React Fragments: Clean and Efficient UI Grouping in React
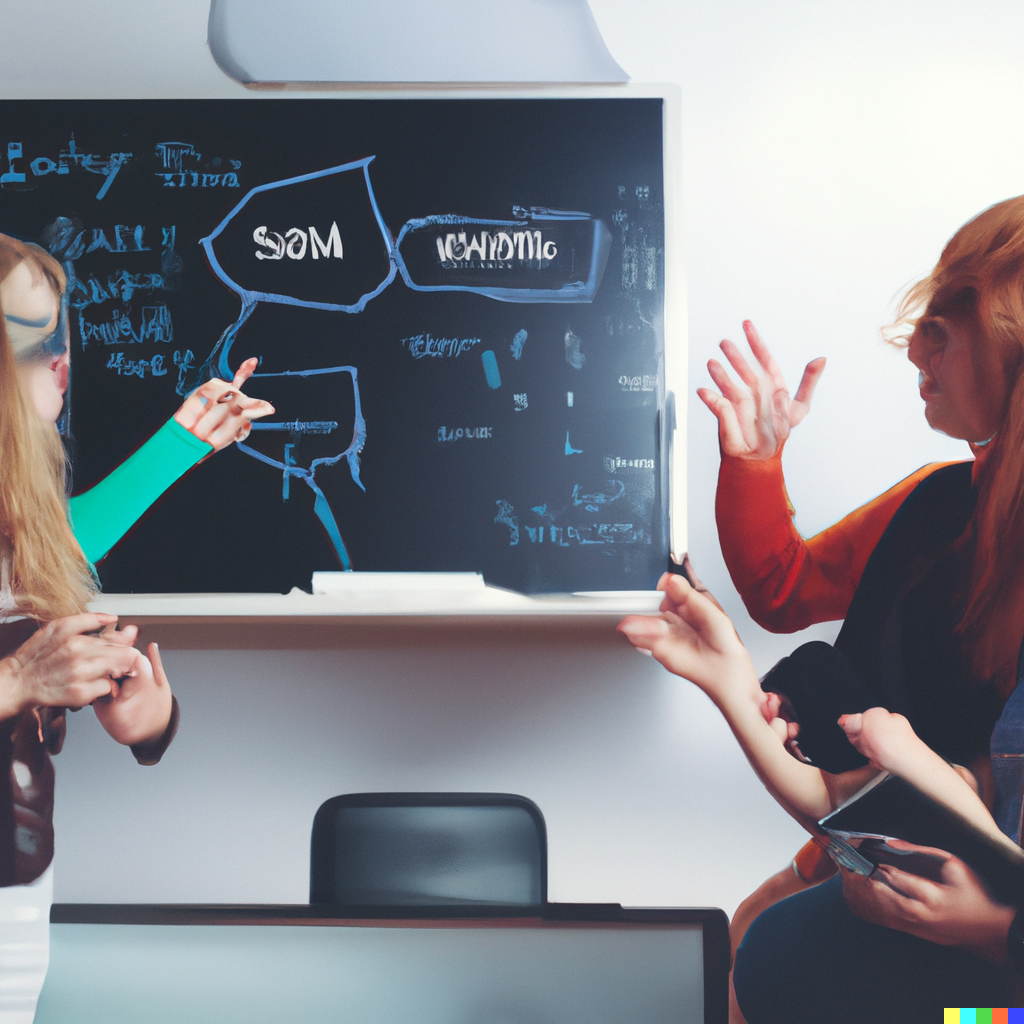
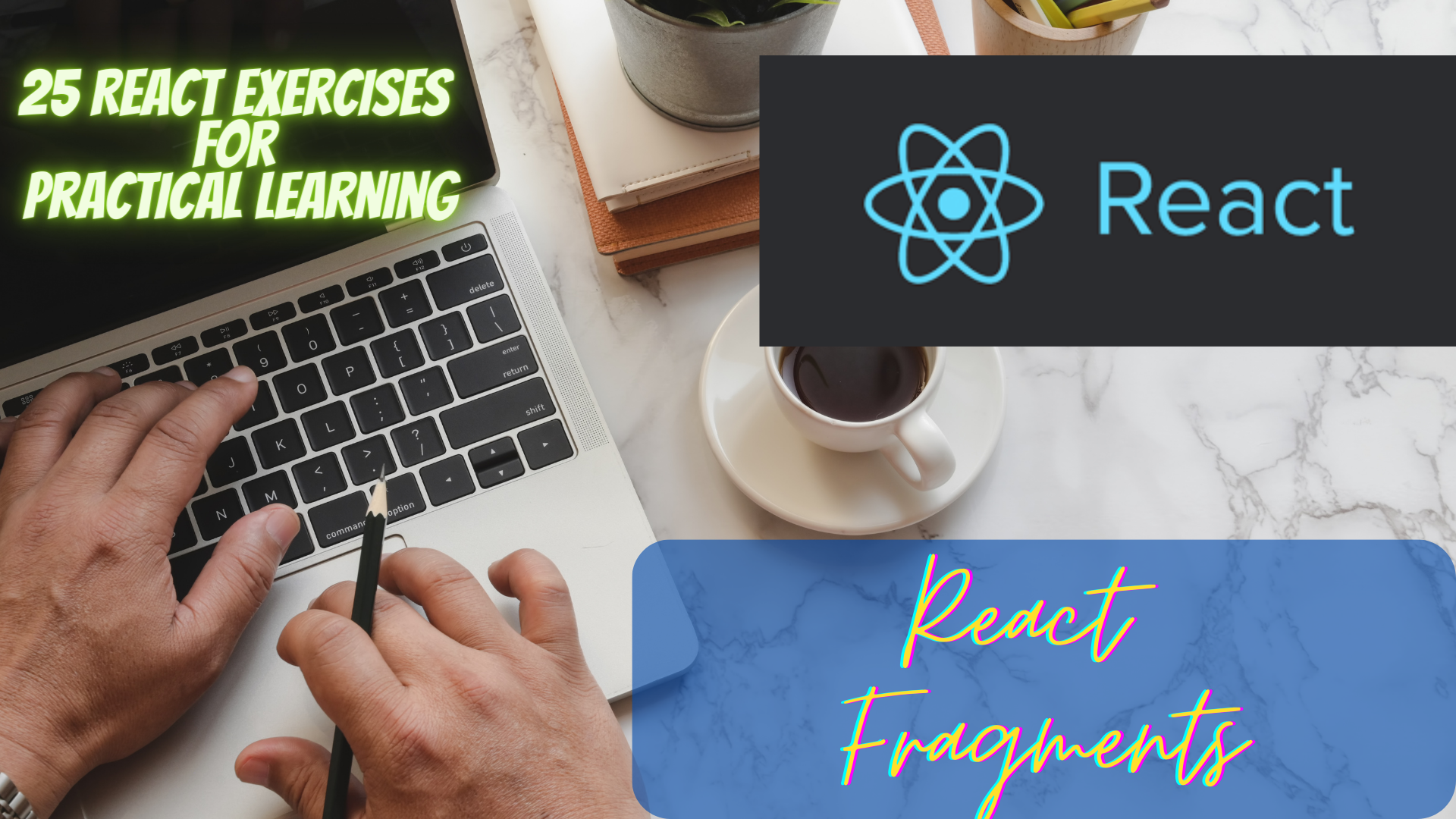
"React Fragments" is a feature in React that allows you to group a list of children elements without adding extra nodes to the DOM. In other words, it's a way to avoid adding unnecessary wrapper elements to your HTML markup.
Let's take a look at an example code snippet:
import React from "react";
function App() {
return (
<>
<h1>React Fragments Demo</h1>
<p>This is a simple demo of React Fragments.</p>
</>
);
}
export default App;
In this example, we have a simple App
component that uses React Fragments to group together two child elements: an <h1>
tag and a <p>
tag. Notice that instead of wrapping these elements in a single parent element, we're using the <>
and </>
syntax to indicate that they belong together as a group.
