Integrating Firebase into Flutter Project
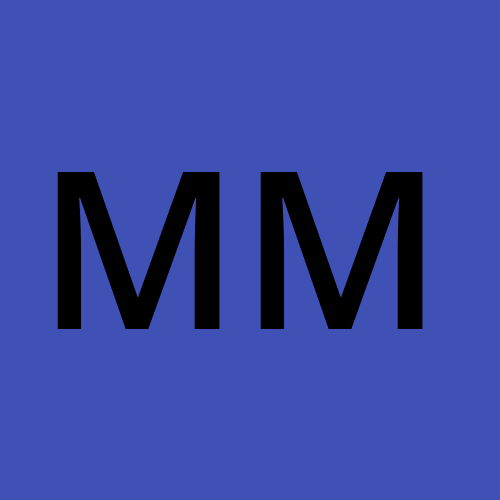
This semester Mobile App Development was one of the courses I had to take and I can not stress how much I did not enjoy it. I have never been a fan of anything user interface related and a large part of the curriculum was understanding Flutter UI widgets. So when it was time to integrate Firebase into our apps a little interest was sparked.
Flutterfire vs Google console
Now a number of tutorials show how to set up Firebase from the console. This includes editing configuration files, and writing to them manually. Guess what? That could not be more annoying to me. So when I came across Flutterfire CLI I almost giggled in joy(Almost because I had lemon tea in my mouth, irrelevant).
I find using the CLI so much better because all the configuration is done for you. With just one command your app gets configured for as many platforms as you choose(automation).
In creating this tutorial I made the following assumptions:
You understand how to create a Flutter project and you already have one running
You have a Firebase account. If not kindly google how to create one.
You are using a Windows computer. Unfortunately, I did not test any of these steps on my Linux machine.
USING FLUTTER-FIRE CLI
Firstly install Firebase locally. Open your terminal and run the following command.
npm install firebase
Verify your installation
firebase --version
If the installation was a success you will get the version back. My first time running the above command I got an error about running scripts is disabled. I typed developer settings on the start menu. Make sure that is turned on as well as the below-shown settings.
When you try running firebase --version
you should get the version back.
Now let's also install Flutterfire CLI. I used the following command
dart pub global activate flutterfire_cli
If you get a warning about dart adding the Flutterfire executable to C:\Users\<your username>\AppData\Local\Pub\Cache\bin, which is not on your path
just copy that path and add it to your environment variable Path
Run
firebase login
This will redirect you to the browser and you will be prompted to log into your Firebase account. Once the login is done, return to your terminal where you will be met with a success message.
Now you can open your app folder, and run the following command:
flutterfire configure
The above command does the following:
Fetches all available projects on your Firebase account.
You are then prompted to choose one project or create a new one.
Once you have selected a project to work with, you will be prompted to select a platform: web, iOS, MacOS, or Android. By default, all platforms are selected.
After choosing your desired platforms, flutter-fire will configure your entire project, adding app identity etc.
Run the following command which installs the core dependencies of Firebase.
flutter pub add firebase_core
Upon completion, you can also add other Firebase dependencies such as firebase_auth
After this, go into your main.dart
file and make the following imports.
import 'package:flutter/material.dart';
import 'package:firebase_core/firebase_core.dart';
import 'firebase_options.dart';
After this replace your current runApp code with the following.
Future<void> main() async {
WidgetsFlutterBinding.ensureInitialized();
await Firebase.initializeApp(options: DefaultFirebaseOptions.currentPlatform);
runApp(MyApp());
}
The above code snippet replaces the one below.
void main() {
runApp(const MyApp());
}
flutter run
If everything is installed properly, this should be running. If not, Sorry. I tried my best, and all I can is that It worked on my computer.
Side note: Installing Firebase and integrating it into our work is not just for decorations. The next stop is going to apply Firebase in user authentication.
I hope this tutorial made it easy to integrate Firebase into the app.
Subscribe to my newsletter
Read articles from Millicent Malinga directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by
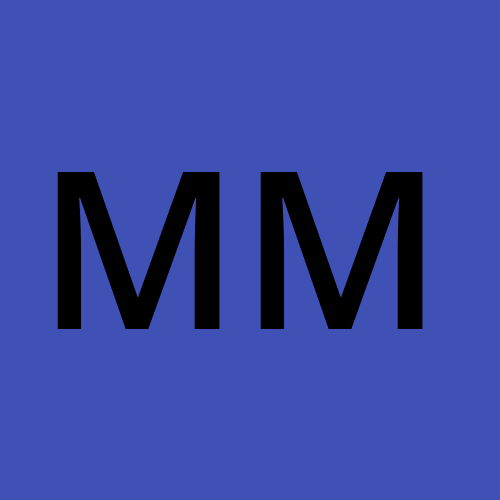