How FinTech Companies Handle Currency Calculations: Best Practices in Python
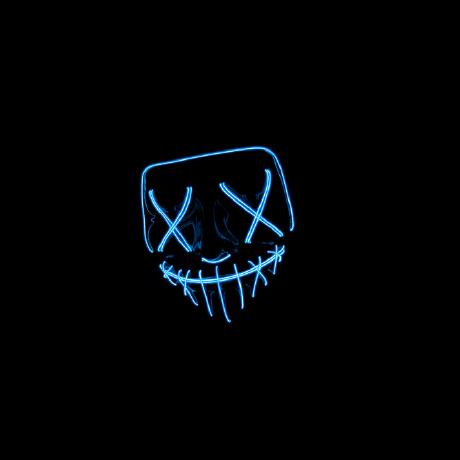
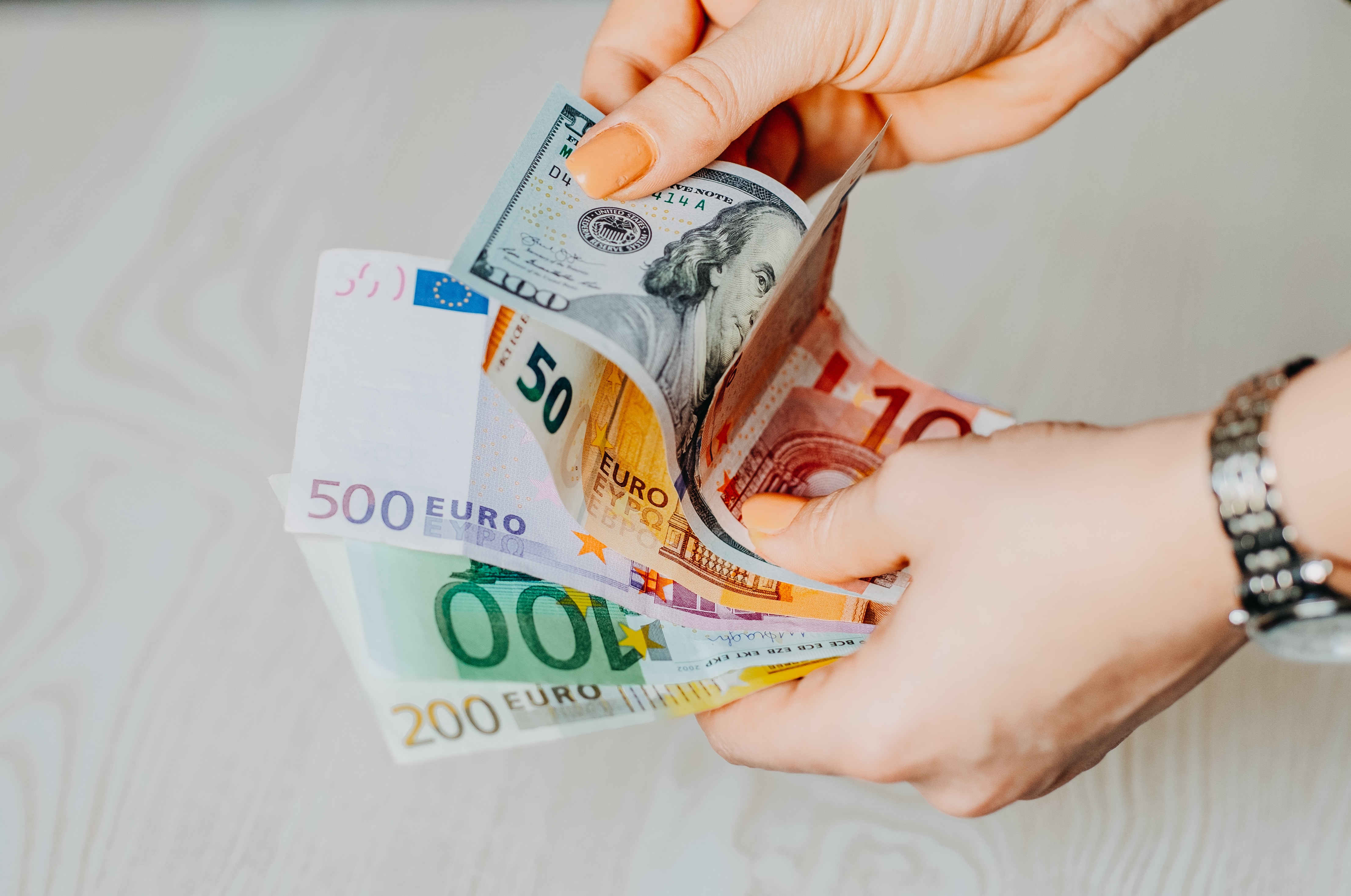
As the world becomes increasingly interconnected and globalized, the importance of accurate and reliable currency calculations cannot be overstated. For fintech companies in particular, who rely heavily on financial transactions, handling currency calculations with precision and efficiency is a critical aspect of their operations.
