Introducing Kotlin SDK for Novu

Table of contents
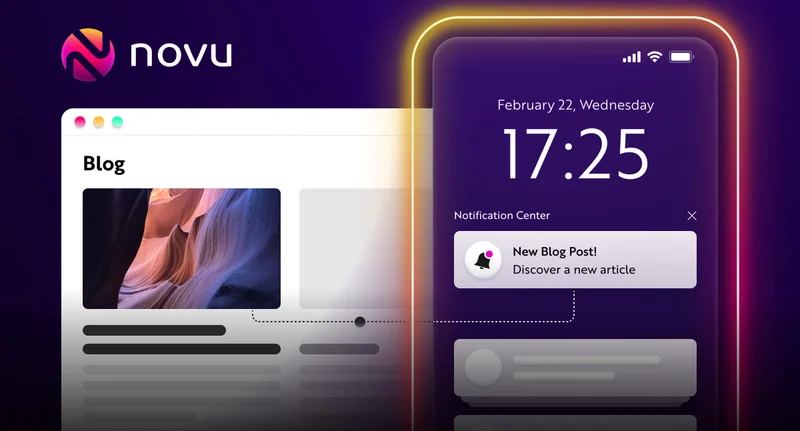
One such library is the Novu client library, which is used for communicating with the Novu API. The Novu API is a powerful tool that allows you to manage notifications and other data in your Novu account.
Why Use Novu? ๐
Developing a notification layer is a common challenge when designing new applications. The process usually starts with sending simple emails, but as the application grows, managing hundreds of notifications across multiple channels becomes cumbersome. Novu's goal is to assist developers in creating meaningful, transactional communication between the product and its users, all while providing an easy-to-use API and outstanding developer experience.
Unified API Today, users receive communication across various providers such as Sendgrid for Email, Twilio for SMS, Mailchimp, Push, Web-Push, and Chat messaging (Slack, etc.). Users expect customization of communication channels to fit their needs and goals. Consequently, developers must manage all of those APIs across the codebase.
Novu solves this challenge by providing a unified API to manage all customer communication. With Novu, developers can add a new provider or switch email providers seamlessly.
Installation
Maven Users
Add Sonatype release/snapshot repositories to your
pom.xml.
<repositories> ... <repository> <id>sonatype</id> <url>https://s01.oss.sonatype.org/content/repositories/releases/</url> // for release or <url>https://s01.oss.sonatype.org/content/repositories/snapshots/</url> // for snapshot </repository> </repositories>
Add Novu dependency in your
pom.xml
dependencies withlatest.version
// add dependency <dependency> <groupId>io.github.crashiv</groupId> <artifactId>novu-kotlin</artifactId> <version>latest.version</version> </dependency>
run
maven install
Gradle Users
Add sonatype release/snapshot repositories to your
build.gradle file
for release versions repositories { ... maven { url "https://s01.oss.sonatype.org/content/repositories/releases/" } or // for snapshot versions maven { url "https://s01.oss.sonatype.org/content/repositories/snapshots/" } }
Add Novu dependency to your
build.gradle
dependenciesimplementation("io.github.crashiv:novu-kotlin:latest.version")
run
gradlew build
Usage โก๏ธ
Once you have installed novu, you can start using it in your Project. The first step is to initialize the client with your API KEY
:
import co.novu.Novu
import co.novu.extentions.environments
fun main()
{
val novu = Novu(apiKey = "API_KEY")
}
Replace API_KEY
with your actual API token. You can find your API token from settings by visiting the Api keys option.
Note: You have to signup on
Novu
to get the API key from their dashboard.
You can then call methods on the client to interact with the Novu API:
After initializing the client, you can call methods on it to interact with the Novu API. For example:
To get a list of subscribers, you can use the following code:
import co.novu.Novu import co.novu.extentions.subscribers fun main() { val novu = Novu(apiKey = "API_KEY") val subscribers = novu.subscribers() println(subscribers) }
This will
return an array of subscriber objects
. You can also pass parameters to this method to filter the results.
Similarly, to trigger an event you can use the following code:
import co.novu.Novu
import co.novu.extensions.subscribers
import co.novu.dto.request.TriggerEventRequest
import co.novu.dto.request.SubscriberRequest
fun main() {
val novu = Novu(apiKey = "API_KEY")
novu.trigger(TriggerRequest.Companion.invoke
(
name = "notificationTemplate",
to = SubscriberRequest(
subscriberId = "harry_potter"
firstName = "Harry",
lastName = "Potter",
phone = "97X98XX98X1",
email = "email@email.com",
loacal = "locale",
avatar = "avatar",
),
payload = mapOf("name" to "Shivam Shah",
"addresss" to "XX XXXX XX XXX XXX")
)
)
}
# HTTP Status Code: 201
# Response Body:
{
acknowledged: true,
status: "status",
error: ["error"],
transactionId: "transactionId"
}
For more information about the available methods and their parameters, you can refer to the Novu API documentation.
Note: When self-hosting Novu, in order to trigger an event you must first create a new Novu
object and configure it with the proper backendUrl
.
import co.novu.Novu
import co.novu.NovuConfig
import co.novu.extensions.subscribers
import co.novu.dto.request.TriggerEventRequest
import co.novu.dto.request.SubscriberRequest
fun main() {
val config = NovuConfig(backendUrl = "http://localhost:4200/")
val novu = Novu(apiKey = "API_KEY",config)
novu.trigger(TriggerEventRequest.Companion.invoke
(
name = "notificationTemplate",
to = SubscriberRequest(
subscriberId = "harry_potter"
firstName = "Harry",
lastName = "Potter",
phone = "97X98XX98X1",
email = "email@email.com",
loacal = "locale",
avatar = "avatar",
),
payload = mapOf("name" to "Shivam Shah",
"addresss" to "XX XXXX XX XXX XXX")
)
)
}
Conclusion ๐
Novu is an open-source notification infrastructure designed to assist engineering teams in creating meaningful, transactional communication between the product and its users, all while providing an easy-to-use API and an outstanding developer experience. By using Novu, developers can simplify the management of multiple communication channels and providers, improving efficiency and productivity. By following the installation and usage instructions provided above, you can start using the library in your Kotlin projects right away.
Cheers,
Shivam Shah
PS. If you find the article relevant, then please share it with your network. ๐
References
Subscribe to my newsletter
Read articles from Shivam Shah directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by
