Program to insert an element at the Bottom of a Stack

Table of contents
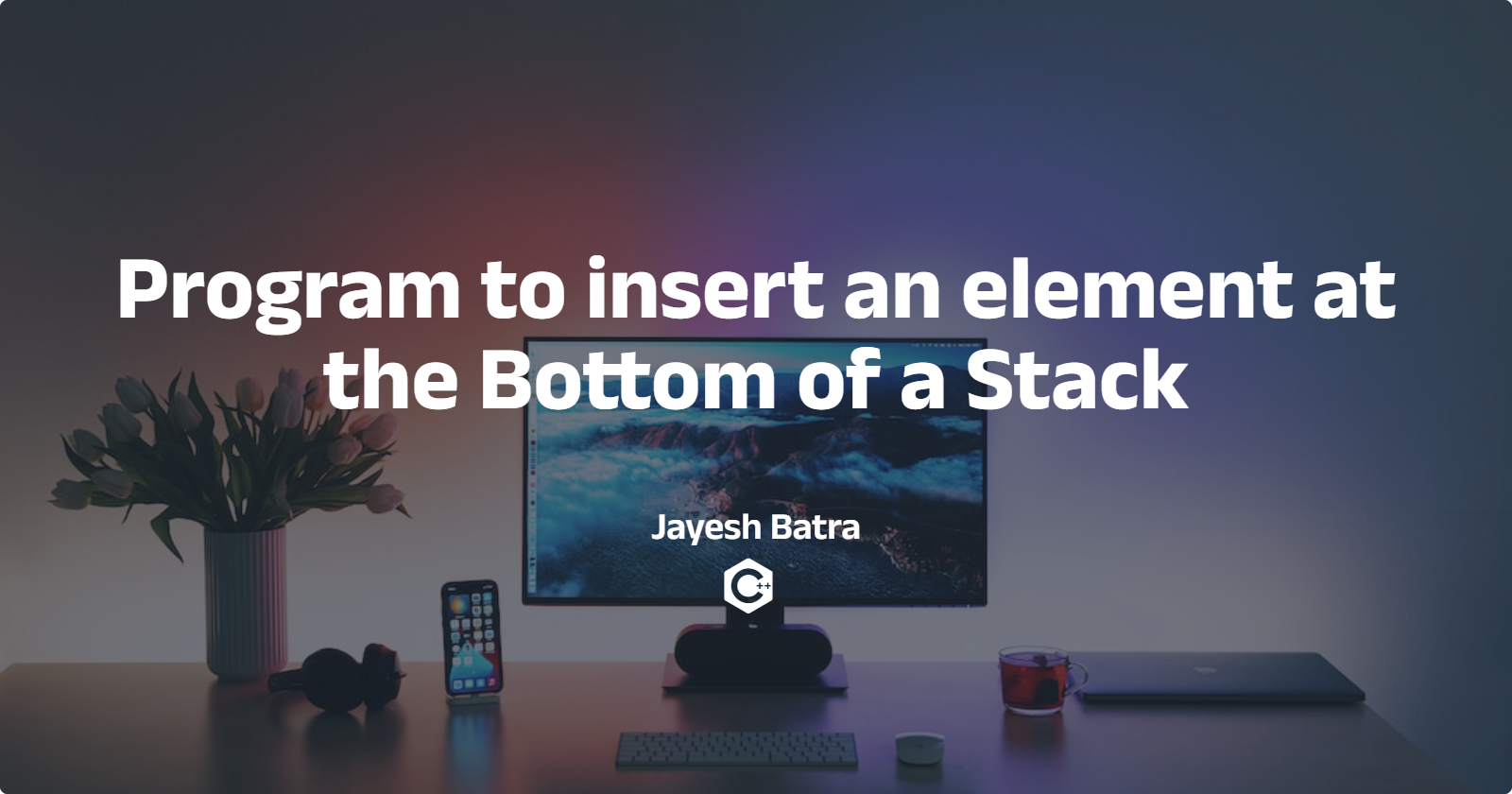
So hey, in this article we are going to learn to insert an element at the Bottom of a Stack
Question Link: https://practice.geeksforgeeks.org/problems/insert-an-element-at-the-bottom-of-a-stack/1
Question Explanation
Inserting an element at the bottom of a stack refers to a specific operation where a new element is added to the bottom-most position in a stack, effectively pushing all the existing elements up by one position. This operation is typically implemented using a recursive approach, as demonstrated in the code provided in the previous response.
Code
void solve(stack<int>& s, int x) {
//base case
if(s.empty()) {
s.push(x);
return ;
}
int num = s.top();
s.pop();
//recursive call
solve(s, x);
s.push(num);
}
stack<int> pushAtBottom(stack<int>& myStack, int x)
{
solve(myStack, x);
return myStack;
}
Code Explanation
The given code implements a function pushAtBottom
that inserts an element x
at the bottom of a stack myStack
using a recursive approach.
The function solve
is a recursive helper function that pops elements from the stack s
until it becomes empty, and then pushes the element x
to the stack. The recursion is used to temporarily remove all the elements from the stack, and then push them back after the desired element x
has been inserted at the bottom.
Here's how the code works:
The base case of the recursion is when the stack
s
is empty. In that case, the elementx
is directly pushed to the stack usings.push(x)
, and the function returns.Otherwise, an element
num
is popped from the top of the stack usings.top
()
ands.pop()
. This element is temporarily stored to be pushed back later.The function
solve
is recursively called with the same stacks
and the elementx
.After the recursion completes, the element
num
is pushed back to the stack usings.push(num)
. This ensures that elementx
is inserted at the bottom of the stack, as all the elements above it have been temporarily removed and pushed back.Finally, the
pushAtBottom
function callssolve
with the given stackmyStack
and the elementx
, and returns the modified stackmyStack
with the elementx
inserted at the bottom.
This code can be useful in scenarios where you need to insert an element at the bottom of a stack, which can be used in various stack manipulation operations or algorithms. It's important to note that using recursion for stack manipulation should be done with caution to avoid stack overflow errors, and proper handling of edge cases and error conditions should be considered in practical implementations.
Subscribe to my newsletter
Read articles from Jayesh Batra directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by

Jayesh Batra
Jayesh Batra
I am a passionate and motivated individual with a strong desire to excel in the field of software development. With my current focus on MERN stack, DSA, and open source, I am well-positioned to make valuable contributions to any project or team I work with.