JavaScript Objects For Beginners.

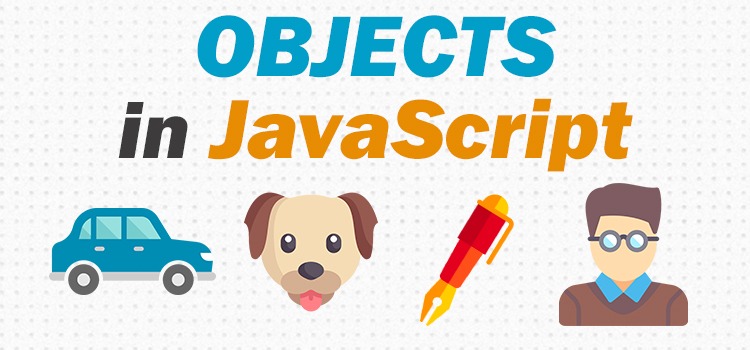
We are all familiar with the saying “JavaScript is hard to learn”. I have seen so many posts saying this and even TikTok videos, all on how hard the programming language is. Before we begin, I would like to share a trick I use in learning javascript. Whenever I am to study JavaScript I always murmur to myself “JavaScript is easy”. This makes me condition my mind and helps me believe I can understand whatever challenge is given to me regardless of how difficult it is. Try it out and let me know if it makes things easier.
What Are JavaScript Objects?
Now back to why we are here, JavaScript Objects. JavaScript object is an entity that holds data which could be strings, boolean, numbers and/or arrays. Simply put, they are a key value of storage. We will be using the block of code below to explain what these JavaScript objects are, how to use them and also how to manipulate them. Below is an example of JavaScript objects that hold pieces of data:
const cat = { name: "whiskers", legs: 4, 5: "five", enemies: ["water", "dogs"] };
From the block of code above, “const” acts as a variable and is used to define the variable in which the object is stored. The strings and numbers on the left represent the properties while the strings and numbers on the right represent the values of the already given properties.
Note: Tips you should always follow while writing JavaScript Objects:
Always use quotations while writing strings.
Do not use quotations for numbers.
Always add a comma(,) after every value of a property except the very last property of the object.
Accessing JavaScript Objects
There are two ways by which JavaScript objects can be accessed. They include
Dot notation.
Bracket notation.
The major difference between these two notations is that one uses dot “.” to access properties in an object while the other uses solid brackets “[]” to access said properties. Another distinguishing factor between these two notations is that dot notation is used when you know the name of the property you are trying to access.
These can be seen below
var catName =
cat.name
; console.log(catName);
From the block of code above, the console would return the string “whiskers”. Now here is why, as seen above the dot notation was saved in a variable “catName” . The dot notation “cat.name” simply means, access/ draw out the value of the property “name” from the object “cat”. Hence the string “whiskers” was returned.
A similar situation can be seen while using the bracket notation as shown below
var catName = cat[name]; console.log(catName);
The above block of code also returns the string “whiskers”. Now you see the same results. I am curious. Of these two notions mentioned above, which do you prefer?
If you understand to this extent, good job! You have done a nice percentage of the work. Next, we would be looking at ways we could manipulate these objects.
Updating JavaScript Object Properties
The values of properties in javascript objects can be easily updated and stick around with me while I show you how. We would also be using the JavaScript object mentioned above as a case study.
const cat = { name: "whiskers", legs: 4, 5: "five", enemies: ["water", "dogs"] };
In this article, I would be using both the dot and bracket notations. However, one is enough whilst you code. Now let’s try changing the name in our JavaScript object, shall we?
cat.name
= "Luke"; console.log(
cat.name
);
The above would return the string “Luke” which is the new value of our “name” property in our “cat” object. Easy right? The same thing can be done using the bracket notation and it would be represented as
cat["name"] = "Luke"; console.log(cat[“name”]);
The above block of code would also return the string “Luke” as the new value of the “name” property. As a challenge, you could try creating your own JavaScript objects and try updating their properties.
Adding New Properties To JavaScript Objects
Similar to updating properties of JavaScript objects, we can also add new properties to these JavaScript objects. The only difference is that here you would be defining both the new property and values using either the dot or bracket notations. Now it’s time for some examples:
const cat = { name: "whiskers", legs: 4, 5: "five", enemies: ["water", "dogs"] };
cat.hair
= “brown”;
console.log(cat);
The above block of code shows a new property attached to the “cat” object. The new property’ value was also indicated as “brown”. Hence, returning the JavaScript object cat gives
const cat = { name: "whiskers", legs: 4, 5: "five", enemies: ["water", "dogs"],
hair: “brown” };
`The above shows that the new property has successfully been added to our JavaScript object. Using the bracket notation, we could also add a new property using:
const cat = { name: "whiskers", legs: 4, 5: "five", enemies: ["water", "dogs"] };
cat[“hair”] = “brown”;
This would also produce the same results as the dot notation above.
Deleting Properties From JavaScript Objects
Just as new properties can be added to JavaScript objects, properties can also be deleted using the “delete” keyword. Now let’s see some examples below:
const cat = { name: "whiskers", legs: 4, 5: "five", enemies: ["water", "dogs"] };
delete.enemies;
This deletes the property “enemies” and returns the object:
const cat = { name: "whiskers", legs: 4, 5: "five", };
You could also delete properties using the bracket notations as mentioned earlier in this article.
Testing JavaScript Objects For Properties
This can be done to test to see if a given property exists in an object. In this scenario, we use the keyword “.hasOwnProperty()” with the property name in parentheses. That is the keyword scans through a given JavaScript object to check if it contains a particular property. This is illustrated below:
const cat = { name: "whiskers", legs: 4, 5: "five", enemies: ["water", "dogs"] };
cat.hasOwnProperty(legs);
Returns the number 4.
cat.hasOwnProperty(enemies);
Returns the array:
["water", "dogs"];
Conclusion
JavaScript objects are simply entities that contain pieces of data that can be edited or replaced. I hope you enjoyed this read and I hope you've understood the basics of JavaScript objects. Have you got questions? Feel free to ask in the comments.
Subscribe to my newsletter
Read articles from Excel Aigbosuria directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by
