React Table Sorting: How to Make Your Data Display More Dynamic
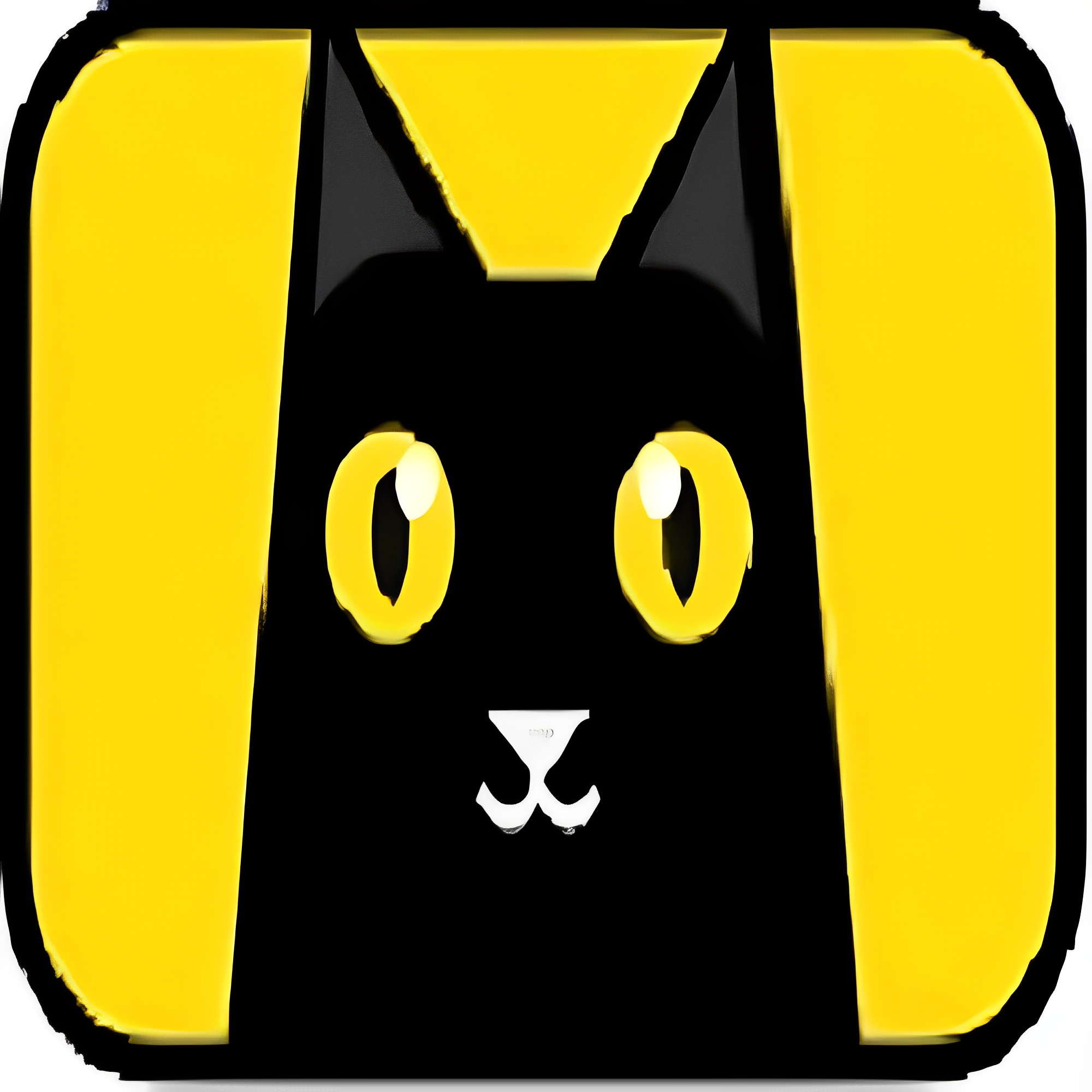
React Table is a powerful library for building tables in React applications, and one of its most useful features is sorting. Sorting allows users to order the data in a table by a particular column, making it easier to find the information they need.
In this article, we'll explore how to implement sorting in React Table and make your data display more dynamic. We'll go over the basics of sorting in React Table, including how to add sorting to your table and customize the sorting behavior.
