Mastering Arrays in JavaScript: A Comprehensive Guide for Developers.
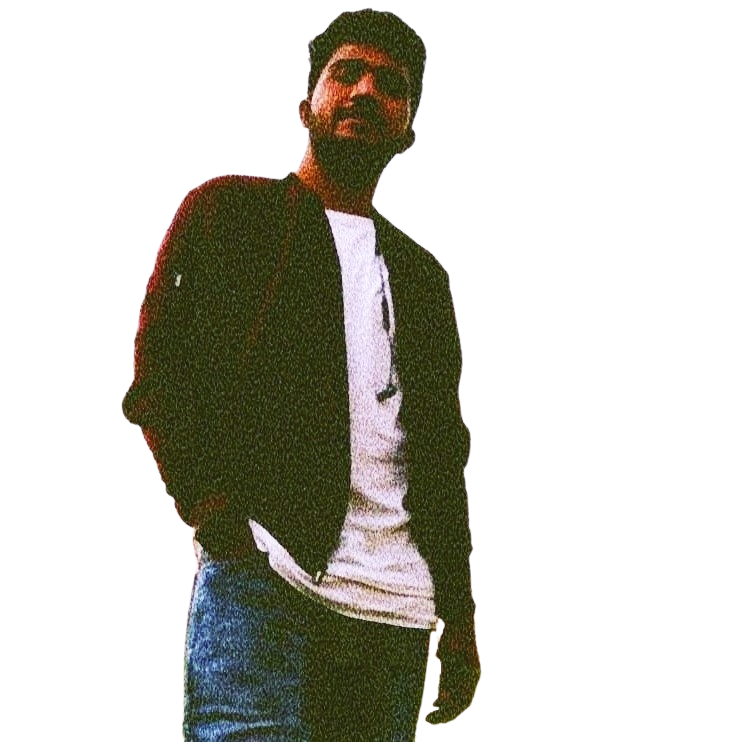
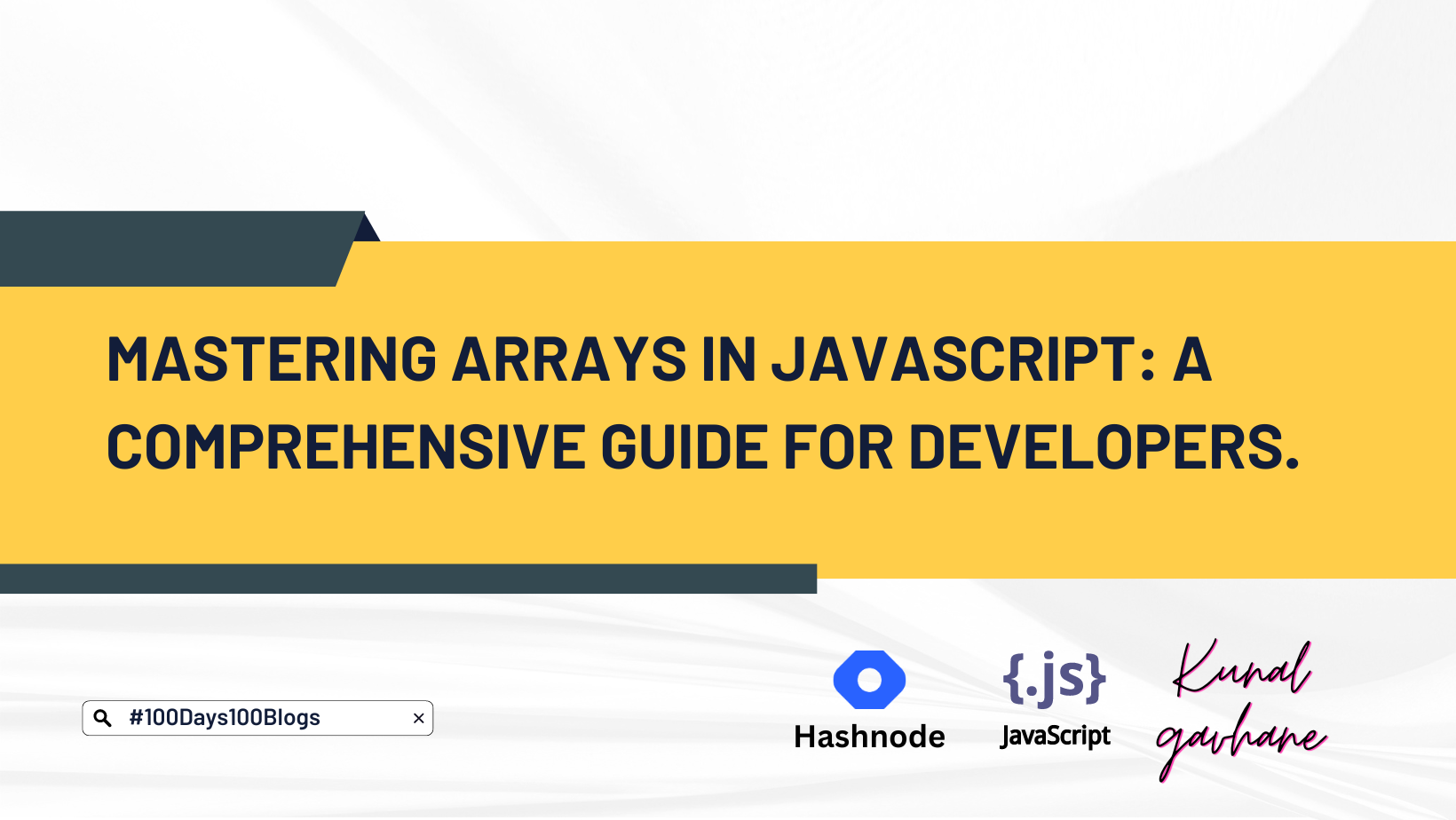
In this article, I will provide a comprehensive guide to arrays in JavaScript, a powerful feature that allows developers to store and manipulate collections of data in a single variable. My name is Kunal Gavhane, and I have been working as a web developer for the past 1.5 years, during which time I have gained extensive experience using JavaScript. With this guide, I hope to help both beginners and experienced developers alike gain a deeper understanding of arrays and how to use them effectively in their projects.
What is an array?
An array in JavaScript is a data structure that allows you to store and access multiple values in a single variable. It is essentially a collection of values, each of which is assigned a unique index. Arrays can hold any data type, including numbers, strings, objects, and even other arrays.
In simple words,
Imagine that you have a big collection of different types of items, like books, movies, video games, and toys. Now, you want to keep them all organized in a single place so you can find what you're looking for quickly and easily. That's where an array comes in!
An array is like a digital shelf or container where you can store all your items in one place. Each item in the array is given a special index number that tells the computer where it is located in the array. This way, you can easily access a specific item in the array just by referring to its index number.
For example, if you have an array of books and you want to find a specific book, you can look up its index number and go straight to it. It's like having a digital library where everything is organized and easy to find!
