Understanding Objects in JavaScript :
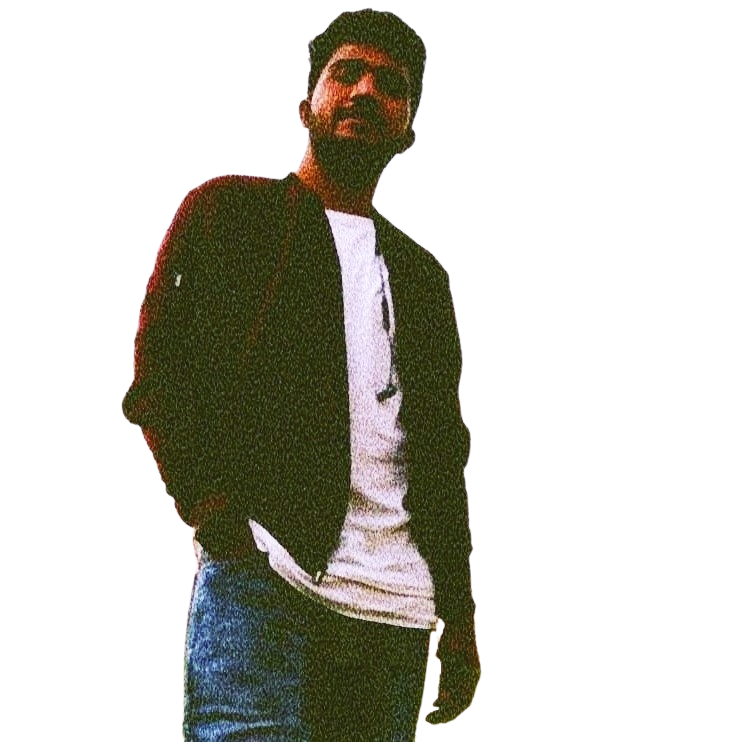
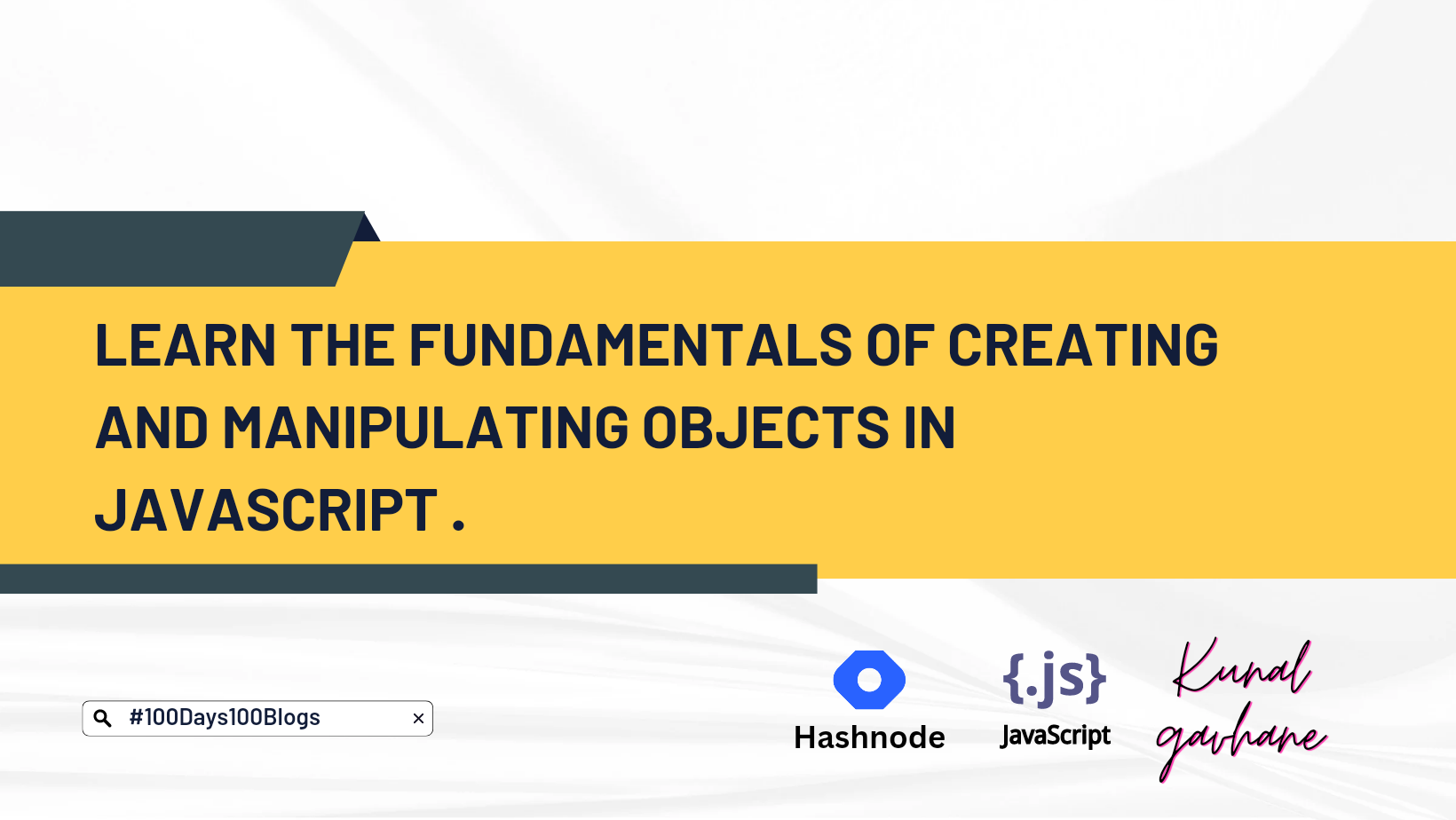
Script is a popular programming language used to create interactive websites and web applications. One of the fundamental concepts of JavaScript is objects. In this article, we will dive deep into objects in JavaScript and explore how they work.
