Understanding Uncontrolled Components in React
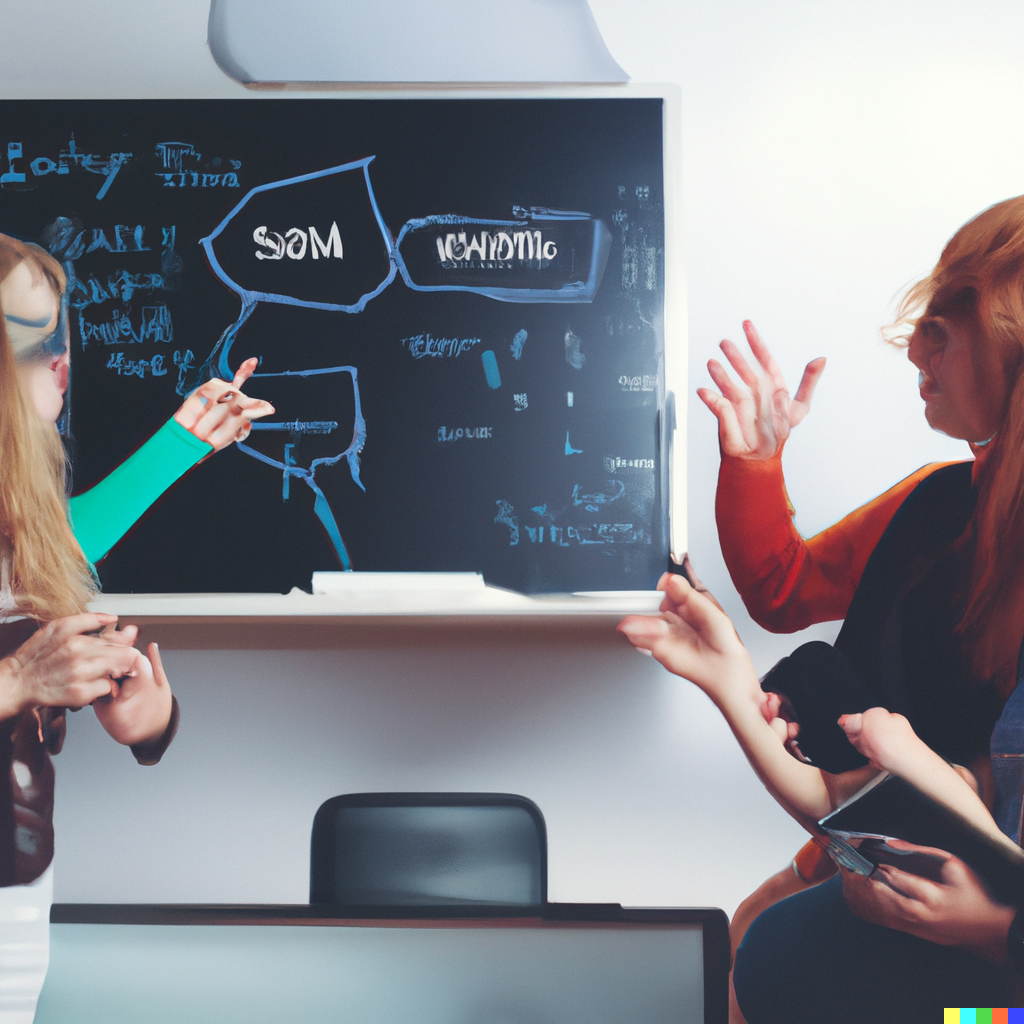
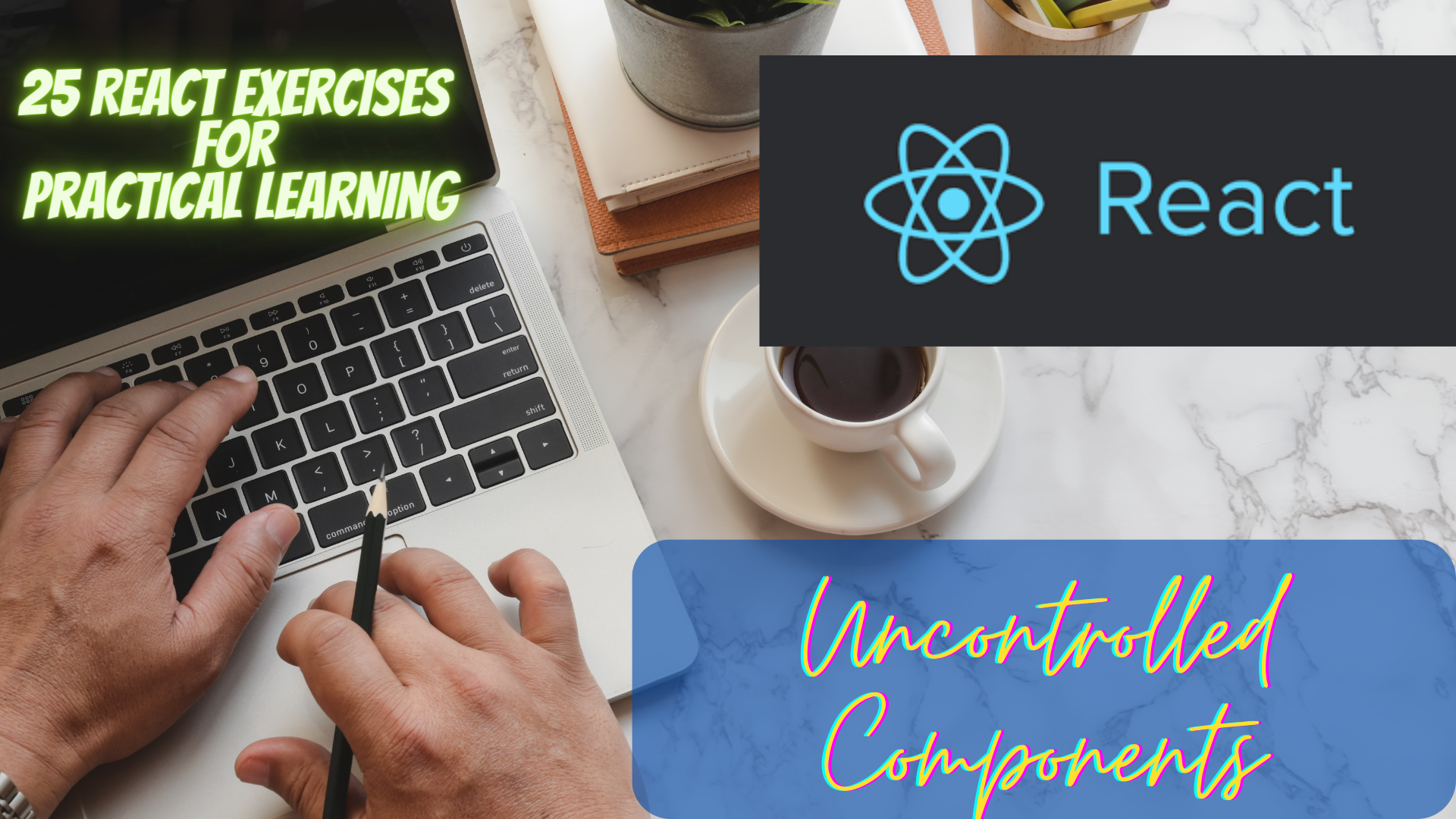
Introduction:
When it comes to form handling in React, there are two types of components - controlled and uncontrolled components. Controlled components are those whose value is managed by React, while uncontrolled components are those whose value is managed by the DOM.
In this article, we'll explore uncontrolled components in detail, including how they differ from controlled components, and when to use them. We'll also go through a simple example of creating an uncontrolled input component and understand how it works.
Let's get started!
Controlled vs. Uncontrolled Components:
Controlled Components:
The value of the input element is managed by React.
The value is stored in React state.
The value can be manipulated using event handlers.
Suitable for complex forms that require data validation, error handling, and other advanced features.
Require more code to implement.
Uncontrolled Components:
The value of the input element is managed by the DOM.
The value can be accessed using the DOM API.
Suitable for simple forms that don't require complex data validation or manipulation.
Require less code to implement.
