Python Syntax

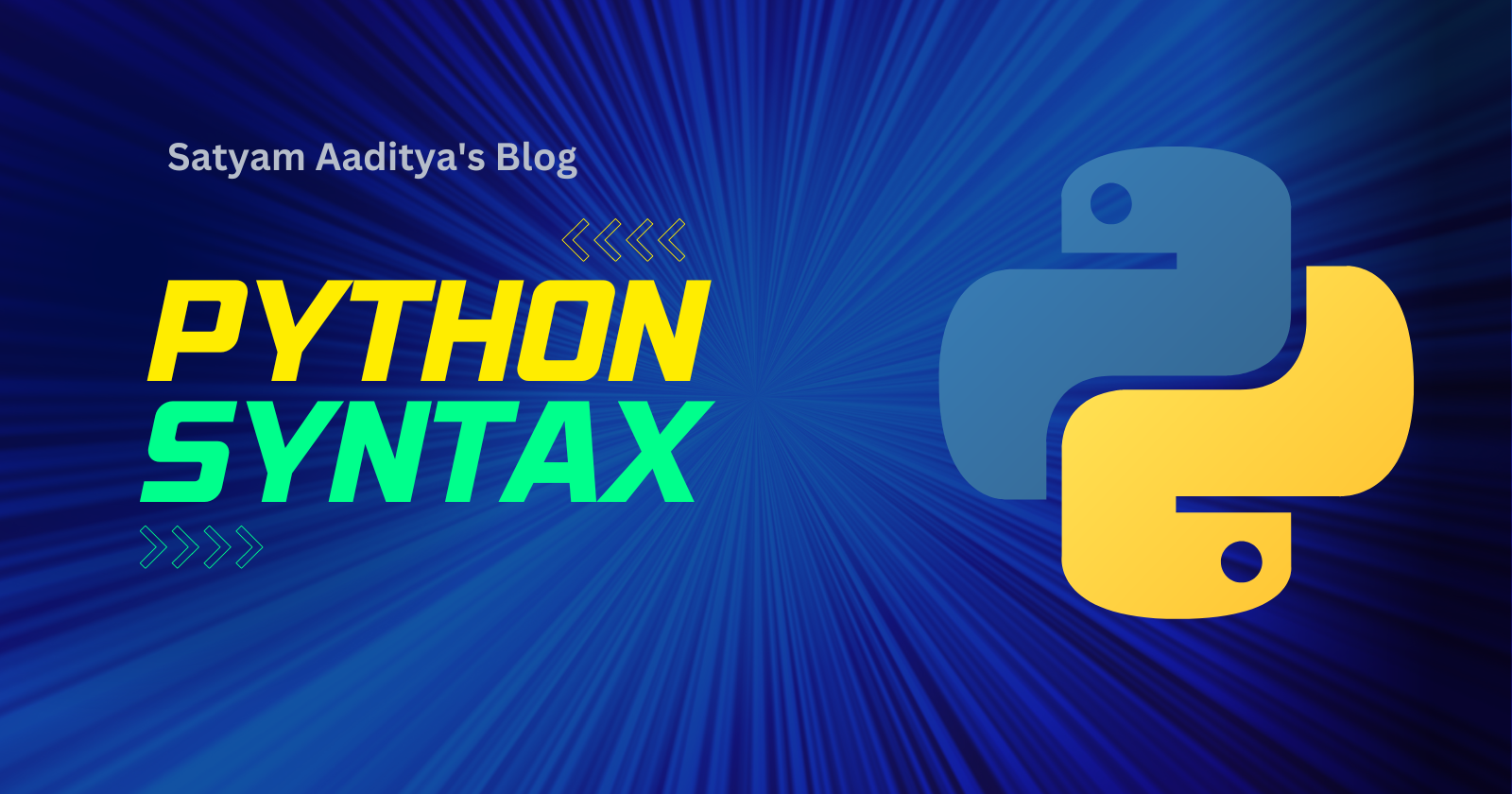
Python is a popular high-level programming language that is known for its simplicity and ease of use. It is a versatile language that can be used for various purposes such as web development, scientific computing, data analysis, artificial intelligence, and machine learning. Python is also an interpreted language, which means that it is executed line by line rather than compiled before execution. In this article, we will discuss Python syntax, which is the set of rules that define how code is written in the Python language.
