Making some changes in a cricket team using splice() Method in Javascript
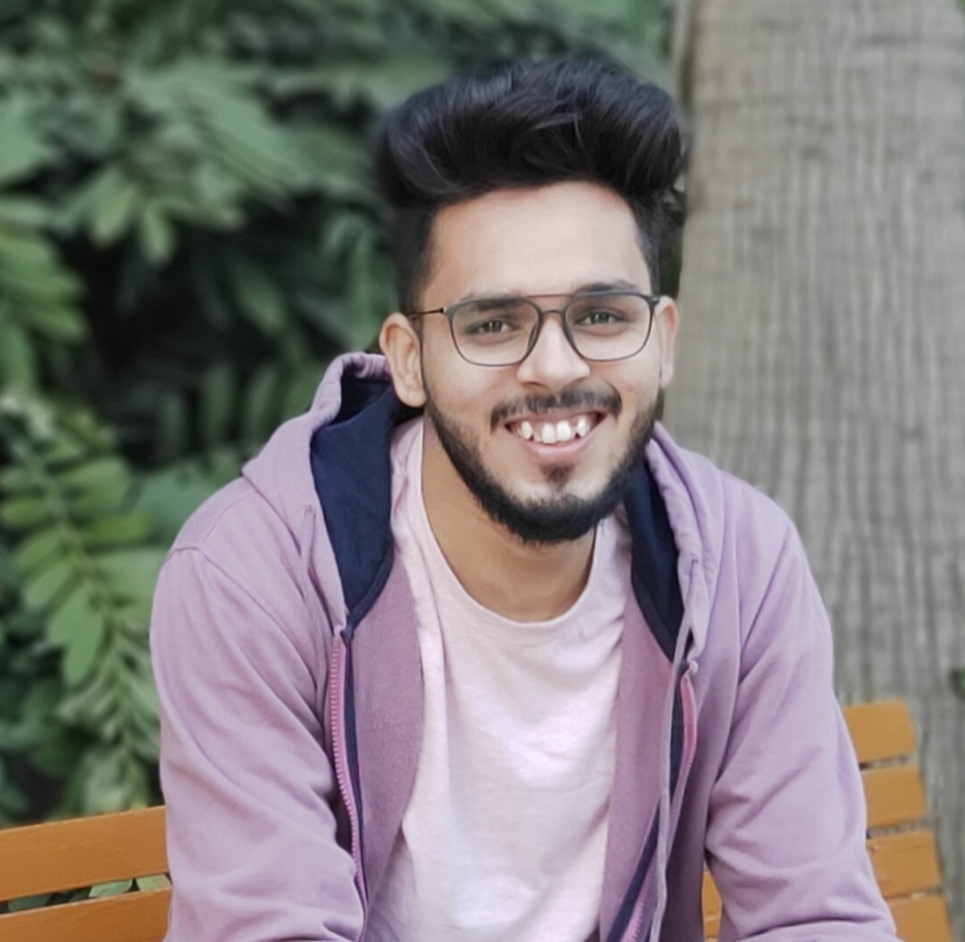
Once again, the cricket season had begun and Ankit, the captain of a local cricket team, was all geared up for a thrilling tournament. But this time, he was faced with a challenge. He gathered 10 players and was struggling to find the last player in the team. His current team list was stored in an array like this:
// The initial team array of 10 players
let team = ['Prashant', 'Ankit', 'Fahad', 'Bhanu', 'Ziyad', 'Arbab', 'Monish', 'Rafey', 'Aryan', 'Irfani'];
Ankit wanted to build a strong team, but the situation got worse when he came to know that their veteran out-of-form middle-order batsman, Ziyad tripped over a cat and broke his foot. The cat is fine though ๐. Ankit realised that they were lacking a power hitter who could hit the ball out of the park and a spinner who could bamboozle the opposition batsmen.
He remembered Rameez aka Sasta Dhoni of the town, who had a reputation for hitting big sixes at will. He also recalled Saarthak aka Kachra (reference from the movie Lagaan), who had a unique way of bowling spin.
Ankit wanted to add Rameez and Saarthak to the team list and remove Ziyad. He knew that there are several methods available in Javascript to manipulate arrays, such as push()
, pop()
, shift()
, and unshift()
, but none of these methods could help him do his desired operation in one go. He opened a new tab on his browser and read about splice()
method from the MDN web docs.
The splice()
method allows you to add or remove elements from an array at a specific index. It takes three arguments: the starting index to remove, the number of elements to remove, and any new elements to add.
