How to Add the Report Viewer Component to an ASP.NET Core Application
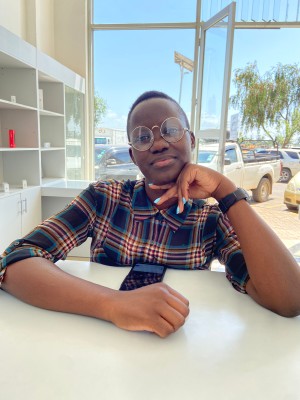
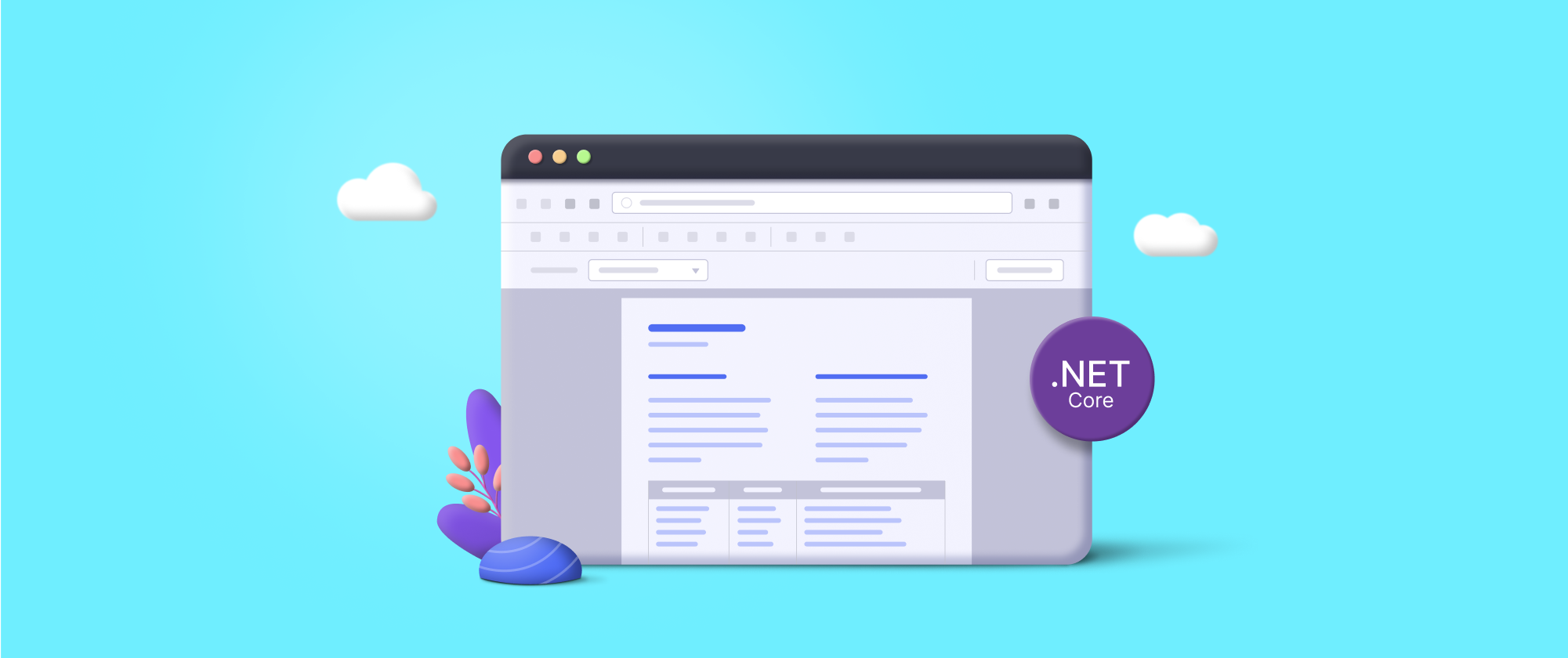
The Report Viewer is a web-based component for visualizing data in meaningful ways and exporting SSRS RDL and RDLC reports.
This blog provides knowledge on how to create an ASP.NET Core reporting web application to display SSRS RDL reports using the Bold Reports ASP.NET Core Report Viewer.
Prerequisites
.NET Core SDK 3.1+
Visual Studio 2019+
Create an ASP.NET Core application.
Open Visual Studio, choose ASP.NET Core Web App, provide the application name, and then click Next.
Create ASP.NET Core Project
Choose the ASP.NET Core version and click Create. Do not select Enable Docker.
Choose .NET Core Version
Install the NuGet packages.
