Functions in Dart

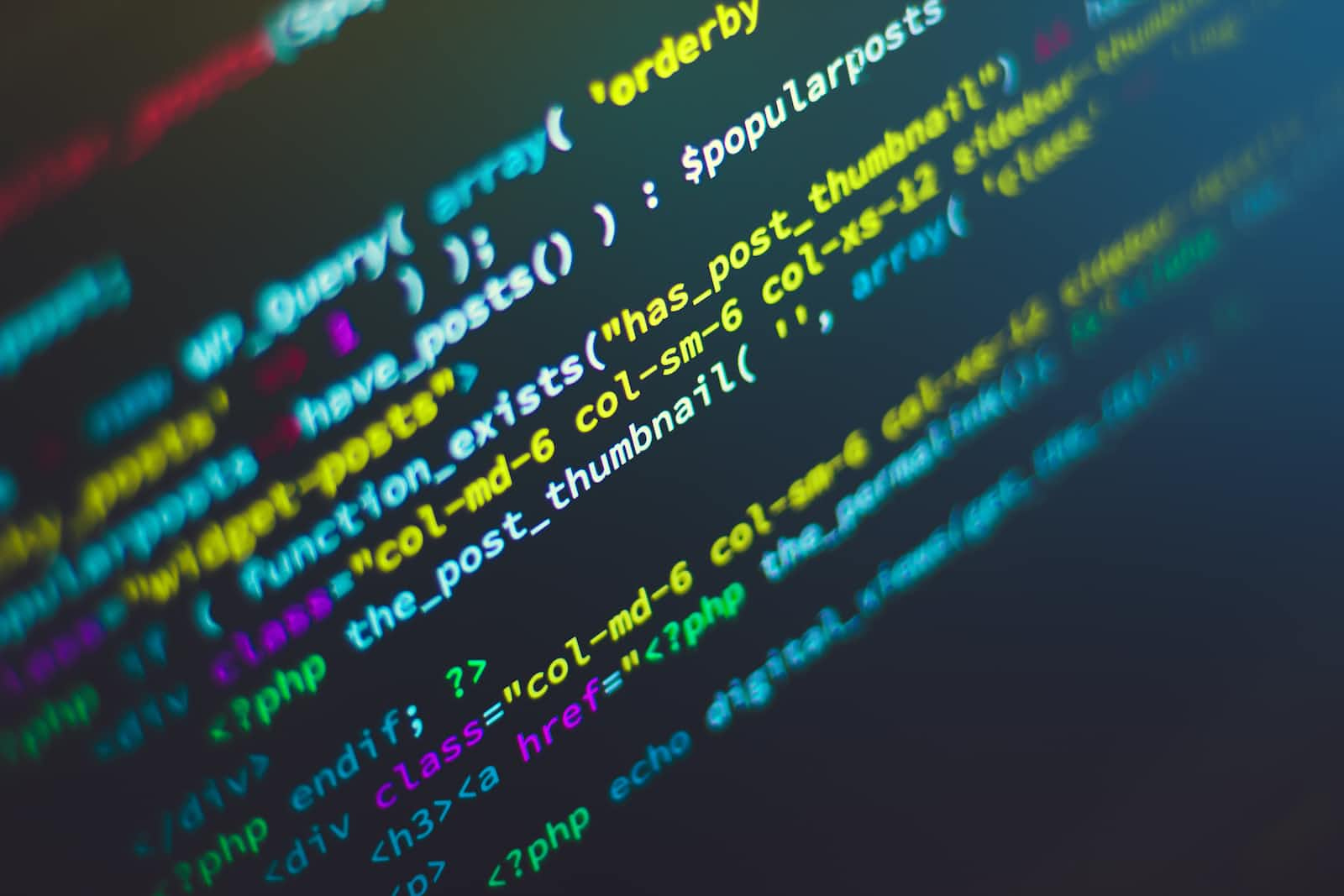
Dart is a modern object-oriented programming language designed to create scalable, robust, and efficient mobile and web applications. One of the essential components of Dart programming is functions, which are self-contained blocks of code that perform a specific task. In this blog, we'll explore the basics of Dart functions and how they work.
Defining a Function:
A function in Dart is defined using the 'Function' keyword, followed by the function name and a set of parentheses that may or may not contain one or more parameters. Here is a simple example of a Dart function that takes two integers as arguments and returns their sum:
int sum(int x, int y) {
return x + y;
}
In the above example, the function is named 'sum,' and it takes two integers 'x' and 'y' as parameters. The function returns the sum of the two integers by adding them using the '+' operator.
Calling a Function:
Once a function is defined, you can call it by specifying its name and passing the required arguments. Here is an example of how to call the 'sum' function we defined earlier:
void main() {
int result = sum(3, 5);
print(result); // Output: 8
}
In the above example, we call the 'sum' function and pass two integers (3 and 5) as arguments. The function returns the sum of the two integers (8), which we store in a variable named 'result.' We then print the value of the 'result' using the 'print' statement.
Optional Parameters In Dart:
You can define optional parameters for a function by enclosing them in square brackets ('[]') when defining the function. Optional parameters can have default values that are used if no argument is passed for that parameter. Here is an example of a function with optional parameters:
void printDetails(String name, {int age = 18, String country = 'USA'}) {
print('Name: $name');
print('Age: $age');
print('Country: $country');
}
In the above example, we define a function named 'printDetails' that takes a required parameter 'name' and two optional parameters 'age' and 'country.' If no value is provided for the 'age' parameter, it defaults to 18, and if no value is provided for the 'country' parameter, it defaults to 'USA.' We then print out the details passed to the function.
To call this function, we can pass the required parameter 'name' and optionally pass the 'age' and 'country' parameters like this:
void main() {
printDetails('John');
printDetails('Jane', age: 25);
printDetails('Bob', age: 30, country: 'Canada');
}
In the above example, we call the 'printDetails' function three times with different parameters. In the first call, we only pass the required 'name' parameter, and the optional parameters take their default values. In the second call, we pass the 'name' and 'age' parameters, and the 'country' parameter takes its default value. In the third call, we pass all three parameters.
Anonymous Functions In Dart:
You can also define anonymous functions, also known as lambda functions or closures. Anonymous functions are functions that don't have a name and are defined on the fly. They are often used as arguments to other functions or as the return value of a function. Here is an example of an anonymous function that takes two integers and returns their product:
void main() {
var product = (int x, int y) => x * y;
print(product(3, 5)); // Output
Conclusion:
In conclusion, functions are an essential aspect of programming in Dart, enabling developers to write reusable, modular code. We can define functions with required and optional parameters, and return values, and we can call these functions from within our code to perform specific tasks. Additionally, anonymous functions provide a way to create simple functions without needing to give them a name or define them elsewhere. By mastering the use of functions in Dart, developers can write more efficient, readable, and scalable code.
Happy Coding!
Subscribe to my newsletter
Read articles from Tanisha Jaiswal directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by

Tanisha Jaiswal
Tanisha Jaiswal
Actively looking for internship & Full-Time opportunity in Tech | Software Developer | B.Tech(CSE) | 2023 Batch