Shell basics handbook
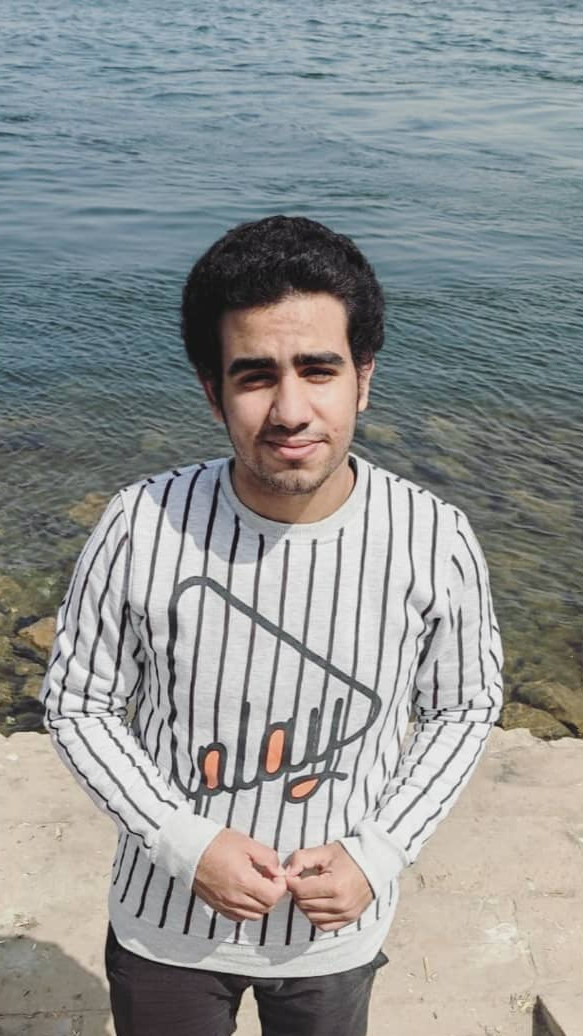
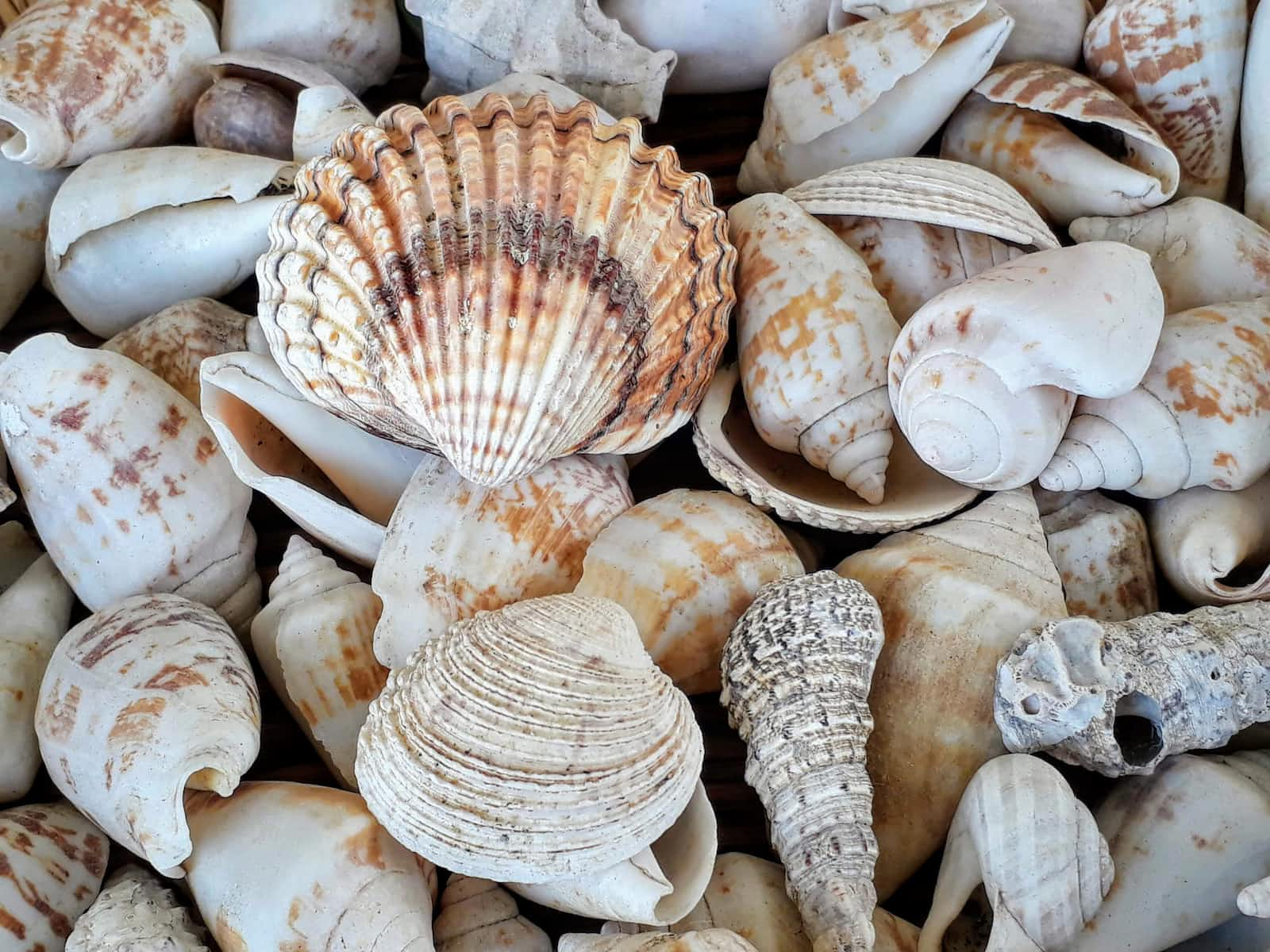
Buy me a coffee ☕ to help me keep going, thanks in advance! ❤
A shell is a program that acts as an interface between a user and the kernel. It allows a user to give commands to the kernel and receive responses from it. Through a shell, we can execute programs and utilities on the kernel. Hence, at its core, a shell is a program used to execute other programs on our system. It is responsible for the control management, and execution of processes, and ensuring proper utilization of system resources.
Being able to interact with the kernel makes shells a powerful tool. Without the ability to interact with the kernel, a user cannot access the utilities offered by their machine’s operating system.
What's a "Terminal?"
It's a program called a terminal emulator. This is a program that opens a window and lets you interact with the shell. There are a bunch of different terminal emulators we can use. Some Linux distributions install several.
These might include gnome-terminal
, konsole
, xterm
, rxvt
, kvt
, nxterm
, and eterm
.
In Linux, you can navigate your filesystem using the shell prompt. Navigation is based on the concept of paths. These paths specify what directories to traverse to reach a particular subdirectory or file. There are two types of paths: Absolute paths and relative paths.
In the Linux shell, the $
and #
Symbols have different meanings. The $
symbol is used to indicate that you are logged in as a regular user. The #
symbol is used to indicate that you are logged in as the root user.
In Linux, the root user is the most powerful user on a Unix/Linux system.
File names in Linux, like Unix, are case-sensitive. "File1" and "file1" refer to different files.
Most importantly, do not embed spaces in file names. If you want to represent spaces between words in a file name, use underscore characters. You will thank yourself later.
Working with Commands
we’ll see several commands and their mysterious options and arguments. We will try to remove some of that mystery using the following commands.
Most commands operate like this:
command -options arguments
What are "Commands?"
Commands can be one of 4 different kinds:
An executable program like all those files we saw in
/usr/bin
. Within this category, programs can be compiled binaries such as programs written in C and C++, or programs written in scripting languages such as the shell, Perl, Python, Ruby, etc.A command is built into the shell itself. bash provides several commands internally called shell built-ins. The
cd
command, for example, is a shell built-in.A shell function. These are miniature shell scripts incorporated into the environment. We will cover configuring the environment and writing shell functions in later lessons, but for now, just be aware that they exist.
