code inputs
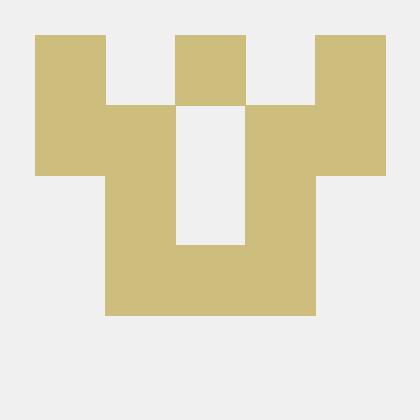
building apps and inputting codes can be done in a variety of ways depending on the programming language or framework being used. In the context of the provided document related to React, building an app can be accomplished by writing reusable components that can be combined together to create complex user interfaces. Inputting codes in React involves writing JSX, a syntax extension to JavaScript that allows developers to define the structure and behavior of their components in a more declarative way. Here is an example of a simple React component that renders a button:
import React from 'react';
function Button({ text, onClick }) { return {text}; }
In this example, the Button
component takes two props, text
and onClick
, and renders a button element with the specified text
and onClick
function handler. To use this component in a React app, you would import it and use it in another component like so:
import React from 'react'; import Button from './Button';
function App() { return (
Hello, World!
<Button text="Click me" onClick={() => console.log('Button clicked')} />
export default App;
In this example, the App
component renders a heading and a Button
component with the specified props. When the button is clicked, the onClick
function handler is called and will log a message to the console.
Based on the provided document, you can render a React app in three steps: Trigger, Render, and Commit.
Trigger: This step involves an event or a change that triggers a re-render, such as a user interaction or a change in state or props.
Render: During this step, React will call each component's function and create a virtual representation of the updated UI in memory. Components higher up in the tree are rendered first.
Commit: After rendering the components, React will modify the DOM as necessary to match the updated virtual representation of the UI, using the
appendChild()
DOM API for the initial render and minimal necessary operations for re-renders. React only changes the DOM nodes that have differences between renders.
Here is an example of a simple React app that can be rendered following these three steps:
import React, { useState } from 'react';
function App() { const [count, setCount] = useState(0);
function handleClick() { setCount(count + 1); }
return (
Click Count: {count}
Click me!
export default App;
In this example, the App
component has a state variable count
that starts at 0 and increments by 1 every time the button is clicked. The handleClick
function updates the count state when the button is clicked. When this app is rendered, React will first trigger a re-render when the button is clicked, then render the App
component with the updated state and render the h1
and button
elements, and finally commit the changes to the DOM so that the updated UI is displayed on the screen.
import React, { useState } from 'react'; import { TextInput } from 'react-native';
const MyTextInput = () => { const [value, setValue] = useState('');
const onChangeText = (newText) => { setValue(newText); };
return ( ); };
Subscribe to my newsletter
Read articles from LaurenN directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by
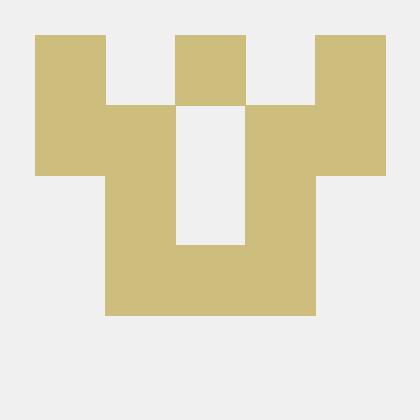