Delete Without Head Pointer in Linked List

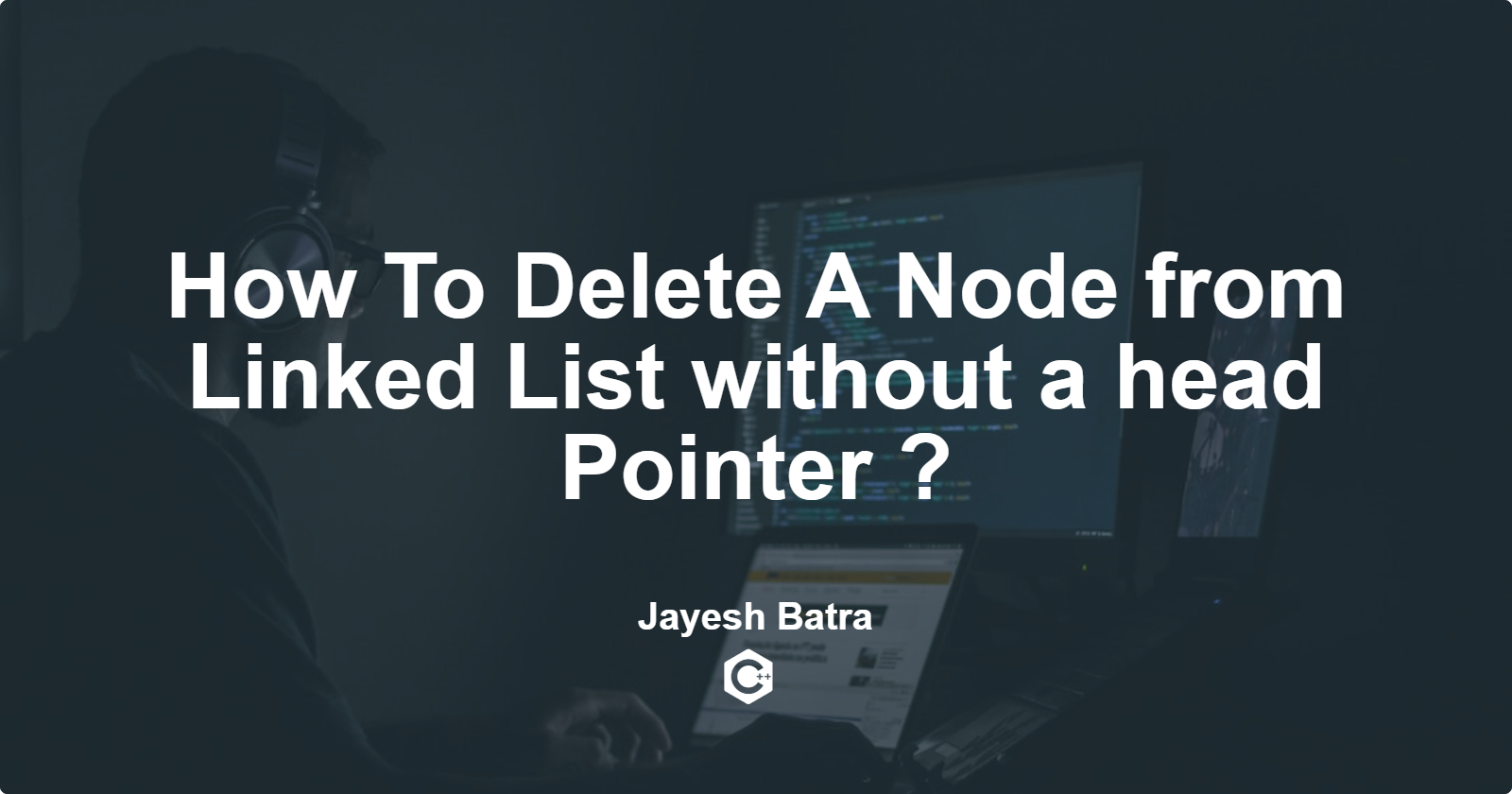
So hey, in this article we are going to learn to Delete Without Head Pointer in a Linked List
Question Explanation
If you could not understand the Question. Refer this :
This question is asking you to delete a node from a linked list without being given a reference to the head node. A linked list is a data structure that consists of a sequence of nodes, each containing a value and a reference to the next node in the sequence. To delete a node from the linked list, you typically need to update the reference of the previous node to point to the next node.
However, in this case, you are not given a reference to the head node, which makes the task more challenging. The question states that you are given a pointer or reference to the node that is to be deleted, and it is guaranteed that this node is not the tail node in the linked list. This means that there is at least one node after the node to be deleted, so you can update the reference of the current node to point to the next node and effectively "skip" over the node to be deleted.
To do this, you can simply copy the value and reference of the next node into the current node, effectively overwriting the current node with the next node. Then, you can delete the next node instead. This effectively removes the current node from the linked list without needing to update any other references.
In summary, this question is asking you to delete a node from a linked list without a reference to the head node. To do this, you can copy the value and reference of the next node into the current node, effectively overwriting it, and then delete the next node instead.
Try the question once more after understanding the Question and if still not able to code then don't worry, here's the code and its detailed explanation.
Code
void deleteNode(Node *del)
{
Node * prev = del;
Node * curr = del->next;
Node * next = del->next->next;
int temp = prev->data;
prev->data = curr->data;
curr->data = temp;
prev->next=next;
curr->next=NULL;
delete curr;
}
Code Explanation
This code defines a function deleteNode that takes a pointer to a Node as its argument. The function is meant to remove the given node from a linked list, by swapping its data with the data of the next node, and then delete the next node.
The function first initializes three pointers: prev, curr, and next. prev is set to the input node, curr is set to the node immediately after the input node, and next is set to the node after curr.
Then, the function swaps the data of prev and curr nodes using a temporary variable temp.
Next, prev is made to point to next, effectively removing curr from the linked list. curr's next pointer is set to NULL, indicating that it is no longer part of the list.
Finally, curr is deleted using the delete operator, freeing up its memory.
Note that this code assumes that the input node is not the last node in the linked list, i.e., that there is a node after it. If the input node is the last node, this code may result in a segmentation fault, as it tries to access memory beyond the end of the linked list.
Subscribe to my newsletter
Read articles from Jayesh Batra directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by

Jayesh Batra
Jayesh Batra
I am a passionate and motivated individual with a strong desire to excel in the field of software development. With my current focus on MERN stack, DSA, and open source, I am well-positioned to make valuable contributions to any project or team I work with.