Constructor Function in JavaScript
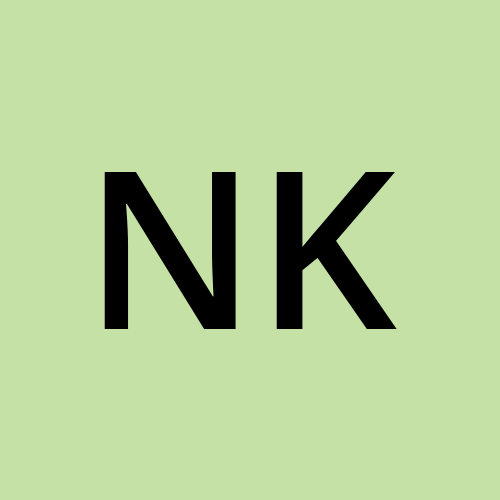
The constructor function is used to create a set of properties and methods for an object that we might want to use over and over again. It is used to avoid repetition and to increase the readability of the code.
For example, details of the student.
Code, if the Constructor function is not used then the code will look something like this
var student1 = {
name: "John",
age: 15,
classNo: 9
};
var student2 = {
name: "Rahul",
age: 16,
classNo: 10
};
console.log(student1.name); // Provides the name of the student1
console.log(student2.age); // Provides the age of the student2
As you can see that when we create separate objects and assign their properties, the code there will be a lot of repetition of the code, and as a programmer, we don't want that. Therefore we use the constructor function
Implementing the same code done above using the constructor function.
function Student(name, age, classNo){
this.name = name;
this.age = age;
this.classNo = classNo;
this.readBook = function(){
console.log("Reading book");
}
}
By convention, the naming of the constructor function uses the PascalCase unlike the naming of the variable or a normal function that uses camelCase.
The argument of the function contains the set of all properties that are used to describe the object. Inside the constructor function, we can also define a method.
'This' keyword in the function - It refers to the object
To create the object and access the properties and methods of the Constructor function, use the following code that implements the properties defined by the constructor function above.
var student1 = new Student("John", 15, 9);
var student2 = new Student("Rahul", 16, 20);
console.log(student1.name); // Provides the name of the student1
student1.readBook(); // Invokes the readBook method
console.log(student2.age); // Provides the age of the student2
Subscribe to my newsletter
Read articles from Nasif Khan directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by
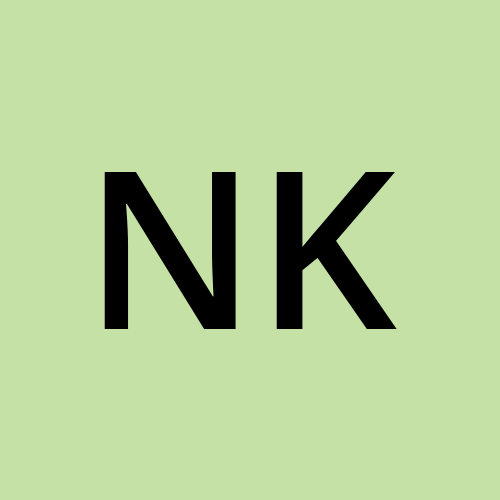