Implementing Image Picker in Flutter

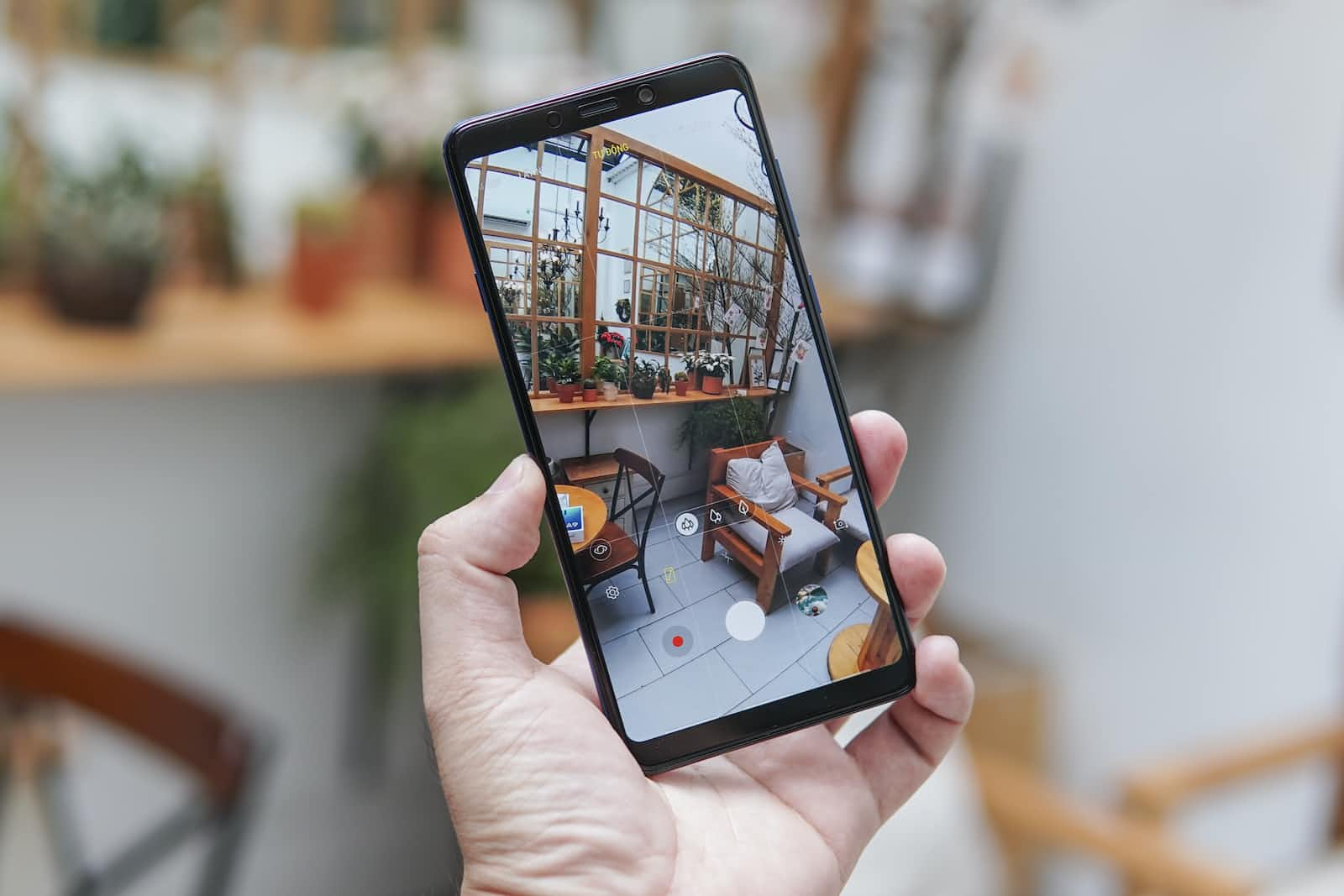
The image_picker package is a great way to add the ability to pick images from the gallery or take a photo with the camera to your Flutter app. It's easy to use and provides a lot of flexibility.
Getting Started
To use the image_picker package, you'll need to add it to your pubspec.yaml file:
dependencies:
image_picker: ^0.8.3
Then, you'll need to import it into your code:
import 'package:image_picker/image_picker.dart';
Picking an Image from the Gallery
To pick an image from the gallery, you can use the pickImage() method:
Future<XFile?> pickImage() async {
final picker = ImagePicker();
final pickedFile = await picker.pickImage(source: ImageSource.gallery);
return pickedFile;
}
The pickImage() method returns a Future<XFile?>. The Future will complete with the picked image file, if any. If no image was picked, the Future will complete with null.
Once you have the picked image file, you can use it however you like. For example, you can display it in a widget, save it to the device, or upload it to a server.
Taking a Photo with the Camera
To take a photo with the camera, you can use the takePicture() method:
Future<XFile?> takePicture() async {
final picker = ImagePicker();
final pickedFile = await picker.takePicture(source: ImageSource.camera);
return pickedFile;
}
The takePicture() method returns a Future<XFile?>. The Future will complete with the taken photo file, if any. If no photo was taken, the Future will complete with null.
Once you have the taken photo file, you can use it however you like. For example, you can display it in a widget, save it to the device, or upload it to a server.
Tips
Here are a few tips for using the image_picker package:
Make sure to ask for permission to access the camera and gallery before using the image_picker package. You can do this by calling the requestPermissions() method.
Be careful not to store sensitive data in the image files you pick or take. The image files may be accessible to other apps on the device.
Use the Image.file() widget to display an image file in your app.
Use the Image.network() widget to display an image from a URL.
Conclusion
The image_picker package is a great way to add the ability to pick images from the gallery or take a photo with the camera to your Flutter app. It's easy to use and provides a lot of flexibility.
Subscribe to my newsletter
Read articles from Sherigabia Pambo directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by

Sherigabia Pambo
Sherigabia Pambo
Human 🙋| Dagbana 🦁| Son😊| Brother 😏|Coolest Uncle Ever😎| Coffee lover❤️| Software Dev💻| Gamer🎮