Adding Sorting to React Table
To add sorting to a React Table, we need to specify which columns should be sortable. We can do this by passing in a columns array to our React Table component and setting the sortable property to true for the columns we want to make sortable.
For example, let's create a simple table of users with their names, ages, and countries, and make the name and age columns sortable:
javascript
import ReactTable from 'react-table';
import 'react-table/react-table.css';
const data = [
{ name: 'John', age: 30, country: 'USA' },
{ name: 'Jane', age: 25, country: 'Canada' },
{ name: 'Bob', age: 40, country: 'Mexico' },
{ name: 'Alice', age: 35, country: 'France' },
];
const columns = [
{
Header: 'Name',
accessor: 'name',
sortable: true,
},
{
Header: 'Age',
accessor: 'age',
sortable: true,
},
{
Header: 'Country',
accessor: 'country',
},
];
function SortingTable() {
return (
<ReactTable data={data} columns={columns} />
);
}
With this code, we've added sorting to our name and age columns. The column headers will now display a clickable arrow icon, indicating that they are sortable.
Customizing Sorting in React Table
React Table provides several options for customizing the sorting behavior of a table. We can control the initial sort order, change the sort icon, and even implement custom sorting logic.
Initial Sort Order
By default, React Table sorts the data in ascending order when the user clicks on a sortable column. We can specify a different initial sort order by setting the defaultSorted prop on the React Table component.
For example, let's sort our table by age in descending order:
javascript
function SortingTable() {
const defaultSort = [{ id: 'age', desc: true }];
return (
<ReactTable data={data} columns={columns} defaultSorted={defaultSort} />
);
}
With this code, our table will be initially sorted by age in descending order.
Custom Sort Icon
React Table allows us to customize the sort icon that appears in the column headers. We can specify our own custom icons for ascending and descending order by passing in a customSortIcon prop to our React Table component.
For example, let's use FontAwesome icons for our sort icons:
javascript
import { FontAwesomeIcon } from '@fortawesome/react-fontawesome';
import { faSort, faSortUp, faSortDown } from '@fortawesome/free-solid-svg-icons';
function SortingTable() {
const customSortIcon = {
sort: <FontAwesomeIcon icon={faSort} />,
asc: <FontAwesomeIcon icon={faSortUp} />,
desc: <FontAwesomeIcon icon={faSortDown} />,
};
return (
<ReactTable data={data} columns={columns} customSortIcon={customSortIcon} />
);
}
With this code, our sort icons will use the FontAwesome icons for sort, ascending order, and descending order.
Custom Sorting Logic
React Table also allows for custom sorting logic to be implemented. This can be useful in scenarios where the default sorting behavior doesn't quite fit your needs. To implement custom sorting logic, you can define a custom sort function and pass it to the useSortBy hook.
The custom sort function should take two arguments, representing the two values to be compared during sorting, and return -1, 0, or 1 to indicate their relative order. For example, if you wanted to sort a list of numbers by their absolute values, you could define a custom sort function like this:
function sortByAbsoluteValue(a, b) {
const absA = Math.abs(a);
const absB = Math.abs(b);
if (absA < absB) {
return -1;
}
if (absA > absB) {
return 1;
}
return 0;
}
You can then pass this function to the useSortBy hook as follows:
const {
headers,
rows,
prepareRow,
setSortBy,
} = useTable(
{
columns,
data,
// Pass the custom sort function to useSortBy
initialState: { sortBy: [{ id: 'value', desc: false }] },
sortTypes: {
absoluteValue: sortByAbsoluteValue,
},
},
useSortBy,
);
In this example, we're passing the custom sort function to useSortBy via the sortTypes option, and specifying that the initial sort should be by the value column in ascending order. Now, when the user clicks the column header to sort the table, our custom sort function will be used instead of the default behavior.
Conclusion
Sorting is a powerful feature in React Table that allows users to quickly and easily find the information they need in a table. By implementing sorting in our React Table components, we can make our data display more dynamic and user-friendly.
In this article, we've covered the basics of adding sorting to a React Table, including how to make columns sortable and how to customize the sorting behavior. We've also explored some advanced sorting features, such as specifying an initial sort order, customizing the sort icon, and implementing custom sorting logic.
With React Table's flexible and customizable sorting options, we can create tables that meet the specific needs of our users and provide a seamless and intuitive experience. By incorporating sorting into our React Table components, we can take our data display to the next level and provide our users with a powerful tool for exploring and understanding our data.
