Mastering GraphQL API : From Basics to Subscriptions and Fragments
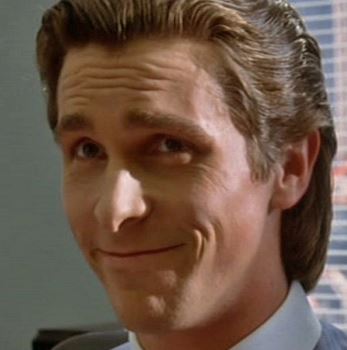
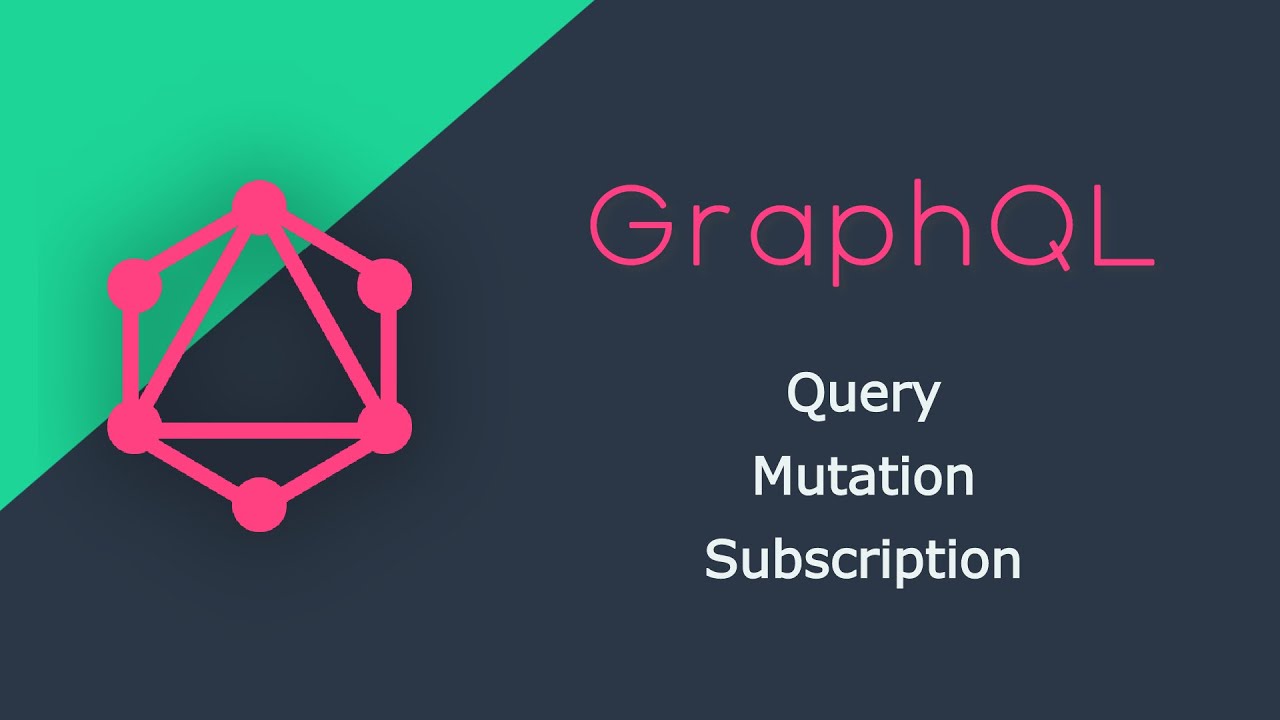
Image Credits : Cached Insights
๐ If you're looking to develop APIs using GraphQL, you're in the right place! ๐ In this tutorial blog series, we'll cover everything you need to know to get started with GraphQL API development. ๐ป We started with the basics, covering what GraphQL is and how it differs from REST APIs in our previous blog post. ๐
In today's post, we'll dive deep into the building blocks of a GraphQL API, including queries, mutations, and scalars. ๐ช We'll also explore how to write a GraphQL schema and how to implement subscriptions using sockets. ๐ Finally, we'll cover fragments, a powerful tool for simplifying complex queries. ๐
To watch the complete series, ๐บ go and checkout my profile โ there you'll find it!
