Flow of code execution in JavaScript
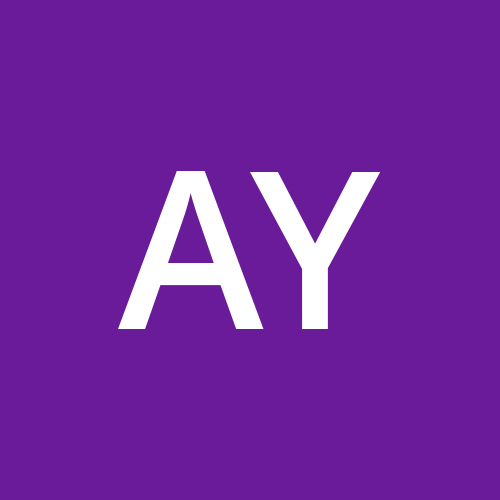
JavaScript is a single-threaded language, which means that it can only execute one piece of code at a time. Understanding the flow of code execution in JavaScript is essential for writing efficient and bug-free code. In this article, we will explore the flow of code execution in JavaScript.
Execution Context
When JavaScript code is executed, it runs inside an execution context. The execution context is a container that holds information about the currently executing code, such as variable values and function calls.
There are two types of execution contexts in JavaScript: global and local. The global execution context is created when the script is loaded and is used to store global variables and functions. Local execution contexts are created whenever a function is called and are used to store local variables and function calls.
Call Stack
The call stack is a data structure that keeps track of the currently executing functions. Whenever a function is called, a new execution context is created and added to the top of the call stack. When the function finishes executing, its execution context is removed from the call stack, and control is returned to the previous execution context.
Here's an example of how the call stack works:
function add(a, b) {
return a + b;
}
function multiply(a, b) {
return a * b;
}
function calculate(a, b) {
const sum = add(a, b);
const product = multiply(a, b);
return sum + product;
}
calculate(2, 3);
When the calculate
function is called, a new execution context is created and added to the top of the call stack. Inside the calculate
function, the add
and multiply
functions are called, and new execution contexts are created and added to the top of the call stack. When the add
and multiply
functions finish executing, their execution contexts are removed from the call stack, and control is returned to the calculate
function. When the calculate
function finishes executing, its execution context is removed from the call stack, and the script finishes executing.
Conclusion
Understanding the flow of code execution in JavaScript is crucial for writing efficient and bug-free code. By understanding how execution contexts and the call stack work, you can better understand how your code is executed and identify potential issues.
Subscribe to my newsletter
Read articles from Aakash Yadav directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by
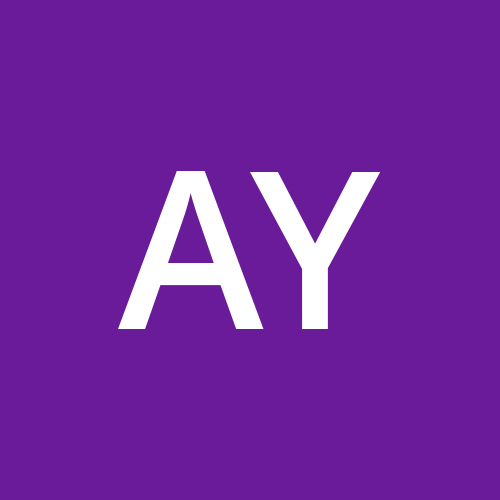