To create an array in JavaScript, you can use square brackets [] and separate the values with commas. For example:
let myArray = [1, 2, 3, 4, 5];
You can also create an empty array by using empty square brackets:
let emptyArray = [];
Once you have an array, you can access individual values by using their index. For example, to access the second value in myArray
, you would use the index 1 (remember, indices start from zero):
let secondValue = myArray[1];
You can also modify the values in an array by assigning a new value to a specific index:
myArray[2] = 6;
In JavaScript, array methods are built-in functions that can be used to perform various operations on arrays. These methods allow you to add, remove, modify, filter, search, and iterate over the elements of an array. There are several built-in array methods in JavaScript that you can use to manipulate arrays.
Here is an overview of all the array methods in JavaScript:
Adding and removing elements
1) push():
Adds one or more elements to the end of an array and returns the new length of the array.
let myArray = [1, 2, 3];
myArray.push(4);
// myArray is now [1, 2, 3, 4]
2) pop():
- Removes the last element from an array and returns it.
let myArray = [1, 2, 3];
let lastElement = myArray.pop();
// lastElement is 3, myArray is now [1, 2]
3) unshift():
Adds one or more elements to the beginning of an array and returns the new length of the array.
let myArray = [1, 2, 3];
myArray.unshift(0);
// myArray is now [0, 1, 2, 3]
4) shift():
Removes the first element from an array and returns it.
let myArray = [1, 2, 3];
let firstElement = myArray.shift();
// firstElement is 1, myArray is now [2, 3]
Modifying existing elements
1) splice():
- Adds or removes elements from an array at a specified index.