Using React Fragments in this way allows us to avoid adding unnecessary divs or other elements to our HTML markup. This can help keep our code cleaner and more readable, while still allowing us to group together related elements.
To replicate this code on your local machine, follow these steps:
Install Node.js and create a new React project using
npx create-react-app my-app
.Replace the contents of
App.js
with the example code above.Run
npm start
to start the development server and view the result in your browser.
Overall, React Fragments are a useful tool for grouping together related elements in a more efficient and readable way. They help us avoid adding unnecessary elements to our HTML markup, while still maintaining a clear hierarchy of child elements.
Subscribe to my newsletter
Read articles from Smart Shock directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by
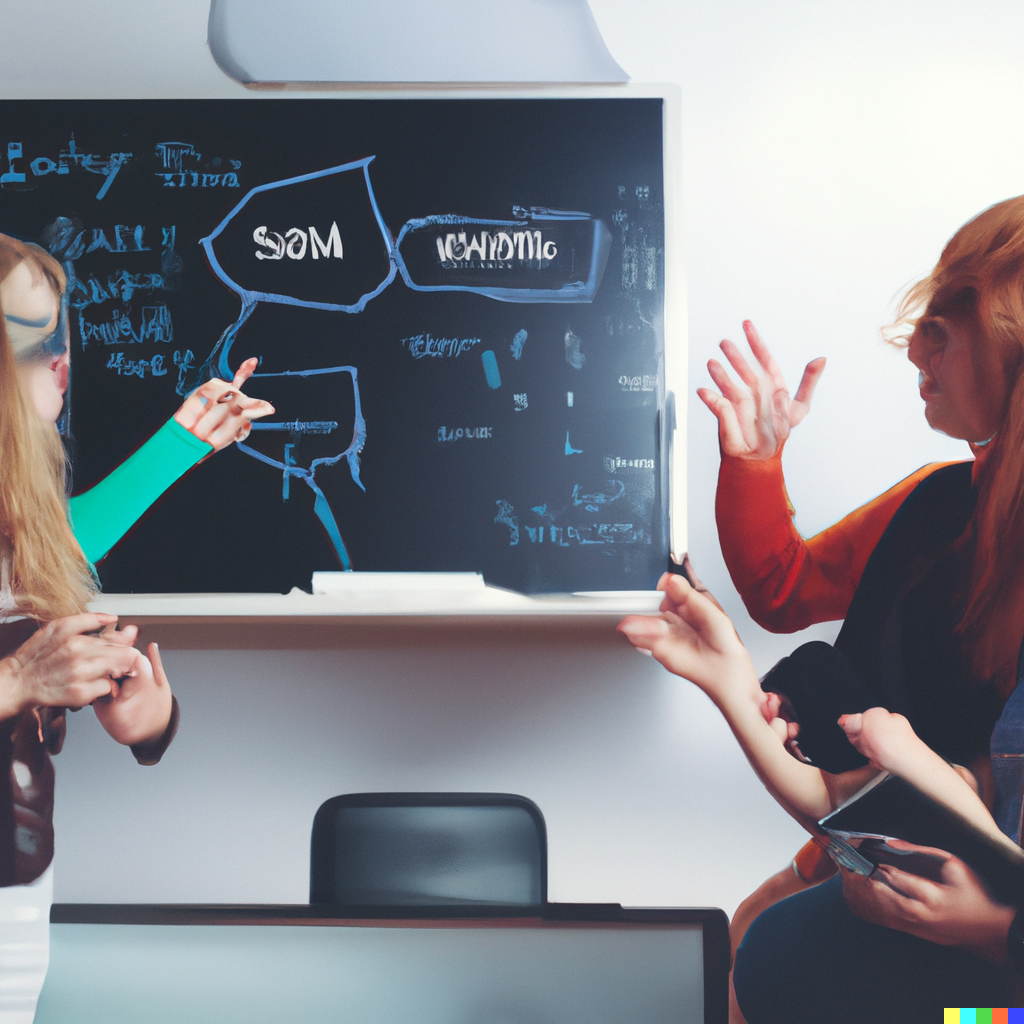