Working with Maps in JavaScript: Storing Key-Value Pairs with Flexibility and Order
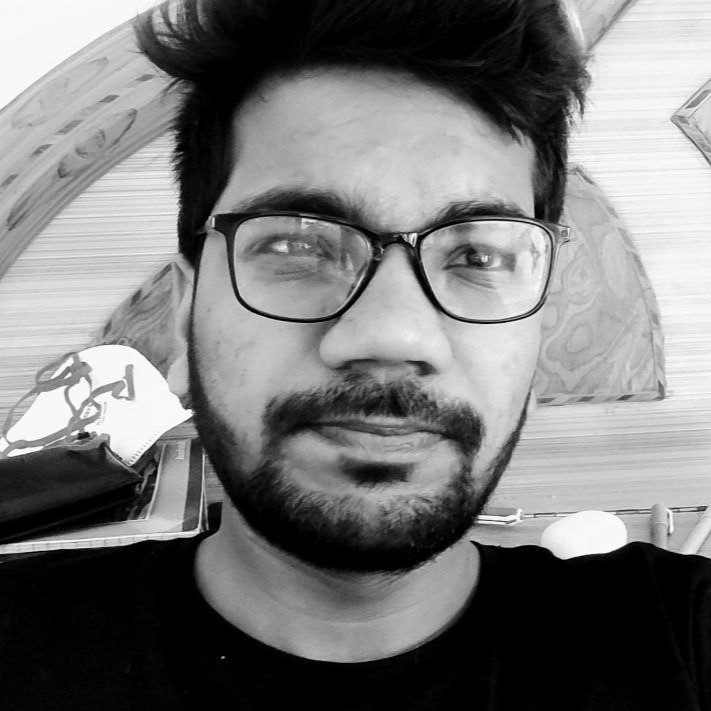
Table of contents
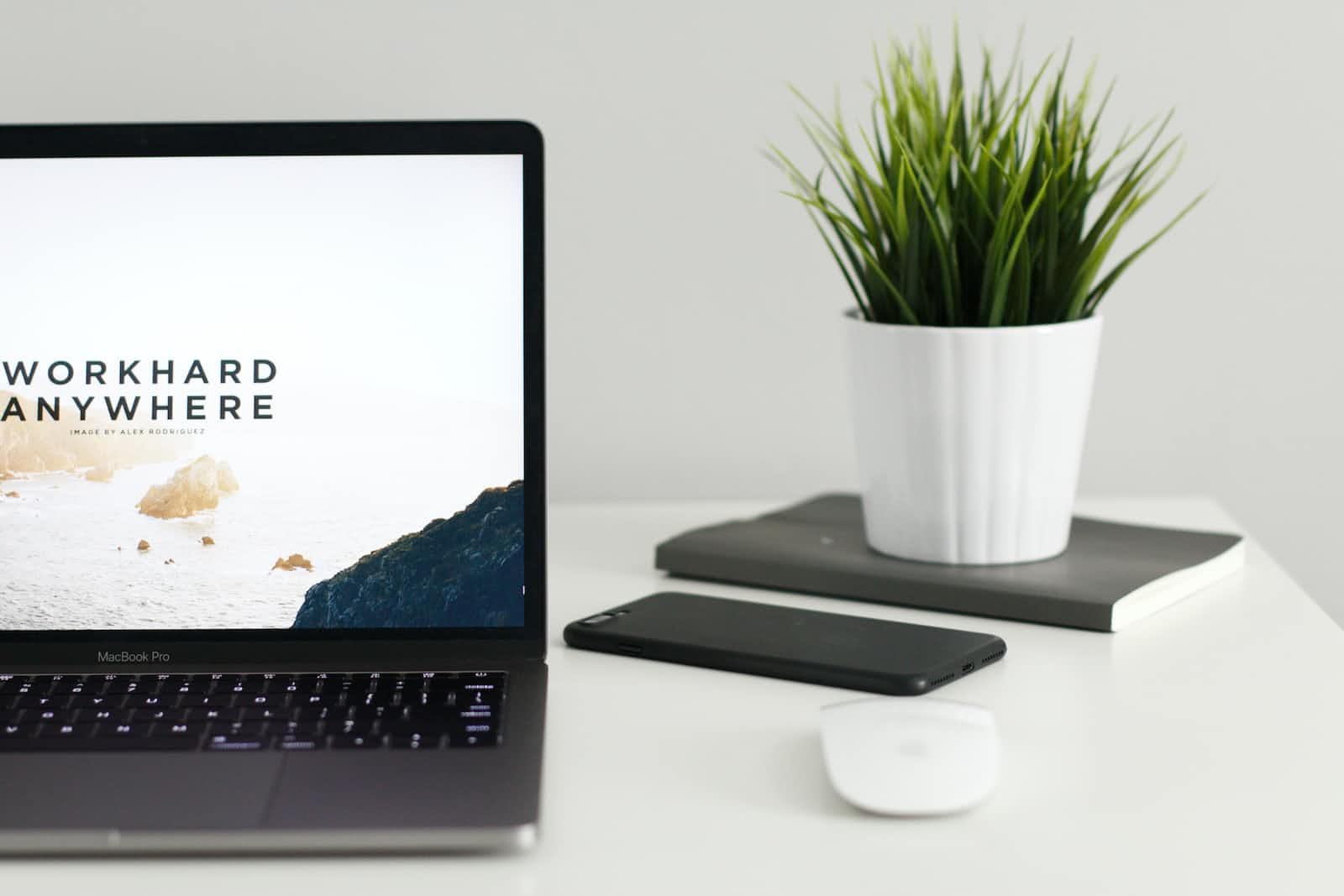
In JavaScript, a map is a built-in data structure that allows you to store key-value pairs. A map is similar to an object, but with some important differences:
A map can use any value as a key, including objects, whereas objects can only use strings or symbols as keys.
A map maintains the order of its elements, whereas object properties have no guaranteed order.
To create a map, you can use the Map constructor or the Map literal syntax:
// Using the Map constructor
const myMap = new Map();
// Using the Map literal syntax
const myMap = new Map([
[key1, value1],
[key2, value2],
[key3, value3],
]);
To add or update a value in a map, you can use the set()
method:
myMap.set(key, value);
To retrieve a value from a map, you can use the get()
method:
const value = myMap.get(key);
To check if a key exists in a map, you can use the has()
method:
const exists = myMap.has(key);
