Using dart to fetch your top tracks from Spotify Web API
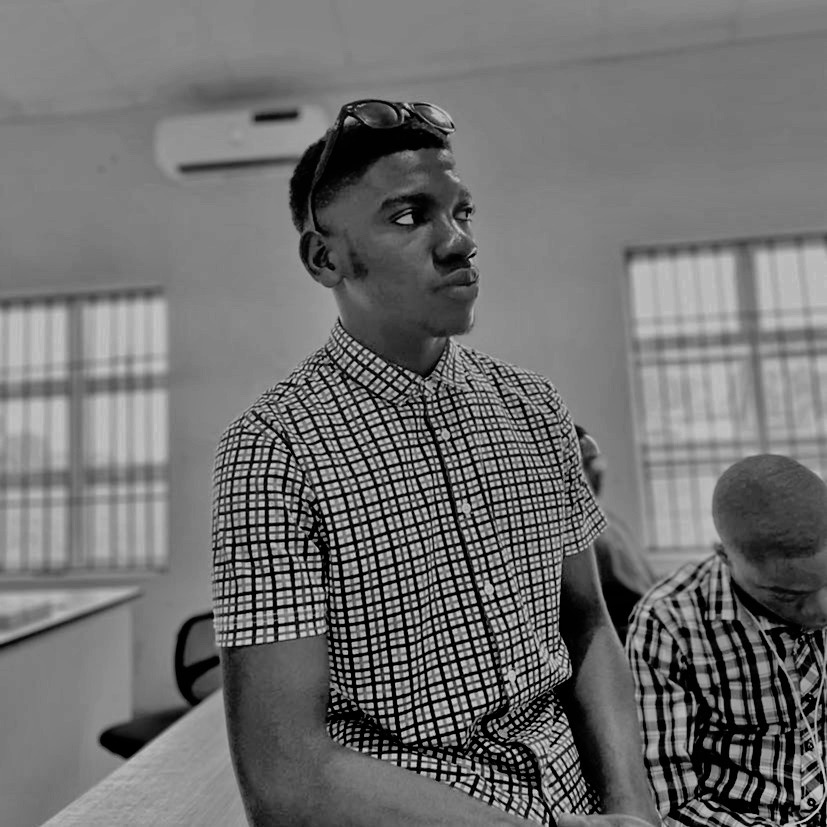
Table of contents
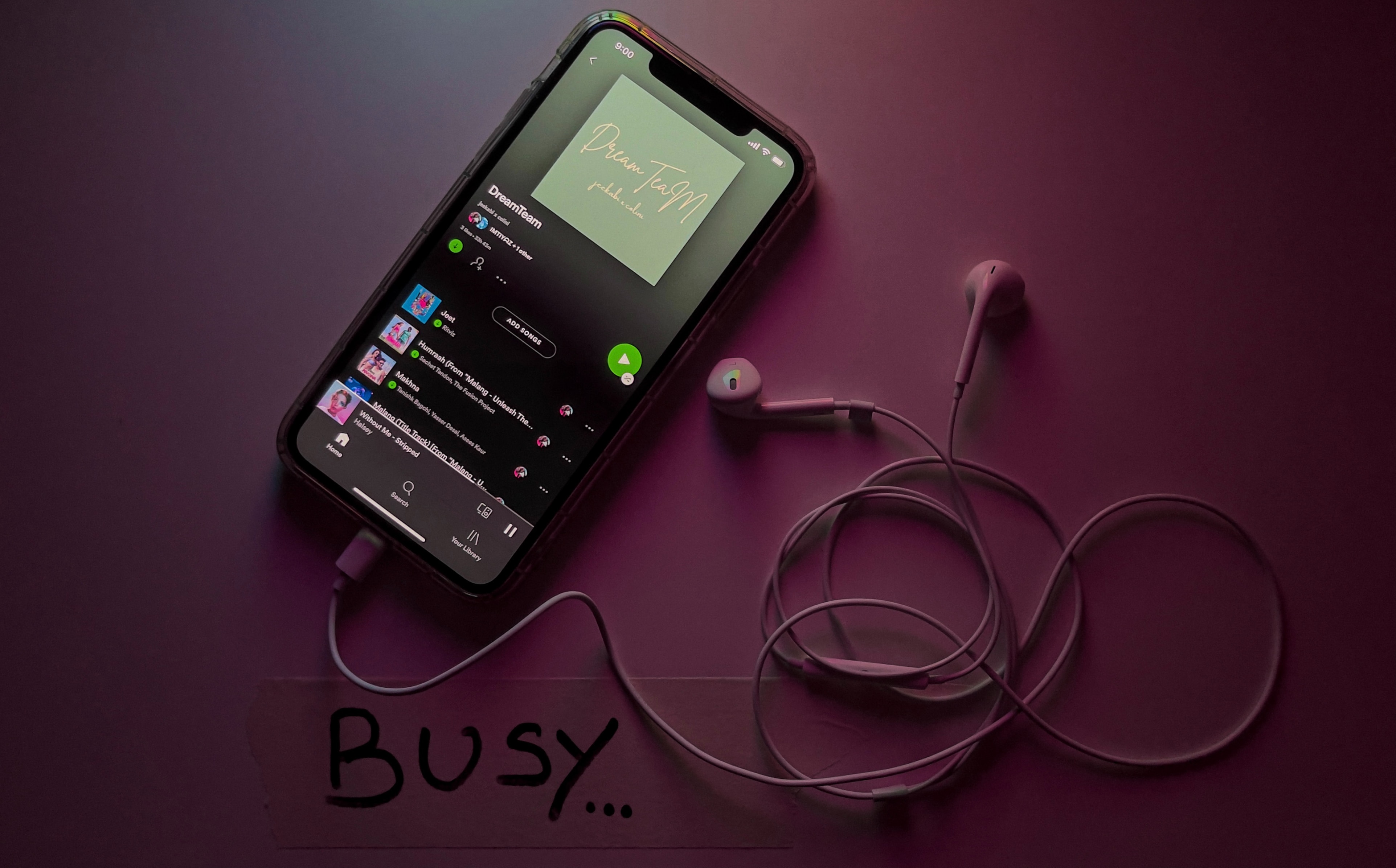
A while ago I was going through a C# code a wrote which was a code to fetch top tracks from a particular artist (ASAKE) Nigerian artist of course ☺️
Still haven't finished that hopefully I do complete it anytime soon.
