Stateless Components in React: What Are They and Why Use Them?
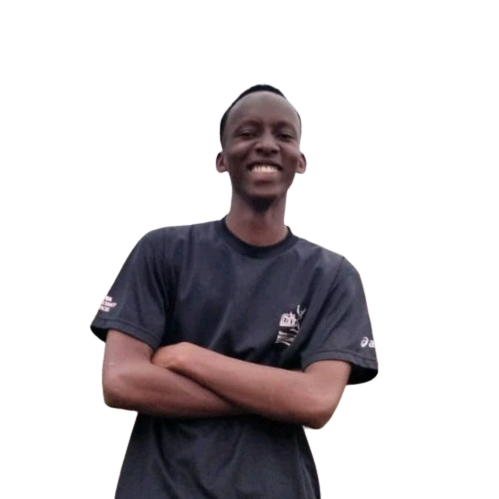
React is a popular JavaScript library for building user interfaces. It is widely used in web development and offers a lot of advantages such as component reusability, performance optimizations, and an easy-to-use programming model. One of the key concepts in React is the use of stateless components.
Stateless components are getting their philosophy from functional programming. The key idea behind functional programming is that a function always returns the same thing exactly on what is given to it. This means that a function does not have any side effects, and its output depends solely on its input. For example, a simple stateless function that calculates the sum of two numbers always returns the same result for the same input.
const statelessSum = (a, b) => a + b;
Stateful functions can have side effects and return different outputs even when called with the same input. For example, a function that increments a variable on each call has a side effect and returns different results each time it is called.
let a = 0;
const statefulSum = () => a++;
In React, a stateless component is a component that does not have any internal state management. It is a pure function of its props and renders the same output for the same input. Stateless components are also called functional components because they are simple JavaScript functions that receive props as input and return rendered JSX code.
Here is an example of a simple stateless functional component in React:
const Greeting = (props) => {
return <h1>Hello, {props.name}!</h1>;
};
This component simply takes a name
prop and renders a greeting message using it. Since it does not have any internal state management, it is a pure function of its props and will always render the same output for the same input.
There are several advantages to using stateless functional components in React. First, they are easy to write and understand. Since they are simple JavaScript functions, they are easy to reason about and test. Second, they are more performant than stateful components because they do not require a backing instance to manage the state. This means that React has more room for optimizations.
In conclusion, stateless functional components are a key concept in React that offers several advantages in terms of simplicity and performance. They are easy to write, understand, and test, and they do not have any side effects or internal state management. Therefore, it is advised to use stateless components more often, since they are side-effect free and will create the same behavior always.
Subscribe to my newsletter
Read articles from Titus Kiplagat directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by
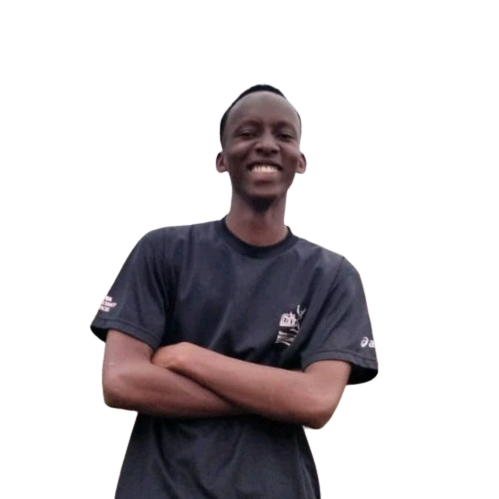
Titus Kiplagat
Titus Kiplagat
I happily spend late nights trying to build anything I think of, training AI models, or building SaaS tools that actually make people’s lives easier. With 5+ years in the game, I move across Python, JavaScript, LLMs, and backend systems like second nature — and I love blending deep tech with real-world impact. Always curious, always hands-on, always curious for the next breakthrough.