For more information check out this React Data Table blog from CopyCat.
Subscribe to my newsletter
Read articles from Copycat Figma directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by
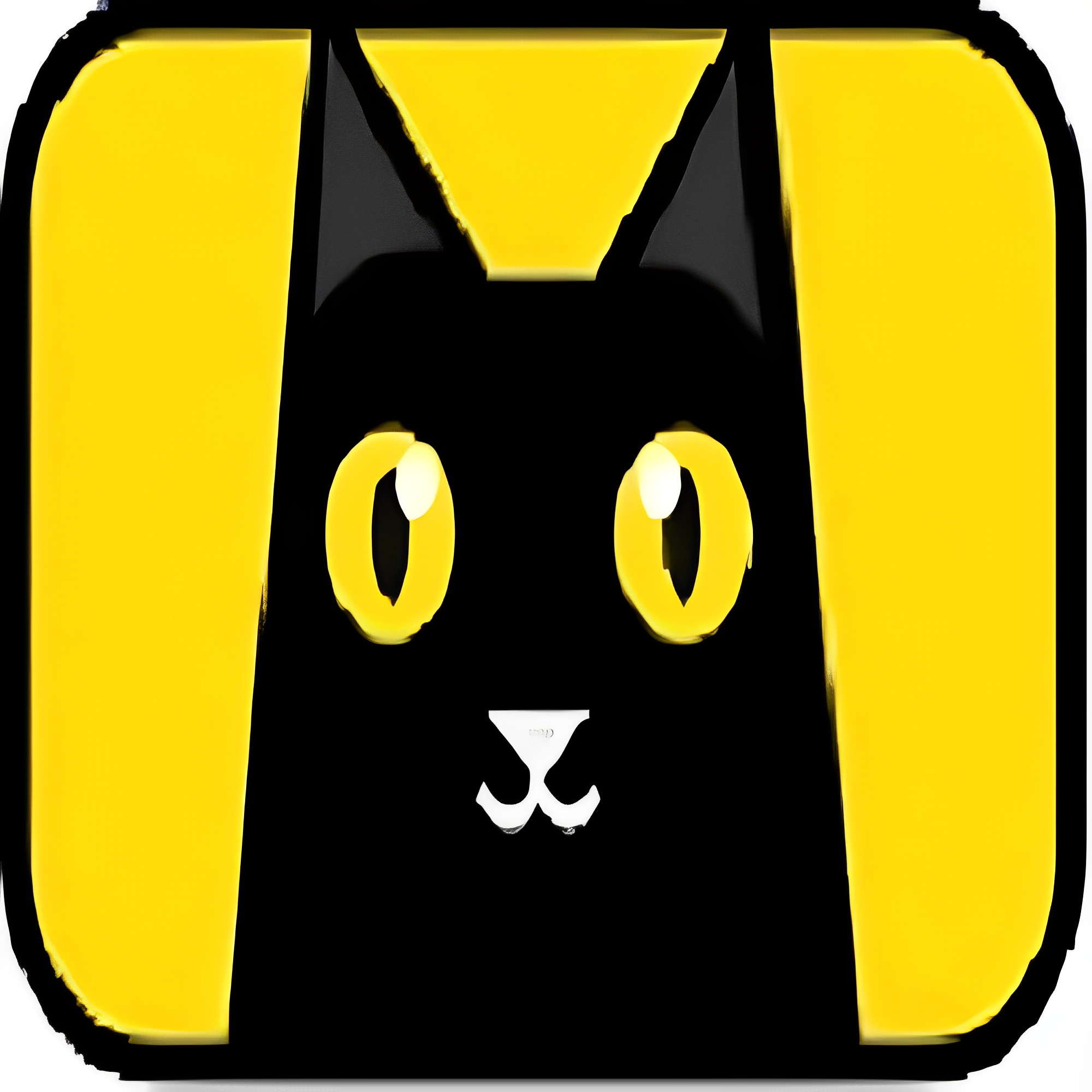
Copycat Figma
Copycat Figma
CopyCat is the Best Plugin that help in to Create beautiful websites and get more visitors to your brand with little to no coding. CopyCat can assist you! You can quickly and easily build beautiful websites with code using our user-friendly platform without knowing any coding. It's as simple as choosing your Figma design, adding your content, and clicking publish.