The Ultimate Guide to Using useState in React: React Hooks useState Explained
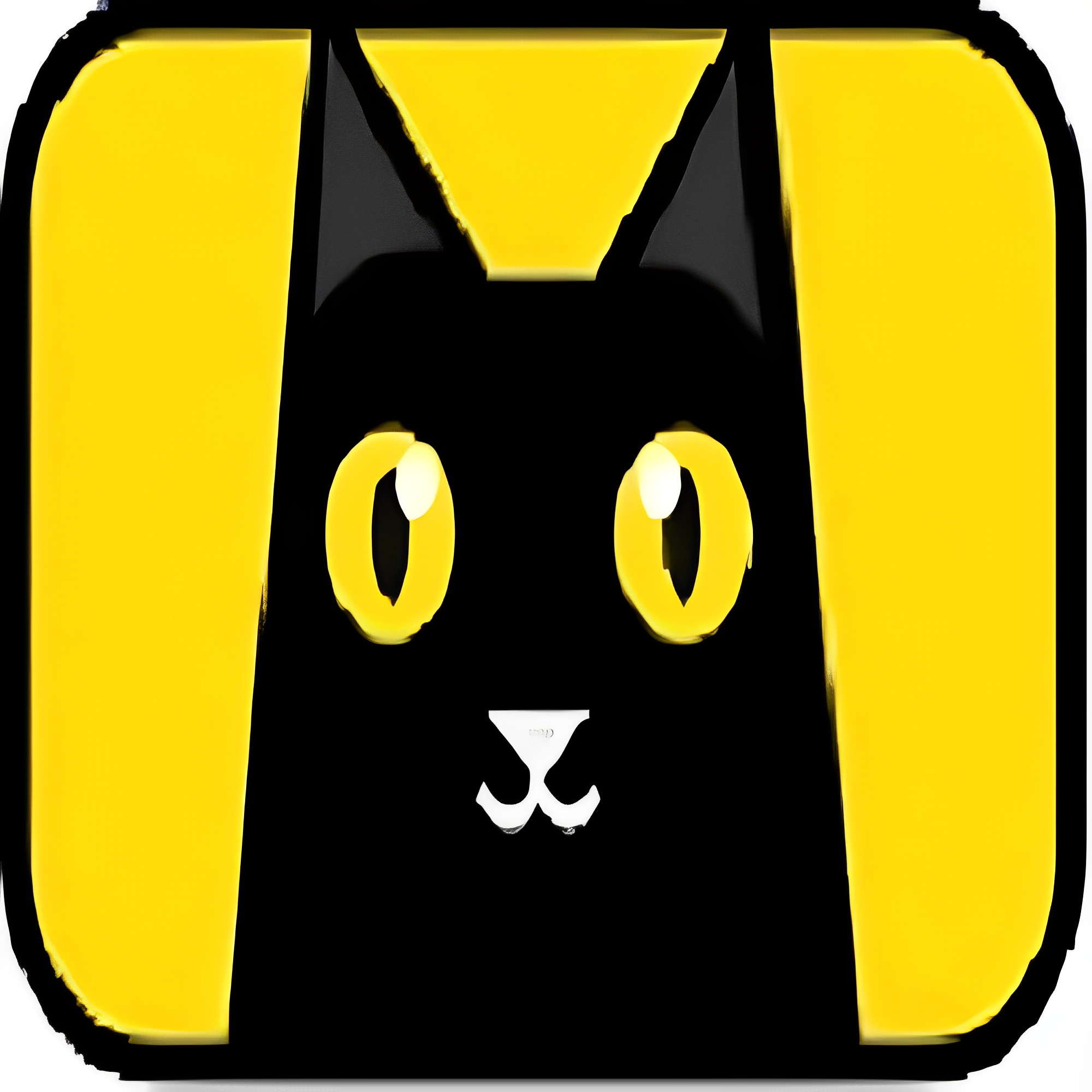
Managing state is a crucial part of building a React application. In the past, the only way to use state in a React component was by using a class component. However, with the introduction of React Hooks, it's now possible to use state in functional components as well. One of the most commonly used Hooks for managing state is the useState Hook. In this article, we'll dive deep into how to use useState in React.
