Introduction to JavaScript:
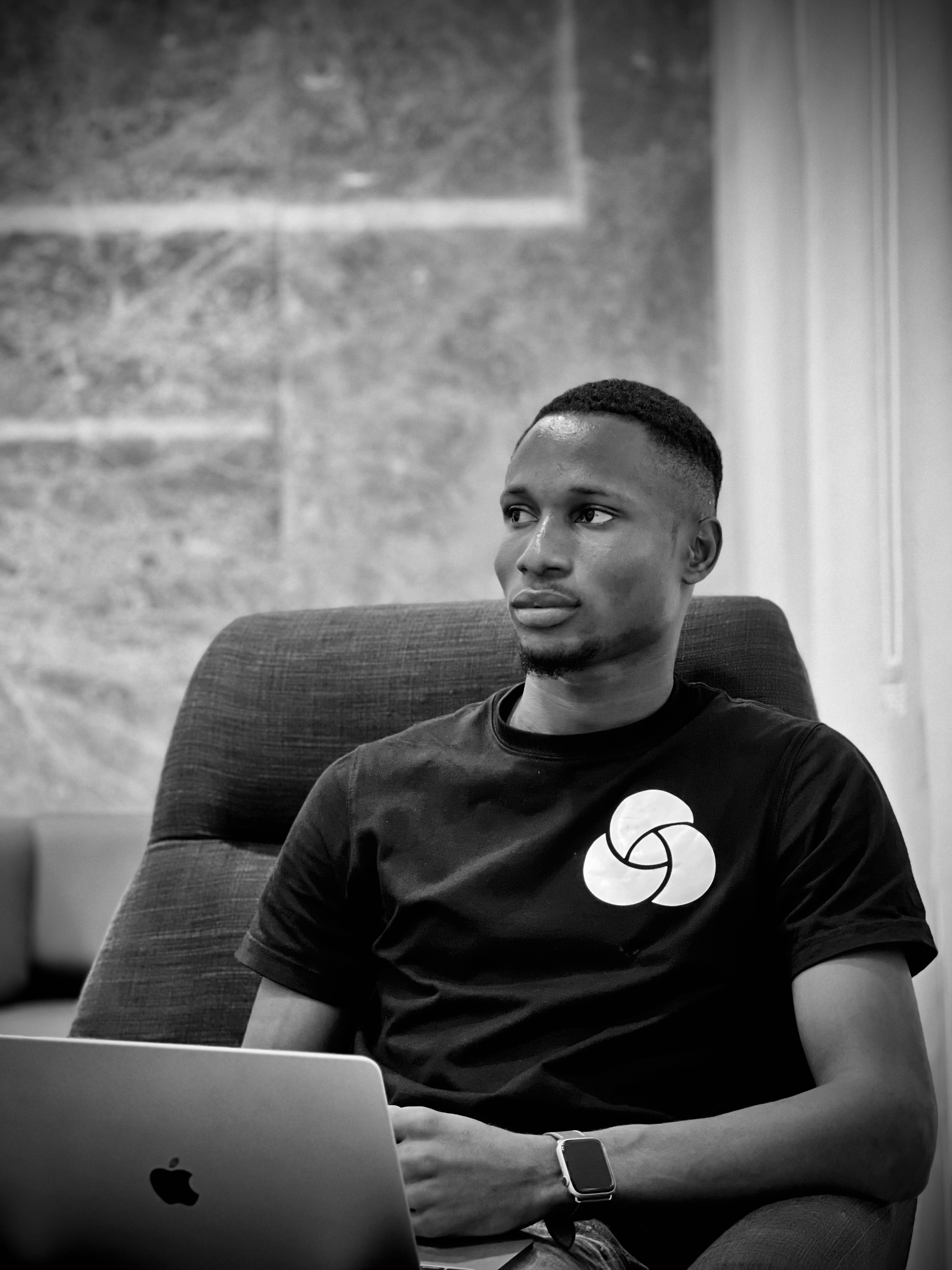
JavaScript is a high-level programming language that is widely used in web development to create dynamic and interactive web pages. It is an object-oriented language that is often used in conjunction with HTML and CSS to create the user interface of a web application. JavaScript runs on the client side, meaning that it is executed by the web browser on the user's computer rather than on the server.
JavaScript is a versatile language that can be used for a wide range of purposes, from simple animations and interactive forms to complex web applications with real-time updates. In this article, we will introduce you to some of the basic concepts of JavaScript programming with examples.
Variables:
In JavaScript, variables are used to store data that can be accessed and manipulated throughout the program. To create a variable, you can use the let
or const
keyword followed by the variable name and an optional initial value. For example:
let age = 25; const PI = 3.14;
In this example, we have created two variables age
and PI
. age
is a variable that stores an integer value of 25, and PI
is a variable that stores a float value of 3.14. The difference between let
and const
is that let
can be reassigned to a new value, while const
cannot.