An alias. Commands that we can define ourselves, are built from other commands. This will be covered in a later lesson.
type
(displays the kind of command the shell will execute)
It is often useful to know exactly which of the four kinds of commands is being used.
[me@linuxbox me]$ type cp
which
(determines the exact location of a given executable)
Sometimes there is more than one version of an executable program installed on a system. While this is not very common on desktop systems, it's not unusual on large servers.
[me@linuxbox me]$ which ls
which
only works for executable programs, not built-ins nor aliases that are substitutes for actual executable programs.
Getting Command Documentation
With this knowledge of what a command is, we can now search for the documentation available for each kind of command.
help
(a built-in facility available for each of the shell built-ins)
we can add the -m
option to change the format of the output.
[me@linuxbox me]$ help -m cd
A note on notation: When square brackets appear in the description of a command's syntax, they indicate optional items. A vertical bar character indicates mutually exclusive items. In the case of the cd command above:
cd [-L|-P] [dir]
--help
(an option that displays a description of the command)
Many executable programs support a --help
option. For example:
[me@linuxbox me]$ mkdir --help
man
(an interface to the system reference manuals)
Most executable programs intended for command line use provide a formal piece of documentation called a manual or man page. A special paging program called man
is used to viewing them.
man command
On most Linux systems,
man
usesless
to display the manual page, so all of the familiarless
commands work while displaying the page.Man pages vary somewhat in format but generally contain a title, a synopsis of the command's syntax, a description of the command's purpose, and a listing and description of each of the command's options. Man pages, however, do not usually include examples, and are intended as a reference, not a tutorial.
README and Other Documentation Files
Many software packages installed on your system have documentation files residing in the /usr/share/doc
directory. Most of these are stored in plain text format and can be viewed with less
. Some of the files are in HTML format and can be viewed with a web browser.
Keyboard shortcuts for Bash
The bash shell features a wide variety of keyboard shortcuts you can use. These will work in bash on any operating system. Some of them may not work if you’re accessing bash remotely through an SSH or telnet session, depending on how you have your keys mapped.
Working With Processes
Ctrl+C
: Interrupt (kill) the current foreground process running-in in the terminal. This sends theSIGINT
signal to the process, which is technically just a request—most processes will honour it, but some may ignore it.Ctrl+Z
: Suspend the current foreground process running in bash. This sends the SIGTSTP signal to the process. To return the process to the foreground later, use thefg process_name
command.Ctrl+D
: Close the bash shell. This sends an EOF (End-of-file) marker to bash, and bash exits when it receives this marker. This is similar to running theexit
command.
Controlling the Screen
Ctrl+L
: Clear the screen. This is similar to running theclear
command.Ctrl+S
: Stop all output to the screen. This is particularly useful when running commands with a lot of long, verbose output, but you don’t want to stop the command itself withCtrl+C
.Ctrl+Q
: Resume output to the screen after stopping it withCtrl+S
.
Tab Completion
Tab completion is a very useful bash feature. While typing a file, directory, or command name, press tab
and bash will automatically complete what you’re typing, if possible. If not, bash will show you various possible matches and you can continue typing and pressing tab
to finish typing.
Working With Your Command History
Up Arrow
: Go to the previous command in the command history.Down Arrow
: Go to the next command in the command history.Alt+R
: Revert any changes to a command you’ve pulled from your history if you’ve edited it.Ctrl+R
: Recall the last command matching the characters you provide. Press this shortcut and start typing to search your bash history for a command.Ctrl+O
: Run a command you found withCtrl+R
.Ctrl+G
: Leave history searching mode without running a command.
The Shebang
It is also called sharp-exclamation
, sha-bang
, hashbang
, pound-bang
, or hash-pling
.
The shebang line is a character sequence consisting of the characters' number sign and exclamation mark #!
at the beginning of a script. When a text file with a shebang is used as if it is executable in a Unix-like operating system, the program loader mechanism parses the rest of the file’s initial line as an interpreter directive. The loader executes the specified interpreter program, passing to it as an argument the path that was initially used when attempting to run the script, so that the program may use the file as input data.