let myArray = [1, 2, 3];
myArray.splice(1, 1, 4);
// myArray is now [1, 4, 3]
2) fill():
- Fills all the elements of an array with a static value, starting from a specified index and ending at a specified index.
let myArray = [1, 2, 3];
myArray.fill(0, 1, 2);
// myArray is now [1, 0, 3]
3) reverse():
- Reverses the order of the elements in an array.
let myArray = [1, 2, 3];
myArray.reverse();
// myArray is now [3, 2, 1]
4) sort():
Sorts the elements of an array in place.
let myArray = [3, 1, 2];
myArray.sort();
// myArray is now [1, 2, 3]
Filtering and searching
1) filter():
- Creates a new array with all elements that pass the test implemented by the provided function.
let myArray = [1, 2, 3];
let filteredArray = myArray.filter((num) => num > 1);
// filteredArray is now [2, 3]
2) find():
- Returns the first element in an array that satisfies the provided testing function.
let myArray = [1, 2, 3];
let foundElement = myArray.find((num) => num > 1);
// foundElement is 2
3) indexOf():
- Returns the index of the first occurrence of a specified element in an array, or -1 if it is not found.
let myArray = [1, 2, 3];
let index = myArray.indexOf(2);
// index is 1
Iterating over elements
1) forEach():
- Calls a function for each element in an array.
let myArray = [1, 2, 3];
myArray.forEach((num) => console.log(num));
// logs 1, 2,
Summary:
Arrays are a fundamental data structure in JavaScript that allows you to store and manipulate collections of data. By understanding how to create, access, and manipulate arrays, you can greatly enhance your ability to write efficient and effective code. Additionally, using array methods in JavaScript can greatly simplify your code by providing built-in functionality for common operations such as adding, removing, and searching for elements within an array. By mastering these concepts and techniques, you can become a more proficient JavaScript developer and achieve greater success in your programming endeavours.
If you found this article helpful in your understanding of arrays and array methods in JavaScript, please consider sharing it with your friends and colleagues who may also find it useful. Your support in spreading the word is greatly appreciated! Additionally, I welcome any feedback or comments you may have on this article. Thank you for taking the time to read and engage with my article.
This blog is part of my #100Days100Blogs ๐ challenge on #hashnode.
To join me In this challenge connect with me on the following handles ๐
Subscribe to my newsletter
Read articles from kunal gavhane directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by
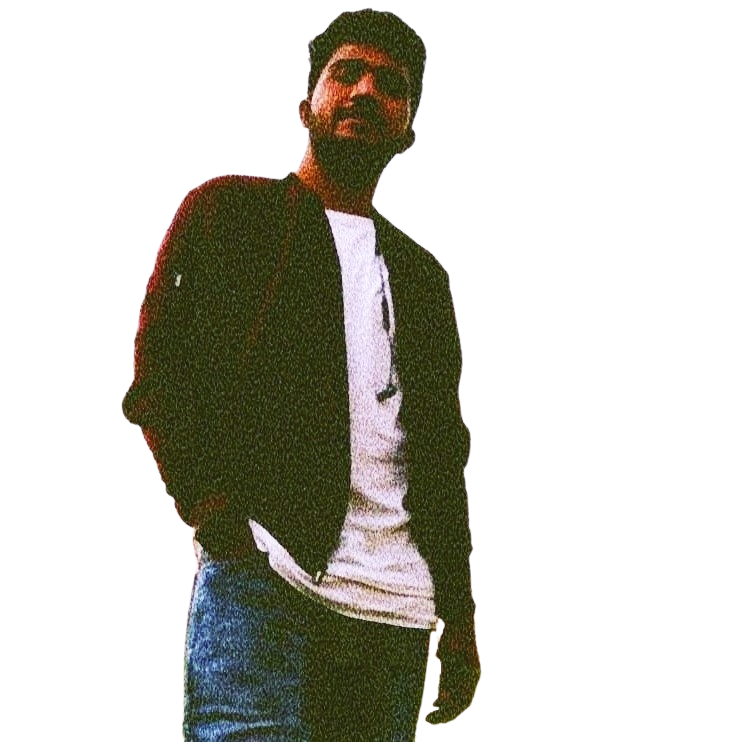
kunal gavhane
kunal gavhane
Where people see a problem, we see an opportunity and a new project! Hello, I am an Engineer;