Now, let's look at an example of an uncontrolled component:
import React, { useRef } from 'react';
function UncontrolledInput() {
const inputRef = useRef(null);
function handleSubmit(event) {
event.preventDefault();
alert(`Input Value: ${inputRef.current.value}`);
}
return (
<form onSubmit={handleSubmit}>
<label>
Enter a value:
<input type="text" ref={inputRef} />
</label>
<button type="submit">Submit</button>
</form>
);
}
export default UncontrolledInput;
In this example, we're creating an uncontrolled input element using the ref
attribute. When the form is submitted, we access the value of the input element using the inputRef.current.value
property.
To replicate this example on your local machine, you can follow these steps:
Create a new React project using
npx create-react-app my-app
.Replace the code in
App.js
with the code above.Start the development server using
npm start
.Open your browser and go to
http://localhost:3000
.Enter a value in the input field and click the "Submit" button.
You should see an alert with the input value.
I hope this example helps you understand the concept of uncontrolled components in React!
Subscribe to my newsletter
Read articles from Smart Shock directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by
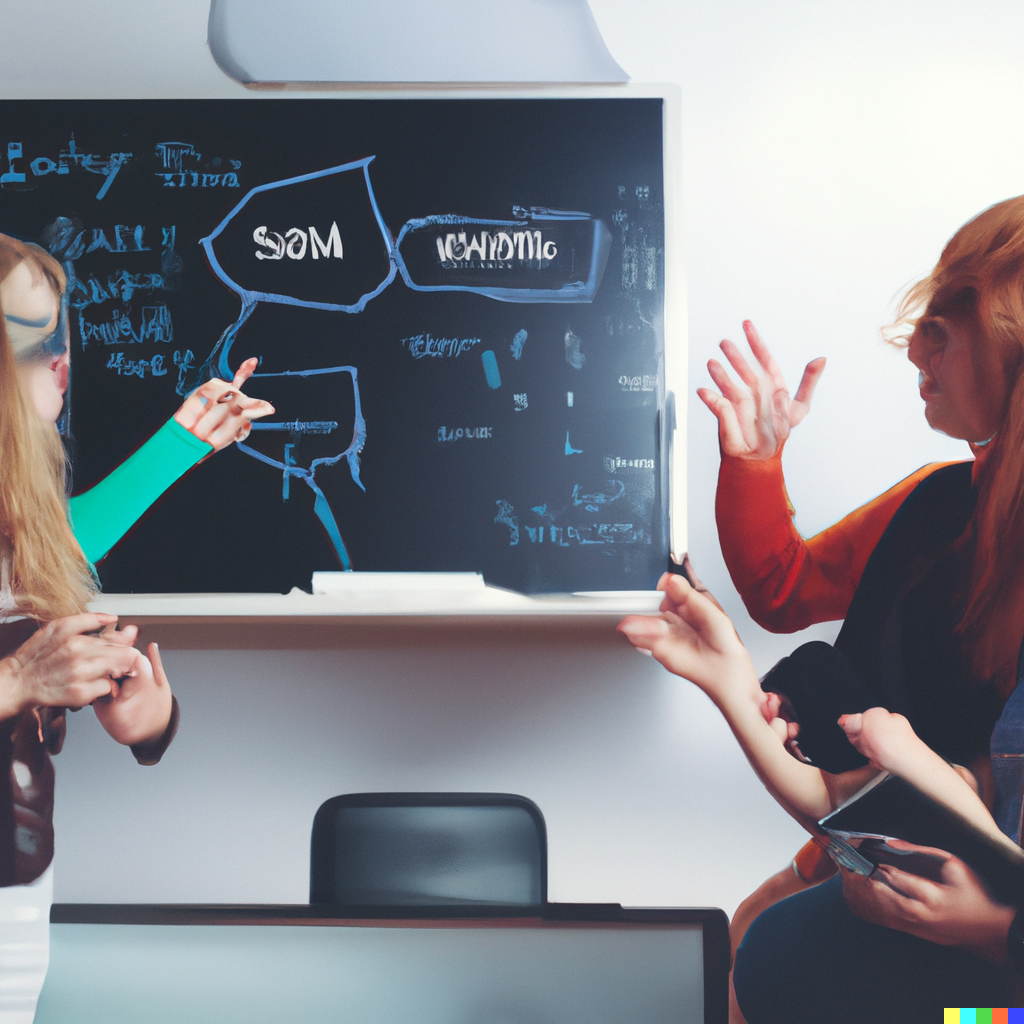