Right-click the project in the solution explorer tab and choose Manage NuGet Packages.
In the Browser tab, search for BoldReports.AspNet.Core and install it in your Core application.
Similarly, install the remaining BoldReports.NET.Core system.
The following table provides details about the packages and their usage.
Package | Purpose |
BoldReports.Net.Core | Creates Web API service used to process the reports. |
BoldReports.AspNet.Core | Contains tag helpers to create client-side reporting control. |
System.Data.SqlClient | This is an optional package. If the RDL report contains the SQL Server or SQL Azure data source, then this package should be installed. The package version should be 4.1.0 or higher. |
Choose NuGet package
And the dependency packages and their usages are as follows.
Package | Purpose |
Exports a report to PDF, Word, or Excel format. It is a base library for the Syncfusion.Pdf.Net.Core, Syncfusion.DocIO.Net.Core, and Syncfusion.XlsIO.Net.Core packages. | |
Syncfusion.Pdf.Net.Core | Exports a report to a PDF file. |
Syncfusion.DocIO.Net.Core | Exports a report to a Word file. |
Syncfusion.XlsIO.Net.Core | Exports a report to an Excel file. |
A base library of the Syncfusion.XlsIO.Net.Core package. | |
Newtonsoft.Json | Serializes and deserializes data for the Report Viewer. It is a mandatory package for the Report Viewer, and the package version should be 10.0.1 or higher. |
Reference the scripts and theme
The following scripts and style sheets are mandatory to use the Report Viewer.
Name | Details |
bold.reports.all.min.css | Contains styles required for Bold Reports components. |
jquery-1.10.2.min.js | External dependent library required for the HTML document traversal and manipulation, event handling , animation, and Ajax. Supported from 1.10 to 3.x |
ej2-maps.min.js | Renders the map item. Add this script only if your report contains the map report item. |
ej2-base.min.js | Render the gauge item. Add these scripts only if your report contains the gauge report item. |
ej2-data.min.js | |
ej2-pdf-export.min.js | |
ej2-svg-base.min.js | |
ej2-lineargauge.min.js | |
ej2-circulargauge.min.js | |
bold.reports.common.min.js | Contains dependent JavaScript components scripts packed in a minified format. |
bold.reports.widgets.min.js | Mandatory to render the Bold Report Viewer. |
ej.chart.min.js | Renders the chart item. Add this script only if your report contains the chart report item. |
bold.report-viewer.min.js | Contains Report Viewer component script. |
Open views shared _layout.cshtml page and reference the following code in the head tag.
<link href="https://cdn.boldreports.com/3.3.23/content/material/bold.reports.all.min.css"
rel="stylesheet" /><script src="https://cdn.boldreports.com/external/jquery-1.10.2.min.js"
type="text/javascript"></script>
<!--Render the gauge item. Add this script only if your report contains the gauge report item. -->
<script src="https://cdn.boldreports.com/3.3.23/scripts/common/ej2-base.min.js"></script>
<script src="https://cdn.boldreports.com/3.3.23/scripts/common/ej2-data.min.js"></script>
<script src="https://cdn.boldreports.com/3.3.23/scripts/common/ej2-pdf-export.min.js"></script>
<script src="https://cdn.boldreports.com/3.3.23/scripts/common/ej2-svg-base.min.js"></script>
<script src="https://cdn.boldreports.com/3.3.23/scripts/data-visualization/ej2-lineargauge.min.js"></script>
<script src="https://cdn.boldreports.com/3.3.23/scripts/data-visualization/ej2-circulargauge.min.js"></script>
<!--Render the map item. Add this script only if your report contains the map report item.-->
<script src="https://cdn.boldreports.com/3.3.23/scripts/data-visualization/ej2-maps.min.js"></script>
<script src="https://cdn.boldreports.com/3.3.23/scripts/common/bold.reports.common.min.js"></script>
<script src="https://cdn.boldreports.com/3.3.23/scripts/common/bold.reports.widgets.min.js"></script>
<!--Render the chart item. Add this script only if your report contains the chart report item.-->
<script src="https://cdn.boldreports.com/3.3.23/scripts/data-visualization/ej.chart.min.js"></script>
<!-- Report Viewer component script-->
<script src="https://cdn.boldreports.com/3.3.23/scripts/bold.report-viewer.min.js"></script>
Configure Bold Reports script manager
Open the layout.cshtml page and replace the code in the body tag as shown in the following code sample.
