How to Integrate Stripe API in Nextjs
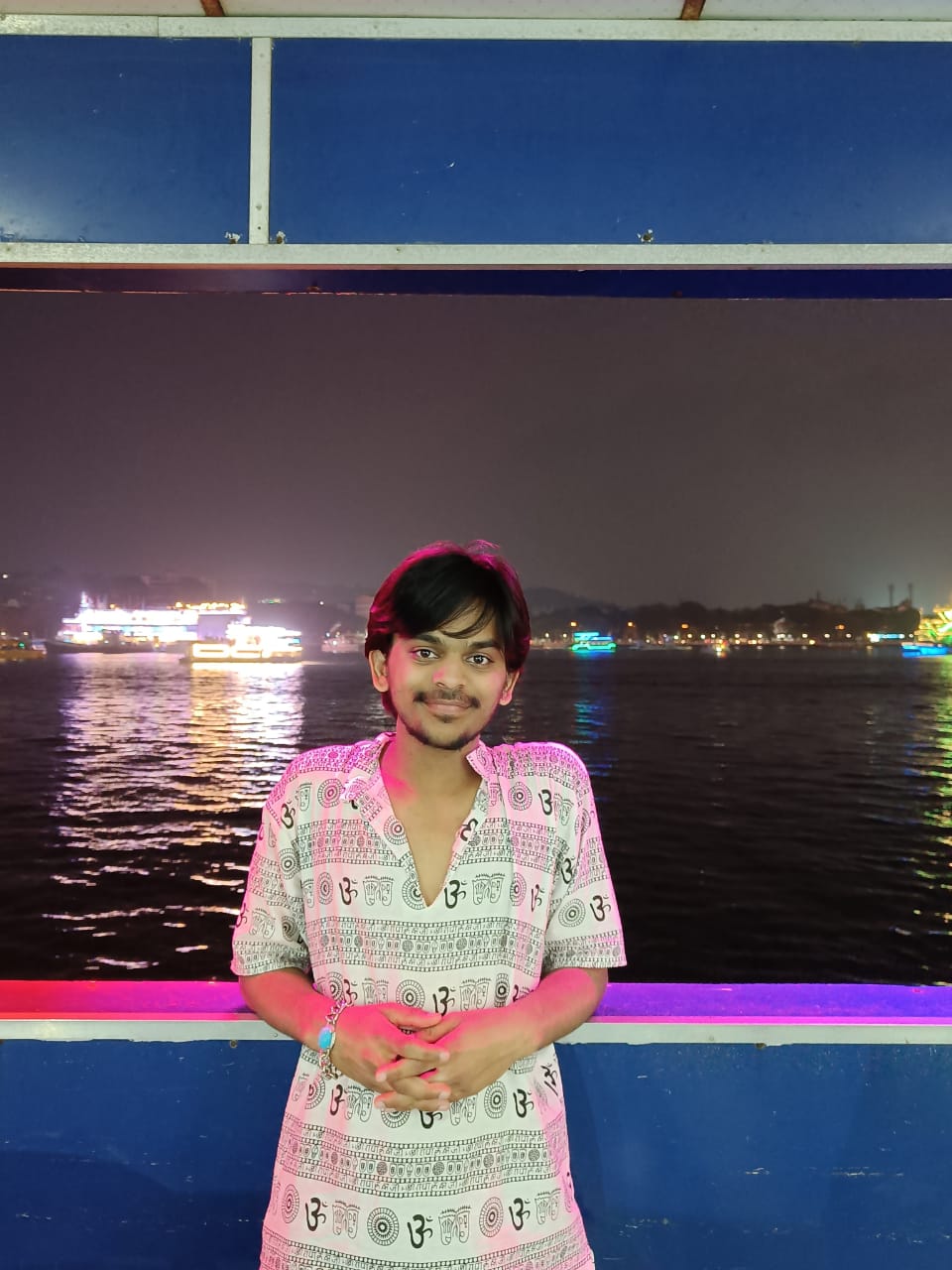
working with Stripe was so hectic task for me which I recently did on my client project. today In this blog I am going to write that experience and how did I do that as well.
first, you have to set up the stripe account and build your products manually there is no code for this(I will not write about it coz, I didn't do that)
Next, you would be required to fetch products from Stripe API.
I tried getStaticProp in nextjs for this.
export async function getStaticProps() {
const stripe = new Stripe(process.env.NEXT_PUBLIC_STRIPE_SECRET_KEY!, {
apiVersion: '2022-11-15',
});
const { data: prices } = await stripe.prices.list({
active: true,
expand: ['data.product'],
});
return {
props: {
prices,
},
};
}
once, you got products you can store them in a variable and use it to develop our product table UI.
Next, you would need to make a POST Request to Stripe API to navigate to the checkout page.
the code for the checkout page would be straightforward.
here is the API we are calling,
import { allowed_countries } from '@/utils/constants';
import Stripe from 'stripe';
const stripe = new Stripe(process.env.NEXT_PUBLIC_STRIPE_SECRET_KEY!, {
apiVersion: '2022-11-15',
});
const handler = async (req: any, res: any) => {
if (req.method === 'POST') {
try {
const { lineItems, email } = req.body;
if (!lineItems.length) {
return res.status(400).json({ error: 'Bad Request!' });
}
const session = await stripe.checkout.sessions.create({
line_items: lineItems,
mode: 'payment',
success_url: `${req.headers.origin}/checkout/success?sessionId={CHECKOUT_SESSION_ID}`,
cancel_url: req.headers.origin,
customer_email: email,
phone_number_collection: {
enabled: true,
},
billing_address_collection: 'auto',
shipping_address_collection: {
allowed_countries: allowed_countries as any
},
"payment_method_types": [
"card"
],
client_reference_id: 'my_id', // uid
consent_collection: {
terms_of_service: 'required',
},
allow_promotion_codes: true,
});
return res.status(201).json({ session });
} catch (e: any) {
return res.status(e.statusCode || 500).json({ message: e.message });
}
}
res.setHeader('Allow', 'POST');
res.status(405).end('Method Not Allowed');
};
export default handler;
this will allow your user to make payment successfully and then navigate to the success page where you can run further queries.