Data Types:
JavaScript supports several data types, including numbers, strings, booleans, arrays, objects, and null. The typeof
operator can be used to check the type of a variable. For example:
JavaScript:
let num = 42; console.log(typeof num); // Output: "number"
let str = "Hello, world!"; console.log(typeof str); // Output: "string"
let bool = true; console.log(typeof bool); // Output: "boolean"
let arr = [1, 2, 3]; console.log(typeof arr); // Output: "object"
let obj = { name: "John", age: 30 }; console.log(typeof obj); // Output: "object"
let nullVar = null; console.log(typeof nullVar); // Output: "object"
Functions:
Functions are used in JavaScript to encapsulate a block of code that can be reused multiple times throughout the program. To create a function, you can use the function
keyword followed by the function name and a set of parentheses containing any parameters that the function requires. For example:
Css:
function addNumbers(a, b) { return a + b; }
In this example, we have created a function addNumbers
that takes two parameters a
and b
and returns their sum. To call the function, you simply need to pass in the required arguments. For example:
Scss:
let result = addNumbers(2, 3); console.log(result); // Output: 5
Arrays:
Arrays are used in JavaScript to store a collection of data, such as a list of names or numbers. To create an array, you can use square brackets []
and separate the elements with commas. For example:
Bash:
let names = ["John", "Jane", "Bob"]; let numbers = [1, 2, 3, 4, 5];
You can access elements in an array using their index number, starting from 0. For example:
Arduino:
console.log(names[0]); // Output: "John" console.log(numbers[2]); // Output: 3
Loops:
Loops are used in JavaScript to execute a block of code multiple times. The for
loop is one of the most commonly used loops.
Subscribe to my newsletter
Read articles from Ifeanyi Obiania directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by
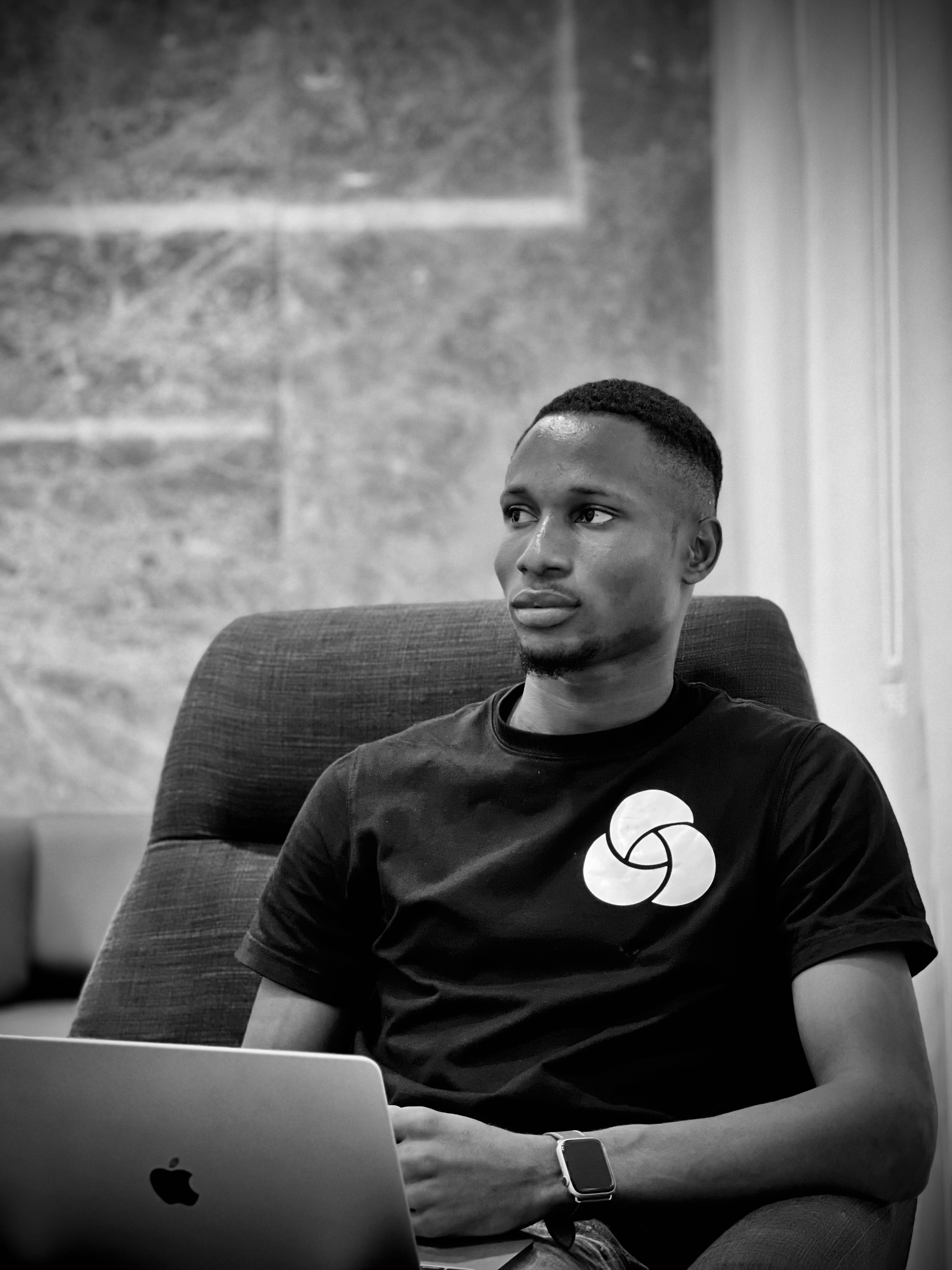
Ifeanyi Obiania
Ifeanyi Obiania
I am Ifeanyi an undergraduate student currently enrolled in the Accounting program at Adekunle Ajasin University in Akungba. Additionally, I am also a self-taught proficient web developer based in Lagos, Nigeria ๐ณ๐ฌ, with expertise across several web development technologies. As a web developer, I am extremely passionate about my work and I thoroughly enjoy sharing my knowledge and experience with other junior developers. I believe in being helpful, efficient, and productive in all my endeavors, and I am constantly striving to enhance my skills and improve my knowledge base. Currently, I actively share my knowledge and projects on Twitter, and I find immense satisfaction in being able to assist other developers in their pursuits. I am always willing to engage in collaborative efforts and welcome opportunities to work with other professionals in the field.