<body>
<div style="min-height: 600px;width: 100%;">
@RenderBody()
</div>
@RenderSection("Scripts", required: false)
<!-- Bold Reports script manager -->
<bold-script-manager></bold-script-manager>
</body>
Initialize the Report Viewer
Open the home index.cshtml page and replace it with the following code.
<bold-report-viewer id="viewer"></bold-report-viewer>
Add a report
Create a Resource folder in the wwwroot folder in the application. This is where you will keep your RDL Report.
Add sales-order-detail.rdl to the Resources folder.
Configure the Web API
The ASP.NET Core Report Viewer requires the Web API service to process RDL, RDLC, and SSRS report files:
Right-click the controller folder and select Add a new item from the context menu.
In the Add new item dialog, select API controller class, name it ReportViewerController.cs, and click Add.
In the ReportViewerController, add the following using statement.
using System.IO;
using BoldReports.Web.ReportViewer;
4. Inherit the IReportController interface, and then implement its methods.
Note: The IReportController interface has the declaration of action methods that are defined in the Web API controller for processing RDL, RDLC, and SSRS reports. It also handles resource requests from the Report Viewer control.
The ReportHelper class contains helper methods that process a POST or GET request from the Report Viewer control and return the response to it.
Add routing information.
Routing is the process of directing an HTTP request to a controller. The functionality of this processing is implemented in System.Web.Routing.
Add the action parameter to the rout template as in the following code sample.
[Route("api/[controller]/[action]")]
public class ReportViewerController : Controller, IReportController
{
...
}
Enable cross-origin requests
Browser security prevents Report Viewer from making requests to your Web API service when both run in different domains. To allow access to your Web API service from a different domain, we must enable cross-origin requests:
Open the Startup.cs file.
Call AddCors in Startup.ConfigureServices to add CORS services to the app’s service container as in the following code.
public void ConfigureServices(IServiceCollection services)
{
services.AddMvc();
services.AddCors(o => o.AddPolicy("AllowAllOrigins", builder =>
{
builder.AllowAnyOrigin()
.AllowAnyMethod()
.AllowAnyHeader();
}));
}
3. Open ReportViewerController.cs file, add the “EnableCors” attribute to the ReportViewerContrller class and specify the policy name that is given in the startup.configureServices.
[Microsoft.AspNetCore.Cors.EnableCors("AllowAllOrigins")]
public class ReportViewerController : Controller, IReportController
{
private Microsoft.Extensions.Caching.Memory.IMemoryCache _cache;
....
....
}
Register the valid license token
Open the startup.cs file and enter the code sample to register the license token in the configure method. Note: License tokens can be generated from the download section of the Bold Reports site.
Select the embedded reporting and click generate license token.
Copy the token.
In the startup.cs file, register the license token.
Set the ReportPath and ServiceUrl.
This is where you will process and render an RDL report in the browser using the Web API service. The ReportPath property sets the path of the report file while the ReportServiceUrl property specifies the report Web API service URL.
- Open the index.cshtml file and set the reportpath and serviceUrl properties as in the following code sample.
<bold-report-viewer id="viewer" report-path="sales-order-detail.rdl" report-service-url="/api/ReportViewer">
</bold-report-viewer>
Note: Build and run the application to preview the report in the Report Viewer as shown in the following screenshot.
Conclusion
In this blog, you have learned how to display an SSRS RDL report using the Bold Reports ASP.NET Core Report Viewer. To explore further, I recommend you go through our sample reports and documentation.
If you have any questions, please post them in the comments section below. You can also contact us through our contact page or, if you already have an account, you can log in to submit your support question. Feel free to check out the Bold Reports demos and documentation to explore the available tools and their various customization features.
Bold Reports now offers a 15-day free trial with no credit card information required. We welcome you to start a free trial and experience Bold Reports for yourself. Give it a try, and let us know what you think!
Stay tuned to our official Twitter, Facebook, and LinkedIn pages for announcements about new releases.
Subscribe to my newsletter
Read articles from Barbra Weke directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by
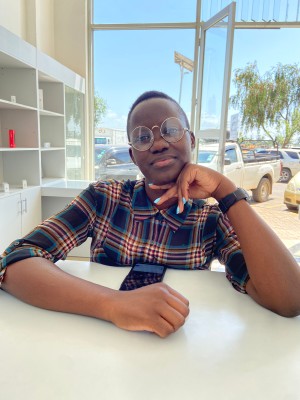
Barbra Weke
Barbra Weke
Barbra is a content producer at Syncfusion who is committed to producing captivating content for Bold Reports products. She works closely with subject matter experts to craft instructional material and collaborates with graphics designers to create engaging content that resonates with the audience.