In this blog post, we'll explore the best practices for currency calculations that help fintech companies ensure accurate and consistent results in their financial transactions. By the end of this post, you'll have a better understanding of how fintech companies handle currency calculations and the tools and techniques available in Python to help you do the same.
Always use the smallest unit of the currency
That's it, we are done. see you next time :)
...
Okay, if you are still here, let's dive deeper.
Floating-Point Precision
As a developer, you may have come across issues with floating-point precision when working with currency values in your code. These issues can lead to unexpected results in your calculations, making it challenging to ensure the accuracy and reliability of your application.
One approach to avoid these issues is to work with values in the smallest unit of the currency, such as the Cent for Dollar or Kobo for Naira. By doing so, you can perform calculations using integers, which are not subject to the same precision issues as floating-point numbers.
Here's an example that illustrates this point:
# Calculation with Naira values (using floating-point numbers)
value_1 = 0.1 # Naira
value_2 = 0.2 # Naira
result = value_1 + value_2
# Conversion to Kobo
result_in_kobo = result * 100 # 1 Naira = 100 Kobo
# Output
print(result_in_kobo) # Output: 30.0 (expected output: 30)
In this example, the calculation involves adding two Naira values represented as floating-point numbers. However, due to the limitations of floating-point precision, the result is not exactly 0.3 as expected, but rather a close approximation. This approximation can lead to errors in subsequent calculations or when converting the result to Kobo.
Working with values in the smallest unit of the currency, such as Kobo, can help avoid these floating-point precision issues, as all calculations can be performed using integers.
Here's an example that uses Kobo values instead:
# Calculation with Kobo values (using integers)
value_1 = 10 # Kobo
value_2 = 20 # Kobo
result = value_1 + value_2
# Output
print(result) # Output: 30
As you can see, working with Kobo values eliminates the floating-point precision issue, and the result is exactly what we expect.
Units Conversion
Another advantage of working with values in the smallest unit of the currency is that it can help simplify your code by eliminating the need for conversions between different currency units. This can make your code more efficient and easier to maintain in the long run.
Here is an example that demonstrates this point:
# Calculation with Naira values
value_1 = 10 # Naira
value_2 = 20 # Naira
result = value_1 + value_2
# Conversion to Kobo
result_in_kobo = result * 100 # 1 Naira = 100 Kobo
# Output
print(result_in_kobo) # Output: 3000
# Calculation with Kobo values
value_1 = 1000 # Kobo
value_2 = 2000 # Kobo
result = value_1 + value_2
# Output
print(result) # Output: 3000
In this example, we perform the same calculation twice, once with Naira values and then with Kobo values. When working with Naira values, we need to convert the result to Kobo, which requires an additional calculation. However, when working with Kobo values, we can perform the calculation directly without the need for conversion, making our code more efficient and easier to understand.
In conclusion, utilizing the smallest unit of a currency is a fundamental best practice for enhancing the precision, dependability, and effectiveness of your application when processing currency values. This approach helps you avoid the costly mistake of incorrectly crediting or debiting values in your customer's account. We hope this article has provided valuable insights into the significance of this practice and how it can be applied to the development/optimization of your fintech products.
Thank you for reading!
Subscribe to my newsletter
Read articles from Uchechukwu Emmanuel directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by
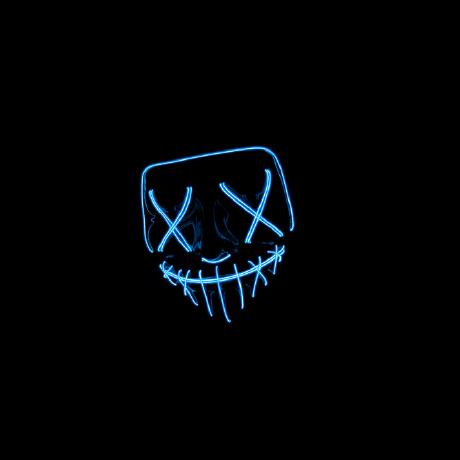