To remove a key-value pair from a map, you can use the delete()
method:
myMap.delete(key);
To iterate over the keys, values, or entries (key-value pairs) in a map, you can use the keys()
, values()
, and entries()
methods, respectively:
// Iterate over keys
for (const key of myMap.keys()) {
console.log(key);
}
// Iterate over values
for (const value of myMap.values()) {
console.log(value);
}
// Iterate over entries
for (const [key, value] of myMap.entries()) {
console.log(key, value);
}
Maps are a useful data structure in many scenarios, such as caching, memoization, and maintaining state.
Subscribe to my newsletter
Read articles from Himanshu Singla directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by
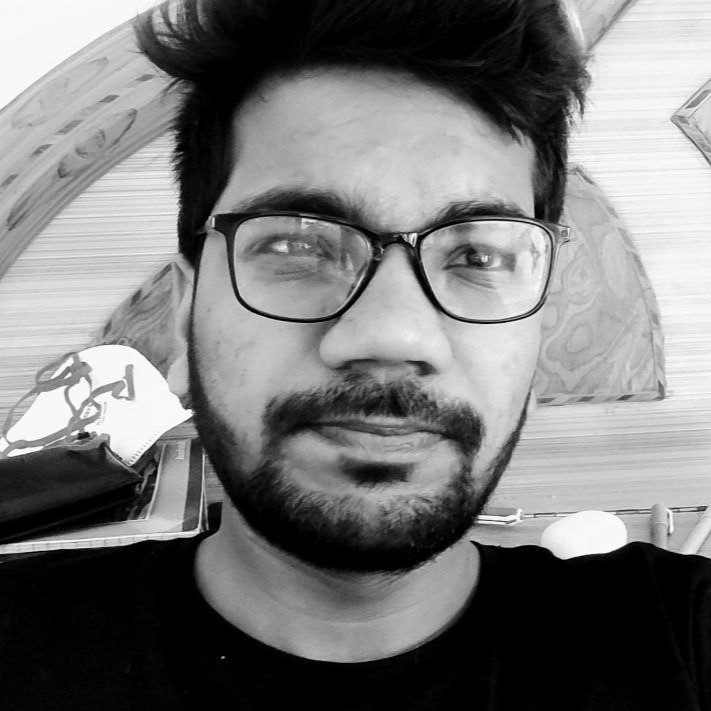
Himanshu Singla
Himanshu Singla
As a MERN stack developer, he holds the role of a Project Engineer at Crio. His insatiable curiosity drives him to actively seek out opportunities to assist and engage with individuals, as well as to continually explore novel concepts and technologies.