Introduction to C# Programming
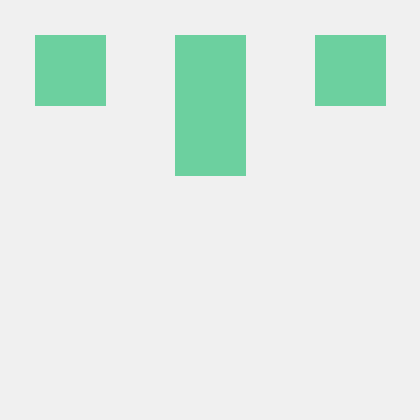
What is C
C# is a high-level programming language developed by Microsoft as one of the languages in the .NET framework. Other languages in .NET include F# and VB.NET. .NET provides an infrastructure on which these languages can be used to develop applications that run on various platforms like Windows, Linux, and macOS.
The Architecture of .NET Applications
.NET consists of two main components:
I. The Common Language Runtime (CLR).
II. Class Libraries.
CLR (Common Language Runtime)
CLR is a runtime environment responsible for executing managed code written in any of the .NET framework languages, including C#. It offers services such as memory management, thread management, type safety, security, and exception handling. When a C# code is executed, it is not converted to a machine-native language; instead, it is converted to an intermediate language known as CIL (Common Intermediate Language), which is independent of the machine it is running on. Then, CLR translates CIL to a native language, which a computer can understand, through a process called JIT (Just-In-Time) compilation. This architecture eliminates the need to convert your app into native languages for different machines.
Class Libraries
Class libraries are blocks of codes called classes that work together at runtime, giving the application its functionalities. A class is a container with some data called attributes and functions also called methods. A class may contain many functions, each designed to perform specific tasks in the application. C# classes are organized and contained in namespaces, which group related classes performing different tasks. For example, you can have a namespace for databases, security, graphics, etc. The C# namespaces are then organized in an assembly, which is a container of namespaces. An assembly is also referred to as a Dynamically Linked Library (DLL).
C# Variables and Constants
A variable is a name given to a storage location in memory, while a constant is an immutable value (mostly used to create safety).
Variable Declaration
When declaring a variable, we start with a type followed by an identifier and finally a semicolon.
For Example:
int number;
In the example above, "int" is a C# data type (integer), while "number" is the identifier. At times, you may need to give a variable a value.
For Example:
int number = 1;
This is called instantiation. Every variable needs to be instantiated before it can be used in an application.
C# Identifier Don'ts
C# language doesn't allow the following ways of naming identifiers:
- Cannot start with a number.
For example:
int 4number;
Instead, use:
int fournumber;
- Cannot include whitespace.
For Example:
string first name;
Instead, use:
string firstname;
- Cannot be a keyword.
For Example:
string int;
Instead, use:
string @int
It is important to use meaningful names as you name identifiers.
For Example:
When naming a string, use:
string firstname;
instead of:
string fn;
Naming Conventions
Note the casing. There are two types of naming conventions used in C#:
I. Camel case:
For Example:
string firstName;
II. Pascal Case:
For Example:
const FirstName;
C# Primitive Types
.NET has the following primitive types:
I. Integral numbers
II. Real numbers
III. Characters
Integral numbers include:
Byte.
Short.
Int.
Long.
Real numbers include:
Float.
Double.
Decimal.
Characters include:
Char.
Bool.
Non-primitive types.
They include the following:
I. String
II.Array
III. Enum
IV. Class
Uses of C#
Backend development of web applications and services.- Building desktop applications.
Game development is used together with Unity game Engine.
Development of mobile applications.
Subscribe to my newsletter
Read articles from Samuel Kamau directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by
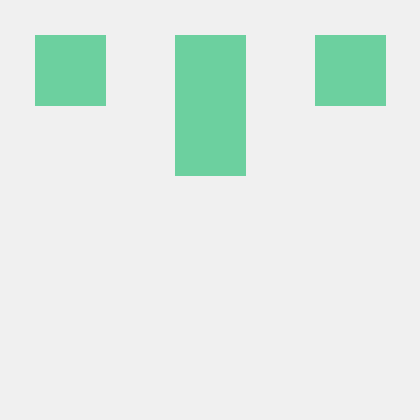