The shebang line is usually ignored by the interpreter because the “#” character is a comment marker in many scripting languages; some language interpreters that do not use the hash mark to begin comments still may ignore the shebang line in recognition of its purpose.
#!/interpreter/[optional-arg]
Examples:
#!/bin/sh
– Execute the file using the Bourne shell, or a compatible shell, assumed to be in the/bin
directory#!/bin/bash
– Execute the file using the Bash shell#!/usr/bin/pwsh
– Execute the file using PowerShell#!/usr/bin/env python3
– Execute with a Python interpreter, using theenv
program search path to find it#!/bin/false
– Do nothing, but return a non-zero exit status, indicating failure. Used to prevent stand-alone execution of a script file intended for execution in a specific context, such as by the.
command fromsh/bash
,source
fromcsh/tcsh
, or as a.profile
,.cshrc
, or.login
file.
Looking Around
ls
(list files and directories)
The ls
command is used to list the contents of a directory. It is probably the most commonly used Linux command.
Command | Result |
| List the files in the working directory |
| List the files in the |
| List the files in the working directory in long format |
| List the files in the |
| List all files (even ones with names beginning with a period character, which are normally hidden) in the parent of the working directory in a long format |
A closer look at long format if we use the -l
option with ls
-rw------- 1 me me 576 Apr 17 2019 weather.txt
drwxr-xr-x 6 me me 1024 Oct 9 2019 web_page
-rw-rw-r-- 1 me me 276480 Feb 11 20:41 web_site.tar
-rw------- 1 me me 5743 Dec 16 2018 xmas_file.txt
---------- ------- ------- -------- ------------ -------------
| | | | | |
| | | | | File Name
| | | | |
| | | | +--- Modification Time
| | | |
| | | +------------- Size (in bytes)
| | |
| | +----------------------- Group
| |
| +-------------------------------- Owner
|
+---------------------------------------------- File Permissions
less
(view text files)
less
is a program that lets us view text files. This is very handy since many of the files used to control and configure Linux are human-readable (I prefer to use cat
command).
less text_file
What is "text"?
To represent information on a computer, a relationship is defined between the information and some numbers, as computers only understand numbers. All data is converted to numeric representation.
Representation systems vary in complexity, with some being highly intricate (e.g. compressed multimedia files) and others being quite simple. One of the earliest, simplest systems is called ASCII text, which stands for American Standard Code for Information Interchange. It's an encoding scheme that maps keyboard characters to numbers and was first used on Teletype machines.
Text is a compact mapping of characters to numbers. Linux systems store many files in text format, and numerous Linux tools work with text files. Even Windows systems recognize the importance of this format; NOTEPAD.EXE is an editor for plain ASCII text files.
Once started, less
will display the text file one page at a time. Here are some commands that less
will accept:
Command | Action |
Page Up or b | Scroll back one page |
Page Down or space | Scroll forward one page |
G | Go to the end of the text file |
1G | Go to the beginning of the text file |
/characters | Search forward in the text file for an occurrence of the specified characters |
n | Repeat the previous search |
h | Display a complete list |
q | Quit |
file
(classify a file's contents)
To efficiently view files in Linux, we can use the file
command to determine their type.
file name_of_file
Its output looks something like this:
index.html: HTML document, ASCII text
---------- -------------------------
| |
File Name File Type
A Guided Tour
The table below lists some interesting places to explore.
Directory | Description |
| The root directory where the file system begins. The root directory will probably contain only subdirectories. |
| This is where the Linux kernel and boot loader files are kept. The kernel is a file called |
| The |
| These two directories contain most of the programs for the system. The |
| The |
| The |
|
|
| The |
| The shared libraries (similar to DLLs in that other operating system) are kept here. |
|
|
| This is the superuser's home directory. |
|
|
| The |
| The |
| Finally, we come to |
A weird kind of file...
