React Components Newbie info
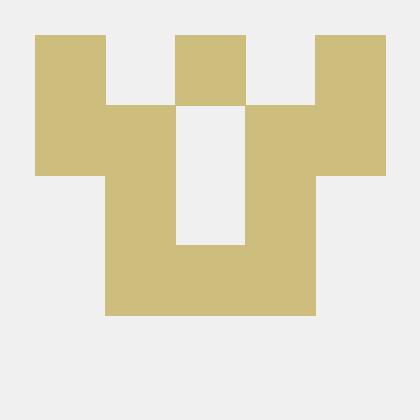
Table of contents
The mentioned documents do not have any specific code snippet related to "values created to make the app function". However, here is a common approach to create values to make a React Native app function.
Typically, in React Native apps, values such as state and props are used to store and manage data and UI-related information. State values can be created using the useState
hook provided by React, and props can be passed down to child components from parent components.
Here is a basic example of state and props in a React Native app:
import React, { useState } from 'react'; import { Text, View } from 'react-native';
const MyComponent = ({ title }) => { const [count, setCount] = useState(0);
const handleIncrement = () => { setCount(count + 1); };
return ( {title} {count} ); };
In the above example, the useState
hook is used to create the count
state value, which is initially set to 0. The setCount
function is used to update the count
value in response to user interaction.
The MyComponent
component also receives a title
prop, which is used to render a title text in the component. The Button
component is used to provide a user interface for the handleIncrement
function, which is called when the user clicks the button.
The AppStateExample
is a class component in React Native that demonstrates the usage of the AppState
API. It uses the AppState
module provided by React Native to listen for changes in the app state and render the current app state on the screen.
In its constructor, it initializes the state
object with the current app state obtained from the AppState.currentState
property.
In componentDidMount
, it adds an event listener to the AppState
API to listen for changes in the app state. Whenever there is a change in the app state, it checks if the app has come into the foreground from the inactive or background state and logs a message to the console. It then updates the state
object with the new app state.
In componentWillUnmount
, it removes the event listener added in componentDidMount
.
Finally, in render
, it renders the current app state on the screen wrapped in a View
component and a Text
component.
Here is the code snippet for the AppStateExample
component with TypeScript type annotations:
import React, { Component } from 'react'; import { AppState, StyleSheet, Text, View } from 'react-native';
type AppStateStatus = 'active' | 'background' | 'inactive';
interface AppStateExampleState { appState: AppStateStatus; }
class AppStateExample extends Component<{}, AppStateExampleState> { appStateSubscription?: ReturnType;
state: AppStateExampleState = { appState: AppState.currentState, };
componentDidMount() { this.appStateSubscription = AppState.addEventListener( 'change', (nextAppState: AppStateStatus) => { if ( this.state.appState.match(/inactive|background/) && nextAppState === 'active' ) { console.log('App has come to the foreground!'); } this.setState({ appState: nextAppState }); }, ); }
componentWillUnmount() { this.appStateSubscription?.remove(); }
render() { return ( Current state is: {this.state.appState} ); } }
const styles = StyleSheet.create({ container: { flex: 1, justifyContent: 'center', alignItems: 'center', }, });
export default AppStateExample;
Subscribe to my newsletter
Read articles from LaurenN directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by
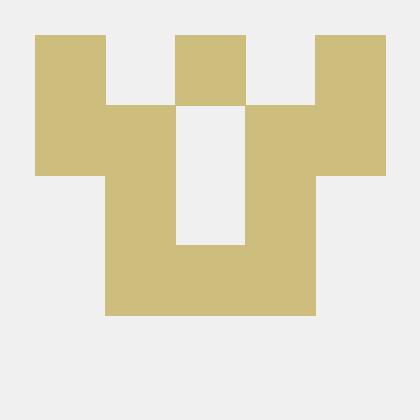