What is SDL? ๐ค
SDL (Schema Definition Language) is the syntax used to define a GraphQL schema. It's used to define the types, fields, and relationships that make up a GraphQL API. SDL is a simple and intuitive syntax that's easy to learn, even for beginners.
Queries ๐
A GraphQL query is used to retrieve data from a GraphQL API. It's similar to a GET request in a REST API. To write a query, you simply specify the data you want to retrieve and the fields you want to retrieve it for. For example:
query {
books {
title
author
publishedYear
}
}
This query retrieves the title, author, and published year of all books in the API. ๐
Mutations ๐จ
A GraphQL mutation is used to modify data in a GraphQL API. It's similar to a POST, PUT, or DELETE request in a REST API. To write a mutation, you specify the operation you want to perform (e.g. create, update, delete), along with any parameters that are required. For example:
mutation {
createBook(title: "The Great Gatsby", author: "F. Scott Fitzgerald", publishedYear: 1925) {
id
title
author
publishedYear
}
}
This mutation creates a new book with the title "The Great Gatsby", author "F. Scott Fitzgerald", and published year 1925. It then returns the ID, title, author, and published year of the new book. ๐
Scalars ๐
Scalars are the basic data types used in GraphQL. They include strings, integers, floats, Boolean, and IDs. Scalars are used to define the types of fields in a GraphQL API. ๐
GraphQL Schema ๐
A GraphQL schema is a collection of types and fields that define the structure of a GraphQL API. It's defined using SDL syntax and provides a blueprint for the data that can be retrieved or modified using the API. Writing a schema is straightforward, and can be done using simple syntax like this:
type Book {
title: String!
author: String!
publishedYear: Int!
}
type Query {
books: [Book!]!
}
In this example, we're defining a Book
type that has three fields: title
, author
, and publishedYear
. We're also defining a Query
type that has a single field called books
, which returns an array of Book
objects. ๐
Subscriptions ๐ฉ
Subscriptions allow us to receive real-time updates from a GraphQL API. They're often used in applications where we need to display live data, such as chat applications or real-time dashboards. In GraphQL, subscriptions are implemented using sockets, which provide a persistent connection between the client and server. Here's an example of a subscription that listens for new books being added to our database:
subscription {
newBook {
id
title
author
publishedYear
}
}
In this subscription, we're listening for the newBook
event, which will be triggered every time a new book is added to the database. When the event is triggered, we'll receive the ID, title, author, and published year of the new book.
Fragments
Fragments are a powerful tool for simplifying complex queries in GraphQL. They allow you to define reusable blocks of query syntax that can be included in multiple queries. This can make queries more concise, easier to read, and more maintainable.
To define a fragment, you simply specify the type and fields you want to include. For example:
fragment BookDetails on Book {
title
author
publishedYear
}
This fragment defines the BookDetails
fragment, which includes the title
, author
, and publishedYear
fields for a Book
object. Once defined, this fragment can be included in any query that returns a Book
object, like this:
query {
books {
...BookDetails
}
}
mutation {
createBook(title: "The Great Gatsby", author: "F. Scott Fitzgerald", publishedYear: 1925) {
...BookDetails
}
}
In this example, we're including the BookDetails
fragment in both a query and a mutation, making our code more concise and easier to read.
Conclusion
That's it for our introduction to building a GraphQL API! We've covered the basics of SDL, queries, mutations, and scalars, as well as more advanced topics like subscriptions and fragments. With this knowledge, you should be well-equipped to start building your own GraphQL APIs. Stay tuned for more tutorials in this series, where we'll dive deeper into GraphQL development and cover more advanced topics.
Subscribe to my newsletter
Read articles from Shubhom directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by
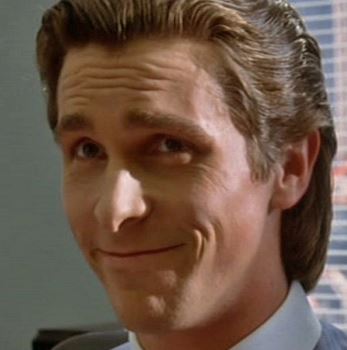