During your tour, you probably noticed a strange kind of directory entry, particularly in the /lib directory. When listed with ls -l, you might have seen something like this:
lrwxrwxrwx 25 Jul 3 16:42 System.map -> /boot/System.map-4.0.36-3 -rw-r--r-- 105911 Oct 13 2018 System.map-4.0.36-0.7 -rw-r--r-- 105935 Dec 29 2018 System.map-4.0.36-3 -rw-r--r-- 181986 Dec 11 2019 initrd-4.0.36-0.7.img -rw-r--r-- 182001 Dec 11 2019 initrd-4.0.36.img lrwxrwxrwx 26 Jul 3 16:42 module-info -> /boot/module-info-4.0.36-3 -rw-r--r-- 11773 Oct 13 2018 module-info-4.0.36-0.7 -rw-r--r-- 11773 Dec 29 2018 module-info-4.0.36-3 lrwxrwxrwx 16 Dec 11 2019 vmlinuz -> vmlinuz-4.0.36-3 -rw-r--r-- 454325 Oct 13 2018 vmlinuz-4.0.36-0.7 -rw-r--r-- 454434 Dec 29 2018 vmlinuz-4.0.36-3
Notice the files,
system.map
, module-info
andvmlinuz
. See the strange notation after the file names?Files such as this are called symbolic links. Symbolic links are a special type of file that points to another file. With symbolic links, it is possible for a single file to have multiple names. Here's how it works: Whenever the system is given a file name that is a symbolic link, it transparently maps it to the file it is pointing to.
Just what is this good for? This is a very handy feature. Let's consider the directory listing above (which is the
/boot
directory of an old system). This system has had multiple versions of the Linux kernel installed. We can see this from the filesvmlinuz-4.0.36-0.7
andvmlinuz-4.0.36-3
. These file names suggest that both version 4.0.36-0.7 and 4.0.36-3 are installed. Because the file names contain the version it is easy to see the differences in the directory listing. However, this would be confusing to programs that rely on a fixed name for the kernel file. These programs might expect the kernel to simply be calledvmlinuz
. Here is where the beauty of the symbolic link comes in. By creating a symbolic link calledvmlinuz
that points tovmlinuz-4.0.36-3
, we have solved the problem.To create symbolic links, we use the
ln
command.
Manipulating Files
Now, to be frank, some of the tasks performed by these commands are more easily done with a graphical file manager. With a file manager, you can drag and drop a file from one directory to another, cut and paste files, delete files, etc. So why use these old command line programs?
Then, how would you copy all the HTML files from one directory to another, but only copy files that did not exist in the destination directory or were newer than the versions in the destination directory? Pretty hard with a file manager. Pretty easy with the command line:
[me@linuxbox me]$ cp -u *.html destination
Wildcards
Before we start using commands, let's learn about a shell feature that makes them powerful - wildcards. These special characters allow you to select filenames based on patterns of characters. See the table below for the list of wildcards and what they select:
Wildcard | Meaning |
| Matches any characters |
| Matches any single character |
| Matches any character that is a member of the set characters. The set of characters may also be expressed as a POSIX character class such as one of the following: |
| Matches any character that is not a member of the set characters |
Here are some examples of patterns and what they match:
Pattern | Matches |
All filenames | |
| All filenames that begin with the character "g" |
| All filenames that begin with the character "b" and end with the characters ".txt" |
| Any filename that begins with the characters "Data" followed by exactly 3 more characters |
| Any filename that begins with "a" or "b" or "c" followed by any other characters |
| Any filename that begins with an uppercase letter. This is an example of a character class. |
| Another example of character classes. This pattern matches any filename that begins with the characters "BACKUP." followed by exactly two numerals. |
| Any filename that does not end with a lowercase letter. |
We can use wildcards with any command that accepts filename arguments.
A note on notation:
...
signifies that an item can be repeated one or more times.
These four commands are among the most frequently used Linux commands. They are the basic commands for manipulating both files and directories:
cp
(copy files and directories)
The cp
program copies files and directories.
[me@linuxbox me]$ cp file1 file2
Other useful examples of cp
and its options include:
Command | Results |
| Copies the contents of file1 into file2. If file2 does not exist, it is created; otherwise, file2 is silently overwritten with the contents of file1. |
| Like above however, since the |
| Copy the contents of file1 (into a file named file1) inside of directory dir1. |
| Copy the contents of the directory dir1. If directory dir2 does not exist, it is created. Otherwise, it creates a directory named dir1 within directory dir2. |
mv
(move or rename files and directories)
The mv
command moves or renames files and directories depending on how it is used. It will either move one or more files to a different directory, or it will rename a file or directory. To rename a file.