What is useState in React?
useState is a Hook provided by React that allows you to add state to a functional component. The Hook takes an initial state value and returns an array containing the current state and a function to update the state. You can then use the state and updater function in your component to manage state changes. In this section, we'll explore the useState Hook in more detail.
Using the useState Hook in React
To use the useState Hook, you first need to import it from the 'react' library. You can do this by adding the following line at the top of your component file:
import React, { useState } from 'react';
Declaring State with useState
Next, you can declare state using the useState Hook. The following code declares a state variable called "count" with an initial value of 0:
const [count, setCount] = useState(0);
The first element in the array, "count," represents the current state value. The second element, "setCount," is a function that can be used to update the state. In this section, we'll explore how to declare state with useState in more detail.
Updating State with useState
To update the state, you can call the updater function returned by the useState Hook. The following code updates the count state value by incrementing it by 1:
setCount(count + 1);
You can also pass a function to the updater function to update the state based on the previous state value. The following code updates the count state value by doubling it:
setCount(prevCount => prevCount * 2);
In this section, we'll explore how to update state with useState in more detail.
Using Multiple State Values with useState
You can declare and use multiple state values in a single component by calling the useState Hook multiple times. The following code declares two state variables, "name" and "age":
const [name, setName] = useState('');
const [age, setAge] = useState(0);
In this section, we'll explore how to use multiple state values with useState in more detail.
Using Objects as State with useState
You can also use objects as state values with useState. The following code declares a state variable called "user" with an initial value of an empty object:
const [user, setUser] = useState({});
To update the state, you can use the updater function and the spread operator to merge the new state value with the previous state. The following code updates the "user" state value by merging a new object with the previous state:
setUser(prevUser => ({ ...prevUser, name: 'John', age: 30 }));
In this section, we'll explore how to use objects as state with useState in more detail.
Tips for Using useState Effectively
While useState can be a powerful tool for managing state in your React components, it's important to use it effectively. Here are a few tips to keep in mind:
1. Use Descriptive Variable Names
When initializing your state variables, use descriptive names that accurately reflect the purpose of the variable. For example, if you're tracking the user's name in a form, use a variable name like userName instead of something generic like value.
2. Avoid Complex Objects as Default Values
While you can use objects as default values for your state variables, it's generally better to avoid using complex objects with nested properties. This can lead to performance issues and make your code more difficult to debug. Instead, try to keep your default values simple.
3. Use Functional Updates for Complex State Changes
If you need to update your state based on its previous value, use a functional update instead of a standard update. This can help prevent race conditions and ensure that your state updates are always applied correctly. Here's an example:
setCount(prevCount => prevCount + 1);
In this example, we're using a functional update to increment the value of count. By using the prevCount argument, we're ensuring that our update is applied correctly even if other state changes are happening concurrently.
4. Avoid Changing State Directly
Finally, it's important to avoid changing your state variables directly. Instead, always use the setCount function (or whatever function corresponds to your state variable) to update your state. Directly changing your state variables can cause unexpected behavior and make your code more difficult to debug.
Conclusion
The useState Hook is a powerful tool for managing state in React functional components. By using the Hook, you can declare and update state values without needing to use class components. By following some best practices, such as using descriptive variable names, avoiding complex objects as default values, using functional updates for complex state changes, and avoiding changing state directly, you can use useState effectively in your React components
For more information check out this React Usestate blog from CopyCat.
