Ruby and Mpesa API : How to integrate daraja API using ruby

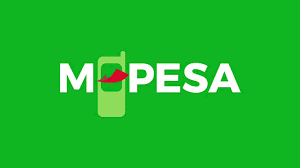
So sometimes tech languages can be the most unpredictable place to find yourself as a new techie. Recently I tried using daraja for one of my projects and was unable to get a guide on how to use it the ones that were present were outdated and couldn't apply to the new version of ruby. Safaricom is a huge enterprise they also do not have the time to guide you through the whole process luckily I am, I faced this challenge and found a solution for it.
First the setup
Set up the file and make imports for dependencies that are required like excon.
That dependency will be necessary as we proceed in the tutorial
gem 'excon', '~> 0.97.1'
Setup for the base64 encoding for the application authorization from the Safaricom sandbox to allow you to use the API
require 'base64'
require 'httparty'
require "excon"
consumer_secret = '[your_consumer_secret]'
consumer_key = '[your_consumer_key]'
def base64Encoded key,secret
data = Base64.encode64(key + ':' + secret).gsub(/\n/, '')
return data
end
token = "Basic "+ base64Encoded(consumer_key,consumer_secret)
Once the base64Encode is set the application has to send a request to confirm that the credentials are correct to let's handle that
url = 'https://sandbox.safaricom.co.ke/oauth/v1/generate?grant_type=client_credentials'
headers = {
Authorization: token
}
response = HTTParty.get(url, headers: headers)
puts response.code
accessToken = JSON.parse(response.body)["access_token"]
Every Mpesa transaction, happens in real-time for the merchant to validate that you made the payment at that particular time of purchase. For that case let's create a function that will generate the time as the transaction occurs.
def createTimestamp
year = Time.now
date = year.to_s.split(' ')[0].split('-').join('')
date_two = year.to_s.split(' ')[1].split(':').join('')
return "#{date}#{date_two}"
end
time = createTimestamp
Now that we have all that let's bring up the last part of the JSON. The Daraja API has a set of objects that are required and they must all be included. Generate the response that is parsed and you're all done.
password = Base64.strict_encode64("174379" + "[your_passKey_mpesa_express]" + time)
puts password
body = {
"BusinessShortCode"=> 174379,
"Password"=> password,
"Timestamp"=> time,
"TransactionType"=> "CustomerPayBillOnline",
"Amount"=> 1,
"PartyA"=> 254704999704,
"PartyB"=> 174379,
"PhoneNumber": 254704999704,
"CallBackURL"=> "https://mydomain.com/path",
"AccountReference"=> "BusinessName",
"TransactionDesc"=> "Payment of X"
}
headers = {
"Authorization"=> "Bearer " + accessToken,
"Content-Type" => "application/json"
}
response=Excon.post("https://sandbox.safaricom.co.ke/mpesa/stkpush/v1/processrequest",:body=>JSON.generate(body),headers:{'Content-Type': 'application/json','Authorization': "Bearer #{accessToken}"})
data=JSON.parse(response.body)
puts data
Hope that you found this article helpful. You find the full code snippet on my GitHub Till the next.
Subscribe to my newsletter
Read articles from George Onyango directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by
