How to Code Rock, Paper, Scissors in Python: A Fun Game for Beginners
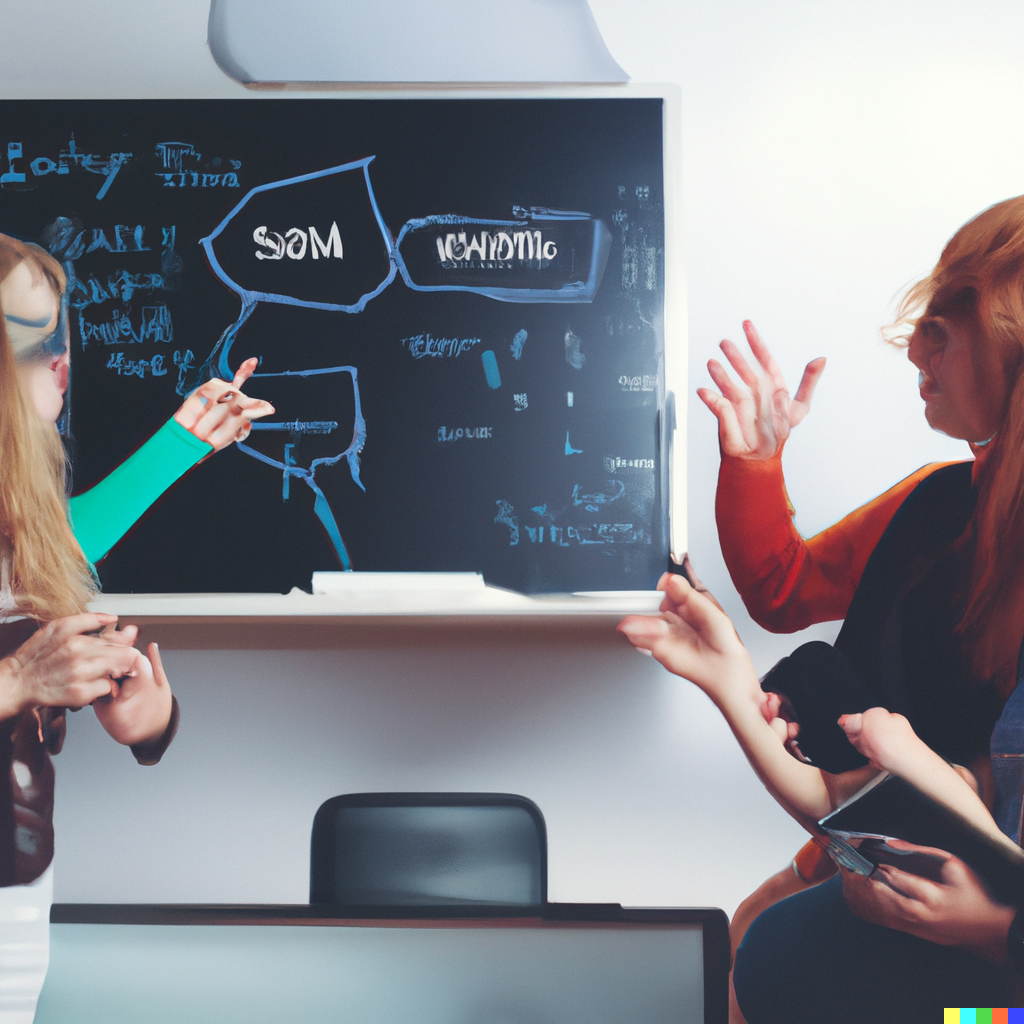
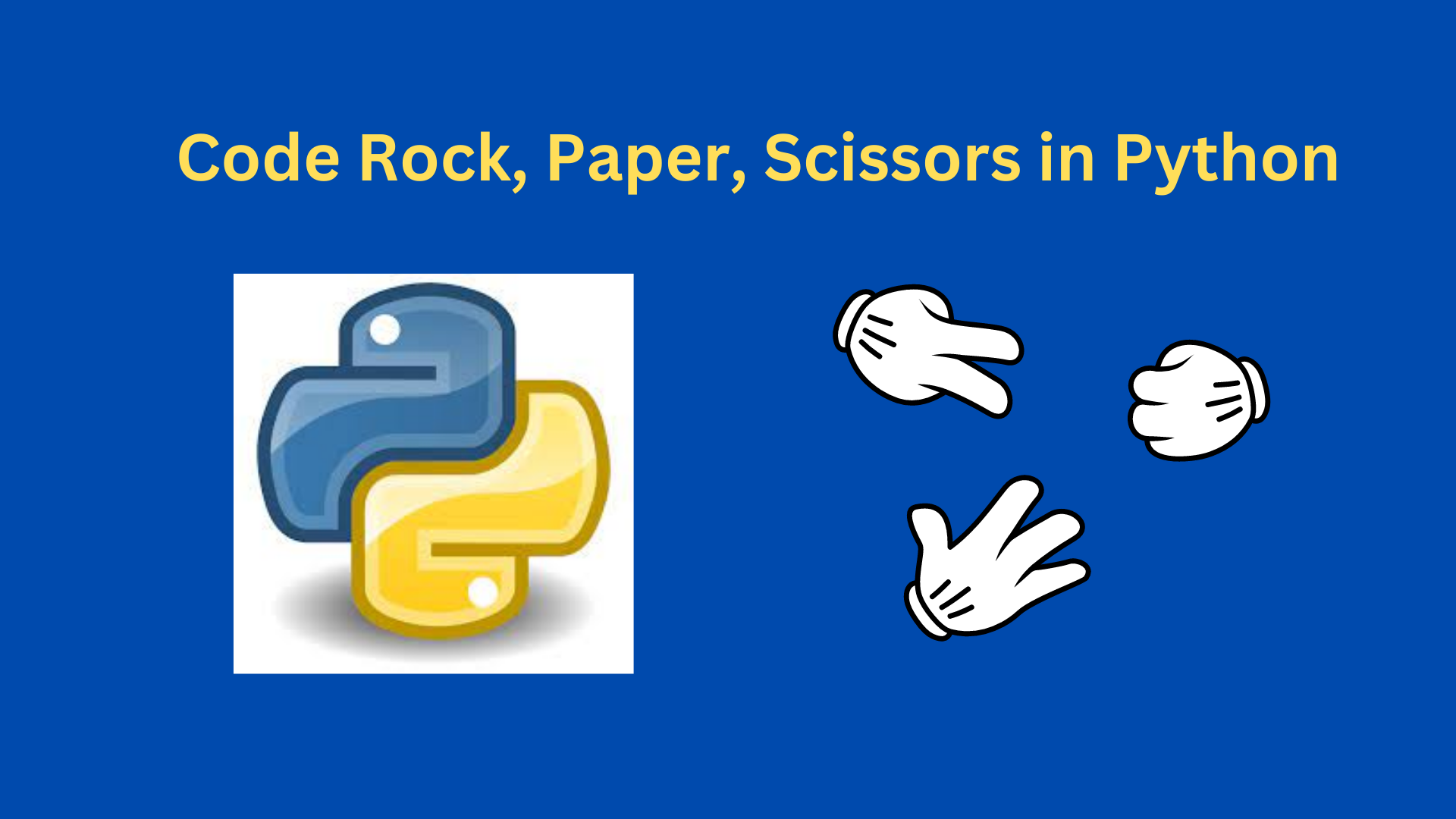
Rock, paper, scissors is a classic game that can be played between two players. The game involves making hand gestures representing rock, paper, or scissors. Each gesture beats another in a specific way - rock beats scissors, scissors beats paper, and paper beats rock.
In this Python code, we are going to implement the game for a single player against the computer.
First, we define a function called rock_paper_scissors()
. Inside the function, we create a list of options for the computer to choose from - 'rock'
, 'paper'
, and 'scissors'
.
Next, we ask the user for their choice by using the input()
function. The user's input is stored in the user_choice
variable.
After the user makes their choice, the computer selects a random option from the options
list using the random.choice()
function. The computer's choice is stored in the computer_choice
variable.
Then, we print out the user's and the computer's choices using print()
.
Next, we compare the user's choice to the computer's choice to determine the winner. If the user's choice is the same as the computer's choice, we print out "It's a tie!". Otherwise, we use a series of conditional statements to determine the winner. For example, if the user chooses rock and the computer chooses scissors, we print out "You win!".
Finally, we call the rock_paper_scissors()
function to play the game.
