an array or a string) into individual elements.
The spread operator is commonly used in array manipulation. For example, let's say we have two arrays:
javascriptCopy codeconst arr1 = [1, 2, 3];
const arr2 = [4, 5, 6];
If we want to concatenate these arrays into a new array, we can use the spread operator like this:
javascriptCopy codeconst newArr = [...arr1, ...arr2];
The result will be a new array containing all the elements from arr1
and arr2
:
csharpCopy code[1, 2, 3, 4, 5, 6]
The spread operator can also be used to make a copy of an array, like this:
javascriptCopy codeconst originalArr = [1, 2, 3];
const copyArr = [...originalArr];
The copyArr
will be a new array with the same elements as originalArr
.
The spread operator can also be used to convert an iterable object into an array. For example, if we have a string, we can use the spread operator to convert it into an array of characters:
rustCopy codeconst str = "Hello";
const arr = [...str];
The arr
will be an array containing each character of the string:
cssCopy code["H", "e", "l", "l", "o"]
Subscribe to my newsletter
Read articles from Lovely Sehotra directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by