here is how you are going to fetch details of current transaction, you can call this on success page to store it in database.
import Stripe from 'stripe';
const stripe = new Stripe(process.env.NEXT_PUBLIC_STRIPE_SECRET_KEY!, {
apiVersion: '2022-11-15',
});
const handler = async (req: any, res: any) => {
if (req.method === 'GET') {
try {
const { sessionId } = req.query;
if (!sessionId.startsWith('cs_')) {
throw Error('Incorrect Checkout Session ID.');
}
const checkoutSession = await stripe.checkout.sessions.retrieve(
sessionId,
{
expand: ['payment_intent', 'line_items.data.price.product'],
},
);
return res.status(200).json(checkoutSession);
} catch (err) {
const errorMessage =
err instanceof Error ? err.message : 'Internal Server Error';
return res.status(500).json({ statusCode: 500, message: errorMessage });
}
}
res.setHeader('Allow', 'POST');
res.status(405).end('Method Not Allowed');
};
export default handler;
Subscribe to my newsletter
Read articles from Abhi Jain directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by
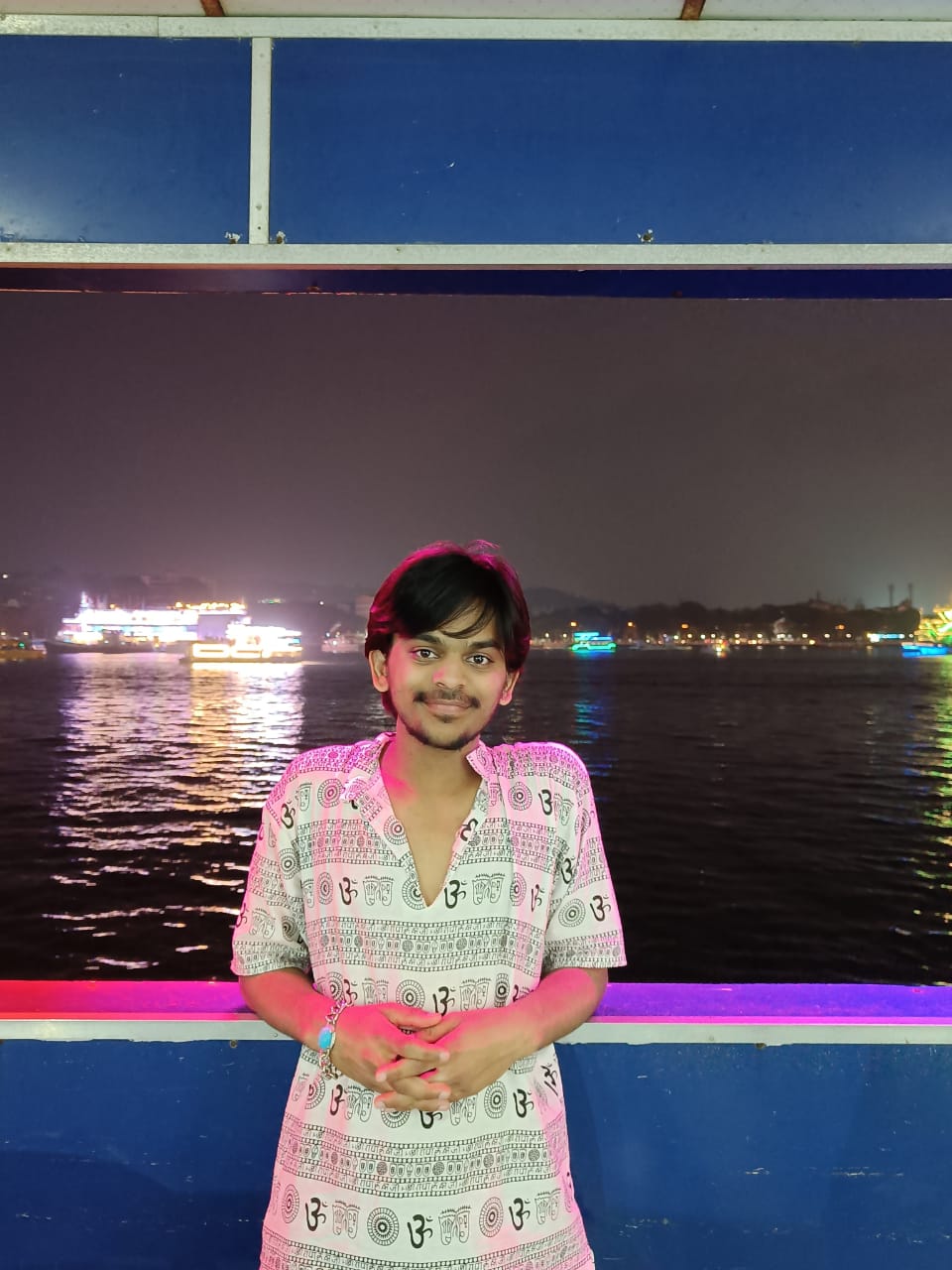
Abhi Jain
Abhi Jain
I am a full-stack web and app developer from India. Love building and collaborating software which solves problem.