Here is the complete code:
import random
def rock_paper_scissors():
options = ['rock', 'paper', 'scissors']
user_choice = input("Enter your choice (rock/paper/scissors): ")
computer_choice = random.choice(options)
print("You chose: ", user_choice)
print("Computer chose: ", computer_choice)
if user_choice == computer_choice:
print("It's a tie!")
elif (user_choice == 'rock' and computer_choice == 'scissors') or \
(user_choice == 'paper' and computer_choice == 'rock') or \
(user_choice == 'scissors' and computer_choice == 'paper'):
print("You win!")
else:
print("Computer wins!")
rock_paper_scissors()
Save this above code as rps.py and then execute the below code to play Rock, Paper and Scissors with Computer!
python3 rps.py
Playing this game is a fun way to practice using conditional statements and the random
module in Python. You could also extend this game by adding a loop to allow the user to play multiple rounds or by creating a two-player version of the game.
Subscribe to my newsletter
Read articles from Smart Shock directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by
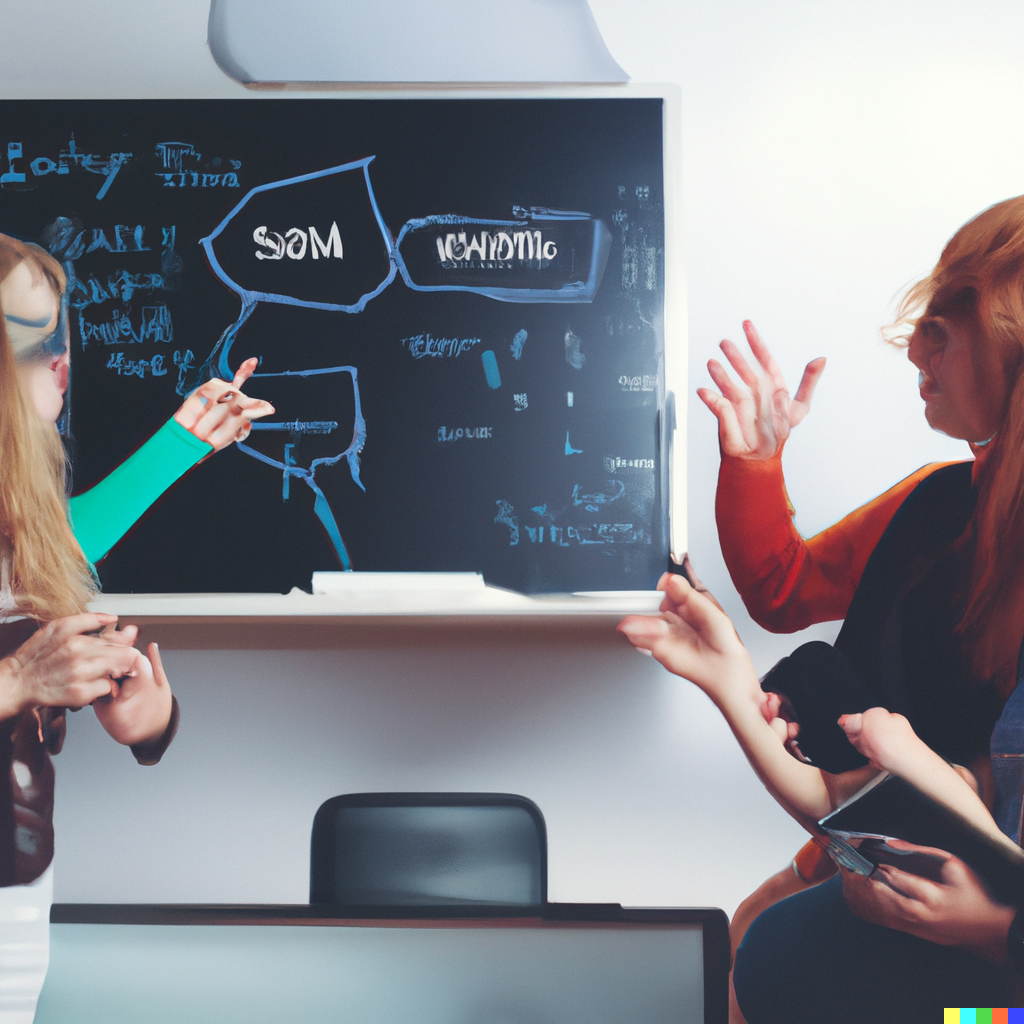