1. Comments
Comments are lines of code that are not executed by the interpreter. They are used to provide information or explanations about the code. In Python, single-line comments start with the "#" symbol, while multi-line comments are enclosed in triple quotes (""").
Example:
# This is a single-line comment
"""
This is a
multi-line comment
"""
2. Variables
Variables are used to store values in memory. In Python, variables do not need to be explicitly declared. A variable is created when a value is assigned to it. Variable names can only contain letters, numbers, and underscores (_), and they cannot start with a number.
Example:
x = 5
y = "Hello, World!"
z = 3.14
3. Data Types
Python has several built-in data types, including integers, floats, strings, booleans, lists, tuples, and dictionaries.
Example:
# Integer
x = 5
# Float
y = 3.14
# String
z = "Hello, World!"
# Boolean
a = True
# List
b = [1, 2, 3]
# Tuple
c = (4, 5, 6)
# Dictionary
d = {"name": "John", "age": 30}
4. Operators
Operators are used to performing operations on variables and values. Python supports various operators, such as arithmetic operators (+, -, *, /), assignment operators (=, +=, -=), comparison operators (==, !=, <, >), logical operators (and, or, not), and more.
Example:
x = 5
y = 3
# Arithmetic operators
print(x + y) # 8
print(x - y) # 2
print(x * y) # 15
print(x / y) # 1.6666666666666667
# Comparison operators
print(x == y) # False
print(x != y) # True
print(x > y) # True
print(x < y) # False
# Logical operators
print(x > 2 and y < 4) # True
print(x < 2 or y > 4) # False
print(not(x > 2 and y < 4)) # False
5. Conditional Statements
Conditional statements are used to execute different blocks of code based on a certain condition. Python supports if-else statements and nested if statements.
Example:
x = 5
if x > 3:
print("x is greater than 3")
else:
print("x is not greater than 3")
if x > 2:
if x < 6:
print("x is between 2 and 6")
6. Loops
Loops are used to execute a block of code repeatedly. Python supports two types of loops: for loops and while loops.
Example:
# For loop
fruits = ["apple", "banana", "cherry"]
for fruit in fruits:
print(fruit)
# While loop
i = 1
while i < 6:
print(i)
i += 1
7. Functions
Functions are used to group a set of statements that perform a specific task. In Python, functions are defined using the "def" keyword, followed by the function name and parameters in parentheses. The code inside the function is indented.
Example:
def greet(name):
print("Hello, " + name)
greet("John")
Output:
Hello, John
8. Classes
Classes are used to create objects that have properties and methods. In Python, classes are defined using the "class" keyword, followed by the class name. The code inside the class is indented.
Example:
class Person:
def init(self, name, age):
self.name = name
self.age = age
def greet(self):
print("Hello, my name is " + self.name)
person1 = Person("John", 30)
person1.greet()
Output:
Hello, my name is John
9. Modules
Modules are used to organize code into separate files for easier management. In Python, a module is a file that contains Python definitions and statements. To use a module in your code, you can import it using the "import" keyword.
Example:
# File: mymodule.py
def greet(name):
print("Hello, " + name)
# File: main.py
import mymodule
mymodule.greet("John")
Output:
Hello, John
In conclusion, Python syntax is the set of rules that define how code is written in the Python language. Understanding Python syntax is crucial for writing correct and efficient code. This article covered some of the fundamental aspects of Python syntax, such as comments, variables, data types, operators, conditional statements, loops, functions, classes, and modules. With this knowledge, you should be able to start writing your own Python programs.
Subscribe to my newsletter
Read articles from Satyam Aaditya directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by