Let's look at how splice()
method helped Ankit to complete his cricket team:
console.log(team); // ['Prashant', 'Ankit', 'Fahad', 'Bhanu', 'Ziyad', 'Arbab', 'Monish', 'Rafey', 'Aryan', 'Irfani']
// The index where Ziyad is located
let indexToRemove = 4;
// The number of elements to remove
let numberOfElementsToRemove = 1;
// The elements to add
let elementsToAdd = ['Rameez', 'Saarthak'];
// Using the splice method to remove Ziyad and add Rameez and Saarthak
team.splice(indexToRemove, numberOfElementsToRemove, ...elementsToAdd);
console.log(team); // ['Prashant', 'Ankit', 'Fahad', 'Bhanu', 'Rameez', 'Saarthak', 'Arbab', 'Monish', 'Rafey', 'Aryan', 'Irfani']
In the above code, Ankit first identified the index of Ziyad in the team
array. Then, he used the splice
method to remove one element from the team
array starting at the index of Ziyad and adding Rameez and Saarthak to the team
array using the spread operator ...
.
Now, the team had a much-needed power hitter and an excellent spinner. Ankit was confident that the team would perform well in the upcoming tournament with the help of these two new players.
In conclusion, the splice
method in JavaScript is a powerful tool for adding or removing elements from an array. In Ankit's case, he was able to add two players to the team using this method and improve the overall strength of the team.
Subscribe to my newsletter
Read articles from Fahad Masood directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by
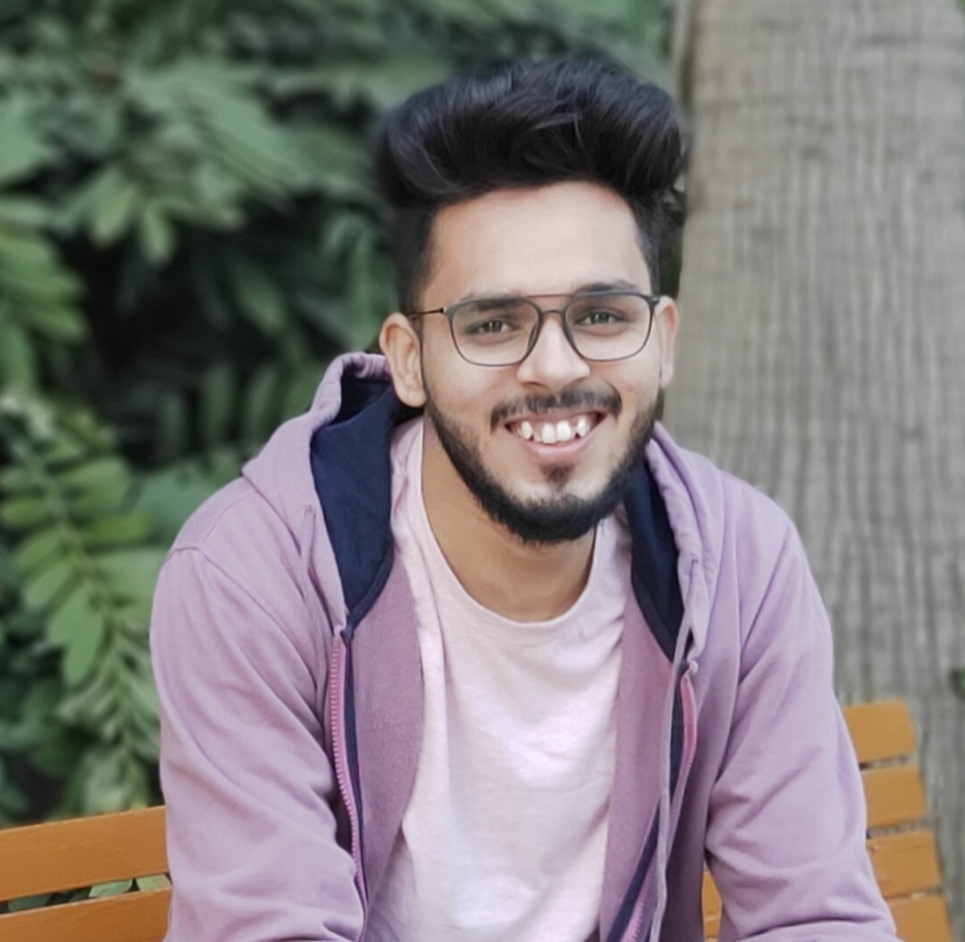
Fahad Masood
Fahad Masood
I am a Front End developer with industry experience building websites and web applications. I specialize in JavaScript and have professional experience working with HTML, CSS, React, Next, and Prismic. My job is to transform raw data into a graphical interface in such a way that users can view and interact with the data. I have a good understanding of SEO principles, Agile Methodologies, Git, and OOP concepts.