[me@linuxbox me]$ mv filename1 filename2
Examples of mv
and its options include:
Command | Results |
| If file2 does not exist, then file1 is renamed file2. If file2 exists, its contents are silently replaced with the contents of file1. |
| Like above however, since the |
| The files file1 and file2 are moved to directory dir1. If dir1 does not exist, |
| If dir2 does not exist, then dir1 is renamed dir2. If dir2 exists, the directory dir1 is moved within directory dir2. |
rm
(remove files and directories)
The rm
command removes (deletes) files and directories.
[me@linuxbox me]$ rm file...
Using the recursive option -r
, rm
can also be used to delete directories:
[me@linuxbox me]$ rm -r directory...
Examples of rm
and its options include:
Command | Results |
| Delete file1 and file2. |
| Like above however, since the |
| Directories dir1 and dir2 are deleted along with all of their contents. |
rm
IS DANGROUS!Linux does not have an undelete command. Once you delete something with
rm
, it's gone. You can inflict terrific damage on your system withrm
if you are not careful, particularly with wildcards.Try construct your command using
ls
instead. By doing this, you can see the effect of your wildcards before you delete files.
mkdir
(create directories)
The mkdir
command is used to create directories.
[me@linuxbox me]$ mkdir directory...
Since the commands we have covered here accept multiple file and directories names as arguments, you can use wildcards to specify them. Here are a few examples:
Command | Results |
| Copy all files in the current working directory with names ending with the characters ".txt" to an existing directory named text_files. |
| Move the subdirectory dir1 and all the files ending in |
| Delete all files in the current working directory that end with the character "~". Some applications create backup files using this naming scheme. Using this command will clean them out of a directory. |
Buy me a coffee ☕ to help me keep going, thanks in advance! ❤
Resources
Subscribe to my newsletter
Read articles from Youssef Hassan directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by
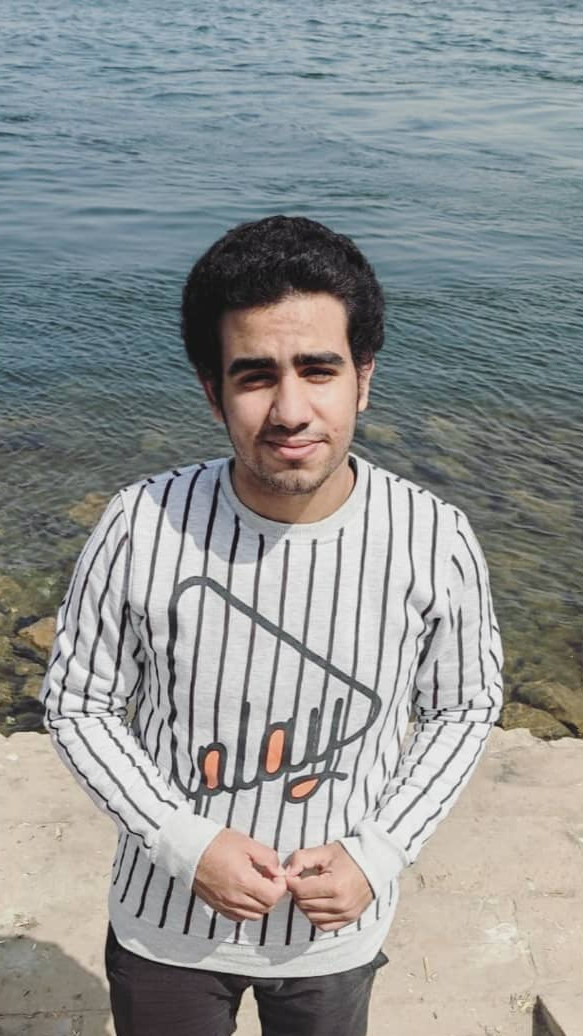