Finding a string from the string collection

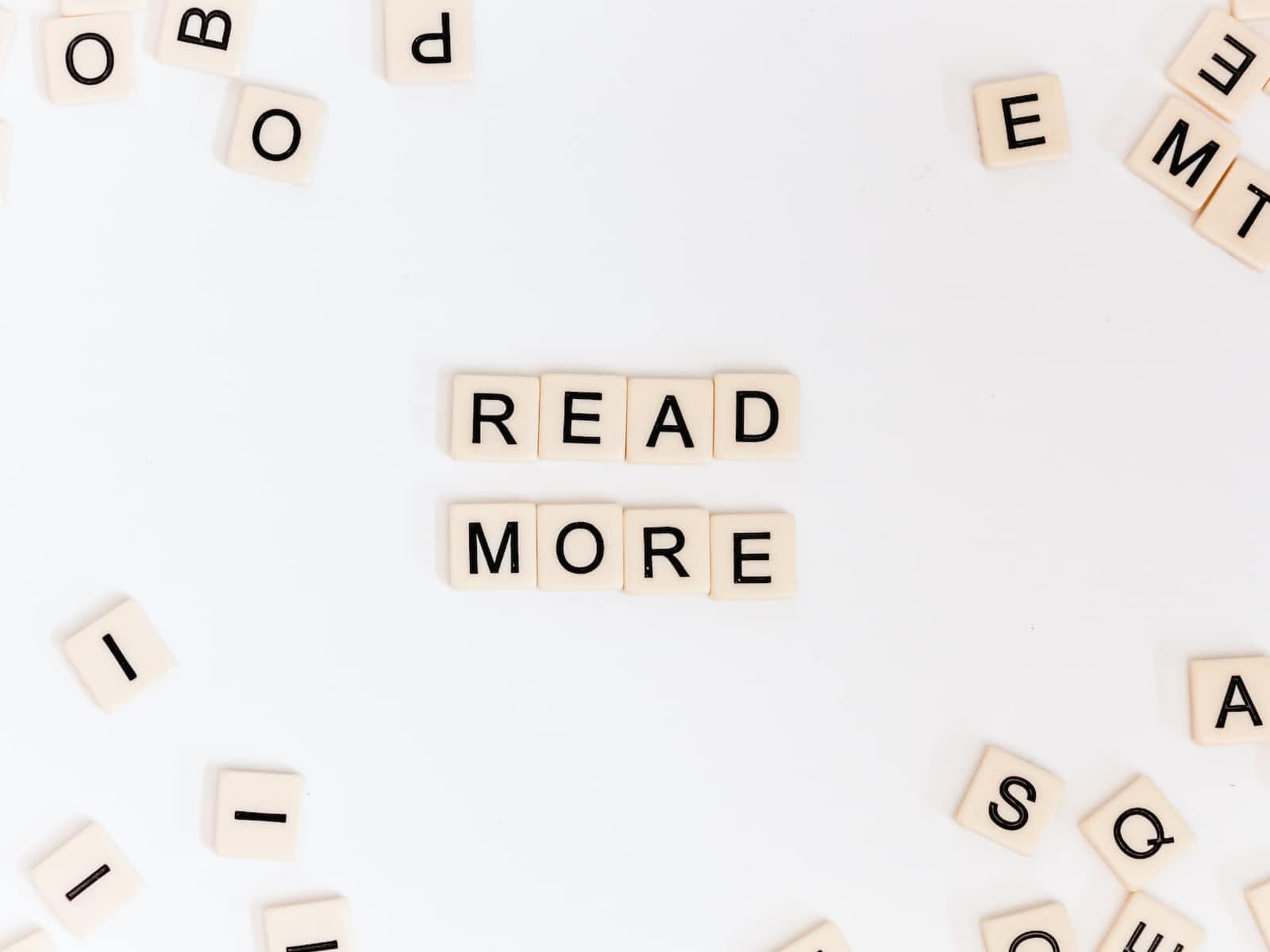
As Uncle Ben once said, "With great power comes great responsibility"
The same is true with the Contains feature; we need it in a variety of situations to accomplish a variety of tasks, but we often run into problems. To better understand this, let's look at an example.
var myString = "AmIThere";
var container = "AmIThereIsThere";
var container1 = "AmIthereIsThere";
if(container.Contains(myString))
Console.WriteLine("My String is available in container");
else
Console.WriteLine("My String is not available in container");
if (container1.Contains(myString))
Console.WriteLine("My String is available in container1");
else
Console.WriteLine("My String is not available in container1");
/*
Output:
My String is available in container
My String is not available in container1
*/
Now one question might come, in both cases, we were comparing the same string but how come it failed in the second check, the reason is
Contains method is Case-sensitive
In the real world, we might face a situation wherein we need to check if a string contains our expected string and not know the case of an incoming string.
What's the alternative we have?
Below are the options we can use to solve our case-sensitive issue from Contains method
// Option1: Using Regex
if (Regex.IsMatch(container1, Regex.Escape(myString), RegexOptions.IgnoreCase))
Console.WriteLine("My String is available in container1");
else
Console.WriteLine("My String is not available in container1");
// Option2: Using IndexOf
if (container1.IndexOf(myString, StringComparison.OrdinalIgnoreCase) != -1) //If string matches then IndexOf would return index of first occurence else -1
Console.WriteLine("My String is available in container1");
else
Console.WriteLine("My String is not available in container1");
//Option3: Using the overloaded Contains method, available only with newer versions of .Net
if (container1.Contains(myString, StringComparison.CurrentCultureIgnoreCase))
Console.WriteLine("My String is available in container");
else
Console.WriteLine("My String is not available in container");
Hope you found this post useful :)
Subscribe to my newsletter
Read articles from Mukesh Pareek directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by
