Reversing a String In C
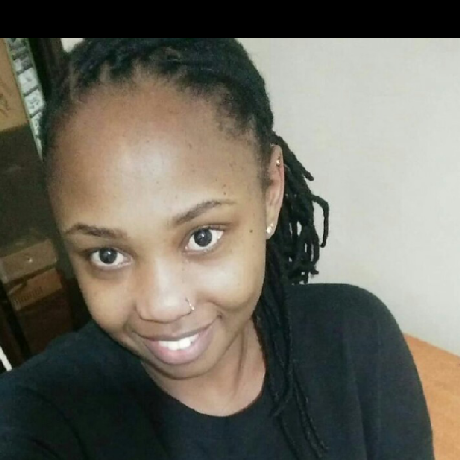
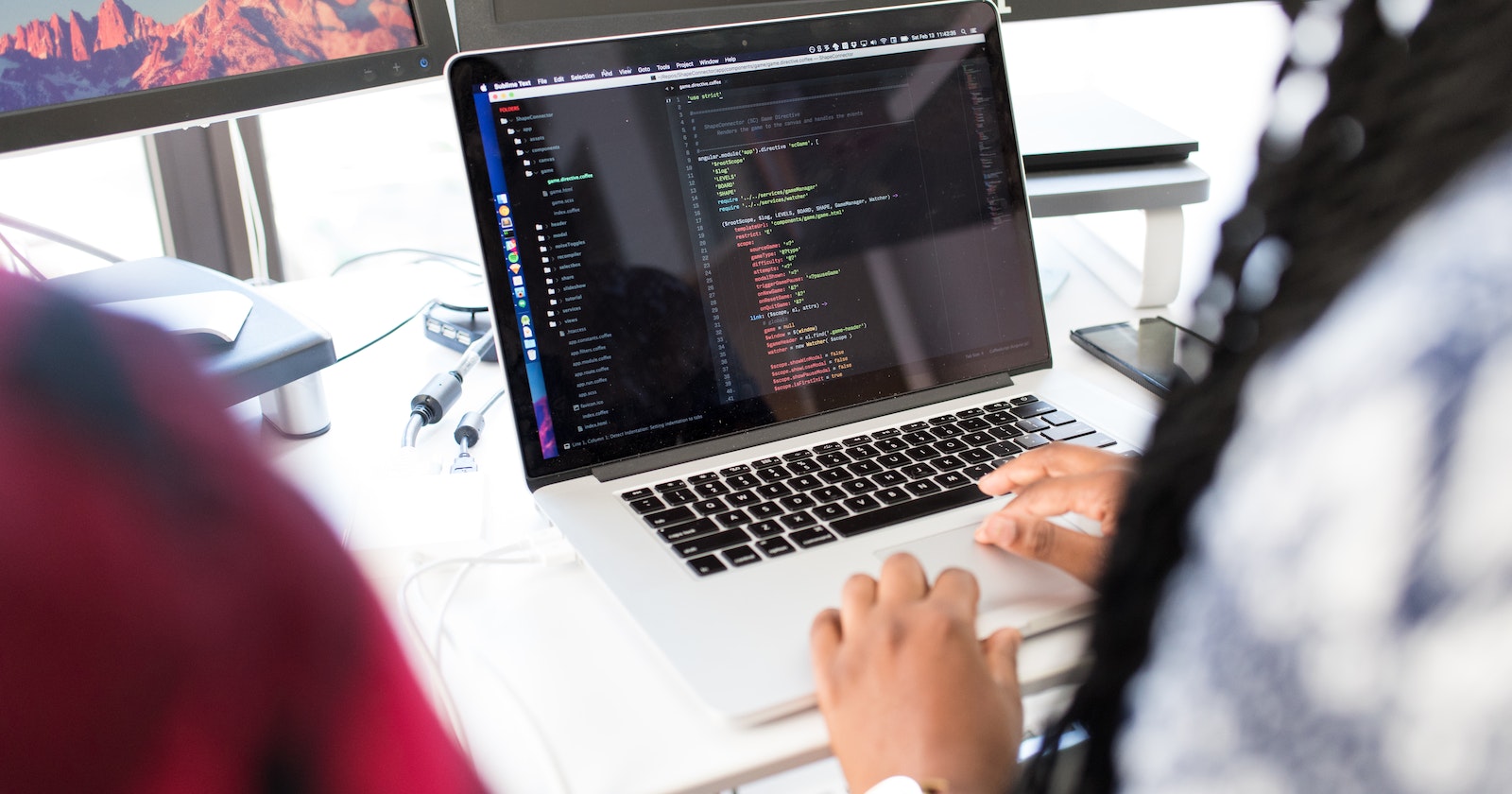
This C program defines two functions to reverse a given string:
_strlen(char *str)
: calculates the length of a stringstr
.reverse_string(char *str)
: reverses the stringstr
by swapping its characters.
The main
function uses reverse_string
to reverse the string "JQK"
and prints the result to the console.
Here's a step-by-step breakdown of what the program does:
The
_strlen
function takes a stringstr
as input and returns its length as an integer. It does this by initializing a variablelen
to 0, then iterating over the characters instr
with a for loop until it reaches the null character ('\0'
). For each character, it incrementslen
by 1. Finally, it returnslen
.The
reverse_string
function takes a stringstr
as input and modifies it in place to reverse its characters. It does this by first calling_strlen
to determine the length ofstr
. It then initializes two variables,i
andj
, to the beginning and end of the string, respectively. It then iterates over the string with a for loop, swapping the characters at positionsi
andj
untili
andj
meet in the middle of the string. It uses a temporary variabletemp
to hold one of the characters during the swap.In the
main
function, a stringstr
containing the characters "JQK" is defined. Thereverse_string
function is then called withstr
as its input, which modifiesstr
in place to reverse its characters. Finally, the reversed string is printed to the console usingprintf
.
The output of the program will be:
Reversed string: KQJ
Subscribe to my newsletter
Read articles from Fridah directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by
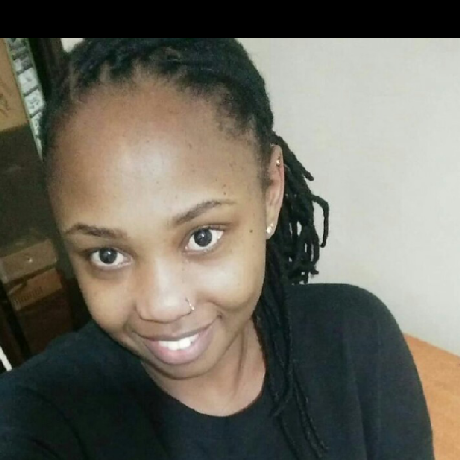