What are Objects in JavaScript?
Objects are one of the data types in JavaScript. They are a collection of related data and functions. In JavaScript, objects are defined using curly braces {} and key-value pairs. A key-value pair is a combination of a key and a value separated by a colon.
Here is an example of an object in JavaScript:
let person = {
name: "John",
age: 30,
city: "New York",
getFullName: function() {
return this.name;
}
};
In this example, we have defined an object called person with three key-value pairs: name, age, and city. The value of the name key is "John", the value of the age key is 30, and the value of the city key is "New York". The object also has a function called getFullName, which returns the value of the name key.
How to Access Object Properties
We can access the properties of an object using dot notation or square bracket notation.
For example, to access the name property of the person object defined above, we can use either of the following notations:
person.name;
person['name'];
Both of these notations will return the value "John".
Adding Properties to an Object
We can add new properties to an object by assigning a value to a new key.
person.email = "john@example.com";
In this example, we have added a new key-value pair to the person object.
Updating Object Properties
We can update the value of an existing property of an object by assigning a new value to the key.
person.age = 35;
In this example, we have updated the value of the age key to 35.
Deleting Properties from an Object
We can delete a property from an object using the delete operator.
delete person.age;
In this example, we have deleted the age property from the person object.
Object Methods :
In addition to properties, an object can also contain functions, which are called methods. We have already seen an example of a method in the person object defined above.
getFullName: function() {
return this.name;
}
In this example, we have defined a method called getFullName, which returns the value of the name property of the object. To call a method, we use dot notation.
person.getFullName();
This will call the getFullName method of the person object and return the value of the name property.
Object Constructors :
In JavaScript, we can create objects using constructors. A constructor is a function that is used to create objects of a certain type.
Here is an example of an object constructor in JavaScript:
function Person(name, age, city) {
this.name = name;
this.age = age;
this.city = city;
this.getFullName = function() {
return this.name;
}
};
let person1 = new Person("John", 30, "New York");
In this example, we have defined a constructor called Person, which takes three arguments: name, age, and city. The constructor sets the name, age, and city properties of the object to the values passed in as arguments. It also defines a method called getFullName.
To create a new object of type Person, we use the new operator.
let person1 = new Person("John", 30, "New York");
This will create a new object of type Person with the name.
This blog is part of my #100Days100Blogs ๐ challenge on #hashnode.
To joined me I'm this challenge connect with me on following handles ๐
๐ฆ Twitter
๐ LinkedIn
Subscribe to my newsletter
Read articles from kunal gavhane directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by
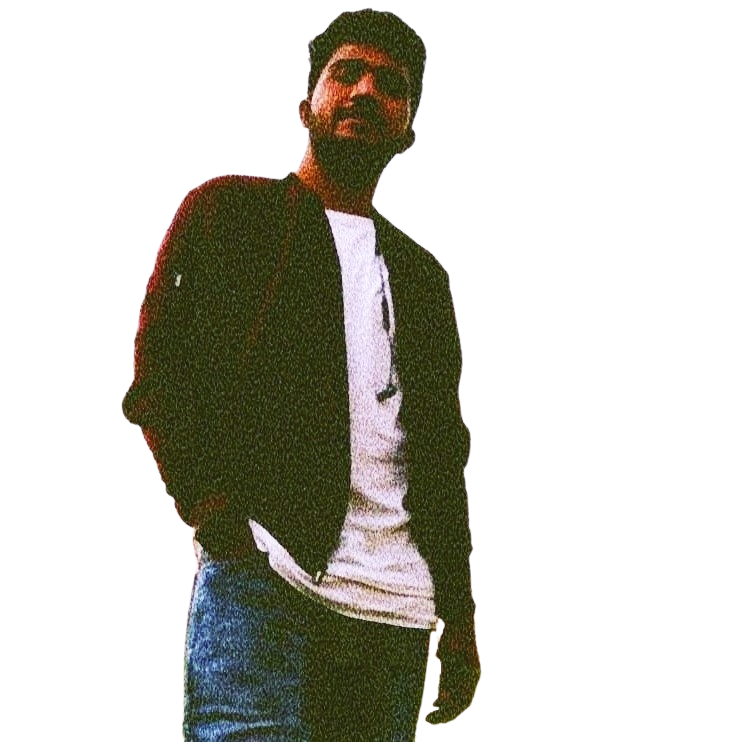
kunal gavhane
kunal gavhane
Where people see a problem, we see an opportunity and a new project! Hello, I am an Engineer;