Subscribe to my newsletter
Read articles from Copycat Figma directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by
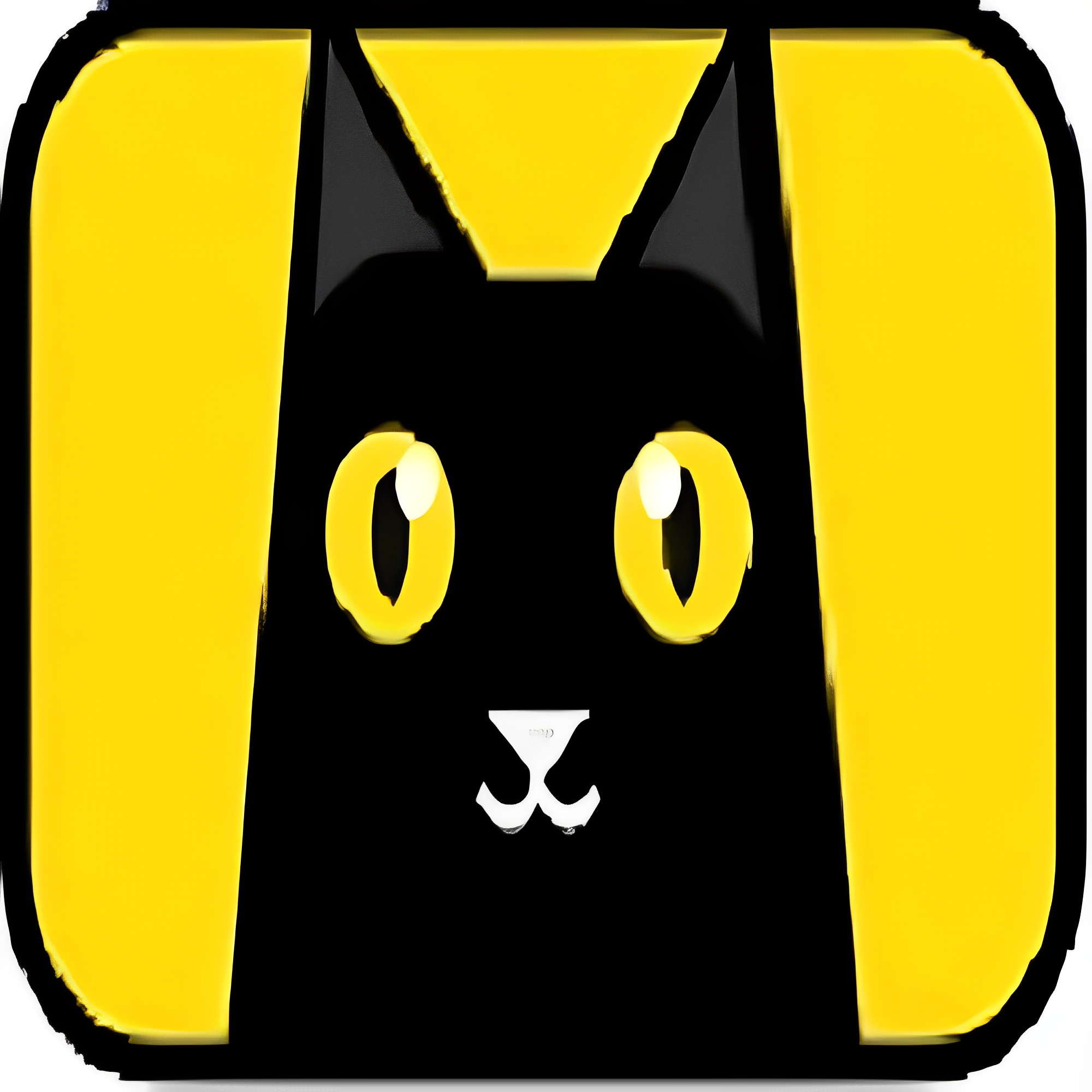
Copycat Figma
Copycat Figma
CopyCat is the Best Plugin that help in to Create beautiful websites and get more visitors to your brand with little to no coding. CopyCat can assist you! You can quickly and easily build beautiful websites with code using our user-friendly platform without knowing any coding. It's as simple as choosing your Figma design, adding your content, and clicking publish.