Set object in JavaScript
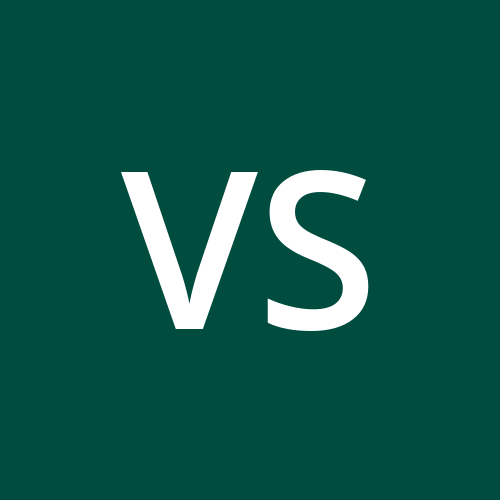
Table of contents
- What is a set in JavaScript?
- How can you create a new set?
- How can you insert a new element into your set?
- If you want to check what "1" has in your set object, How can you do it?
- How can you delete an item from your Set?
- How can you check the size of your Set?
- Can you remove all items from your set?
- Some iterable methods in the set.
- Points to remember
In this post, we will discuss the benefits of JavaScript set objects, and how to use them.
So, let's start.
What is a set in JavaScript?
JavaScript is a powerful programming language that offers variant ways to manage your data and collection of data. And one of those ways is a JavaScript object known as Set, which is used specifically for data collection.
The set object lets you store the unique value of any type. but cannot contain duplicate items, In the case you are trying to add duplicate or equal items, only the first instance will be saved to the set.
const numbers = new Set([1, 2, 3, 4, 5, 2, 3, 2, 4, "hello", "hello"])
console.log(numbers)
//Set(6) { 1, 2, 3, 4, 5, 'hello' }
How can you create a new set?
With the help of the new Set() method, we can create a new set.
For example:-
const mySet = new Set()
Now you have created a new set which is mySet.
How can you insert a new element into your set?
we can use mySet.add() method to insert a new element into our set.
Now we are continuing with our previous set.
in the following example, we are adding three elements to our set.
For example:
const mySet = new Set()
mySet.add(1)
mySet.add({ a: 1, b: 2 })
mySet.add('some text')
console.log(mySet)
//Set(3) { 1, { a: 1, b: 2 }, 'some text' }
Now we have three elements in our set first is "1" second is "{ a: 1, b: 2 }" and the third is 'some text'.
If you want to check what "1" has in your set object, How can you do it?
We have set.has() method in our Set object which helps us check existing value/item. And return a boolean value asserting whether an element is present with the given value in the set object or not.
console.log(mySet.has(1))
//true
How can you delete an item from your Set?
We can use mySet.delete() method and delete any item from existing sets.
mySet.delete('some text')
console.log(mySet)
//Set(2) { 1, { a: 1, b: 2 } }
How can you check the size of your Set?
Use mySet.size and check your set's size
console.log(mySet.size)
//2
Can you remove all items from your set?
Yes, we can use mySet.clear() and remove all the items from our set.
mySet.clear()
console.log(mySet)
//Set(0) {}
Some iterable methods in the set.
entries():- It returns an object of Set iterator that contains an array of [value, value] for each element. In array[value, value], the first value represents the key whereas the second value represents the value of the element
const mySet = new Set()
mySet.add(1)
mySet.add({ a: 1, b: 2 })
mySet.add('some text')
console.log(mySet.entries())
//[Set Entries] {
// [ 1, 1 ],[ { a: 1, b: 2 }, { a: 1, b: 2 } ],[ 'some text', 'some text' ]}
value():- It returns an object of Set iterator. This object contains the value for each element and maintains the order of insertion.
const mySet = new Set()
mySet.add(1)
mySet.add({ a: 1, b: 2 })
mySet.add('some text')
const mySetValue = mySet.values()
console.log(mySetValue)
//[Set Iterator] { 1, { a: 1, b: 2 }, 'some text' }
Points to remember
Sets are collections of unique value.
Sets can contain values of any data type and do not have keys associated with the values.
Sets are not immutable, they can be changed after creation.
A set object iterates its elements in insertion order.
There are multiple ways to iterate over sets.
In the Set, we can not store data in key-value pairs
If I made any mistake in this article. So, I am sorry, Please give me your suggestion..!!!.
Thank you🙂.
Subscribe to my newsletter
Read articles from Vinayak Singh directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by
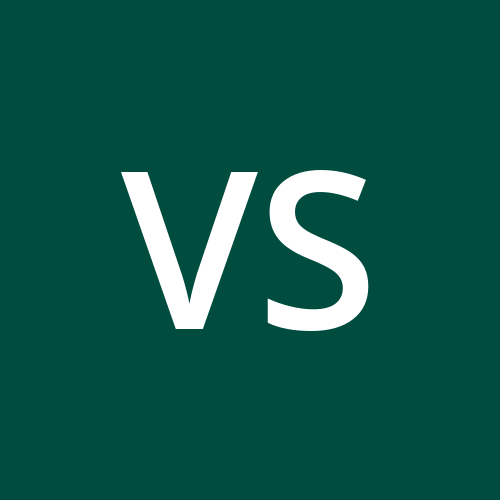