Then I saw a javascript method used to fetch top tracks from Spotify user with their authorized tokens from Spotify. Why not implement it with Dart so we can use them in ourFlutter apps... 🤷🏼♂️
Let's get to it🚀
First we import our packages right?
import 'dart:convert';
import 'dart:io';
import 'package:http/http.dart' as http;
The final token
variable is set to the value of the SPOTIFY_TOKEN
environment variable. This variable is set from the Command Line Interface (CLI) when running the application. Your specialized token can be obtained from your spotify api account. developer.spotify.com
final token = Platform.environment['SPOTIFY_TOKEN']!;
The fetchWebApi
function is defined as an asynchronous function that accepts an endpoint
, a method
, and an optional body
. This function sends an HTTP request to the Spotify Web API with the appropriate headers and body.
Future<Map<String, dynamic>> fetchWebApi(String endpoint, String method,
[Map<String, dynamic>? body]) async {
try {
final response = await http.post(
Uri.parse('https://api.spotify.com/$endpoint'),
headers: {'Authorization': 'Bearer $token'},
body: jsonEncode(body ?? {}),
);
if (response.statusCode == 200) {
return jsonDecode(response.body);
} else {
throw Exception('Failed to fetch data from API');
}
} catch (e) {
throw Exception('Failed to connect to API server');
}
}
The getTopTracks
function is defined as an asynchronous function that calls the fetchWebApi
function with the appropriate endpoint and method. The function retrieves the user's top five tracks in the short term and returns them as a list.
Future<List<dynamic>> getTopTracks() async {
try {
final response = await fetchWebApi(
'v1/me/top/tracks?time_range=short_term&limit=5', 'GET');
return response['items'];
} catch (e) {
print(e);
return [];
}
}
The main
function is defined as an asynchronous function that calls the getTopTracks
function and prints the track names and artists to the console.
void main() async {
final topTracks = await getTopTracks();
if (topTracks.isEmpty) {
print('Failed to get top tracks from API');
} else {
print(topTracks
.map((e) =>
'${e['name']} by ${e['artists'].map((artist) => artist['name']).join(', ')}')
.toList());
}
}
In conclusion, this Dart code retrieves the top five tracks from a user's account using the Spotify Web API. The code is designed to be used in conjunction with a valid authorization token from the Spotify Web API. The code uses asynchronous functions to retrieve and display the user's top tracks.
There we have it!!
Subscribe to my newsletter
Read articles from Oluwaseyi Ogunjinmi directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by
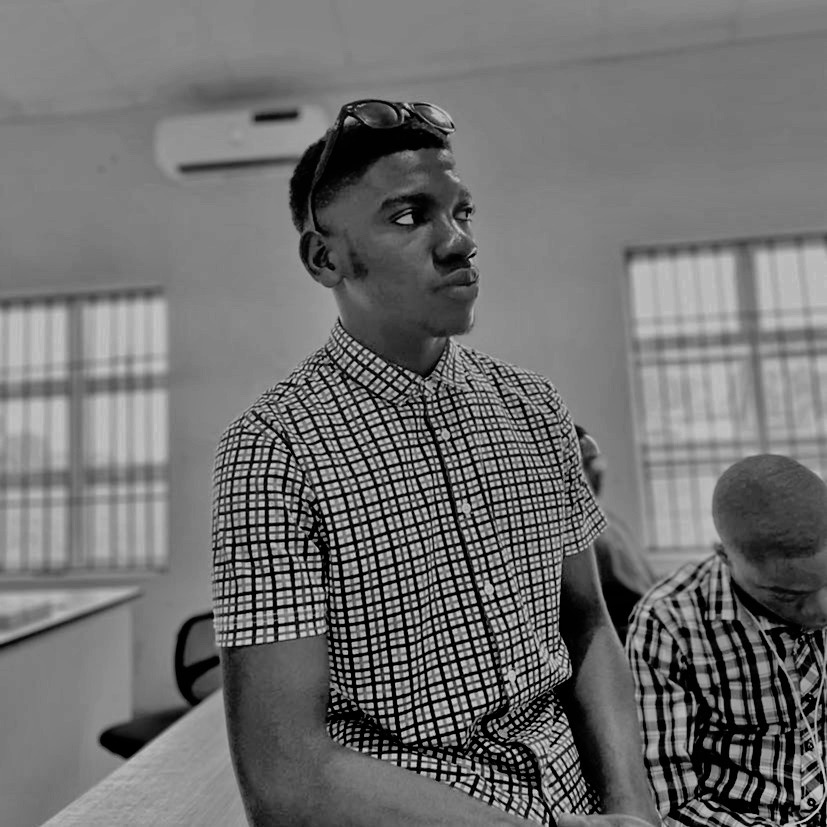
Oluwaseyi Ogunjinmi
Oluwaseyi Ogunjinmi
A skilled software engineer with expertise in Golang, Kubernetes, and backend systems , autonomous machine development. Focused on building scalable solutions and enhancing deployment efficiency. A solid interest in biology and ecosystems. A skilled technical writer.