Python String

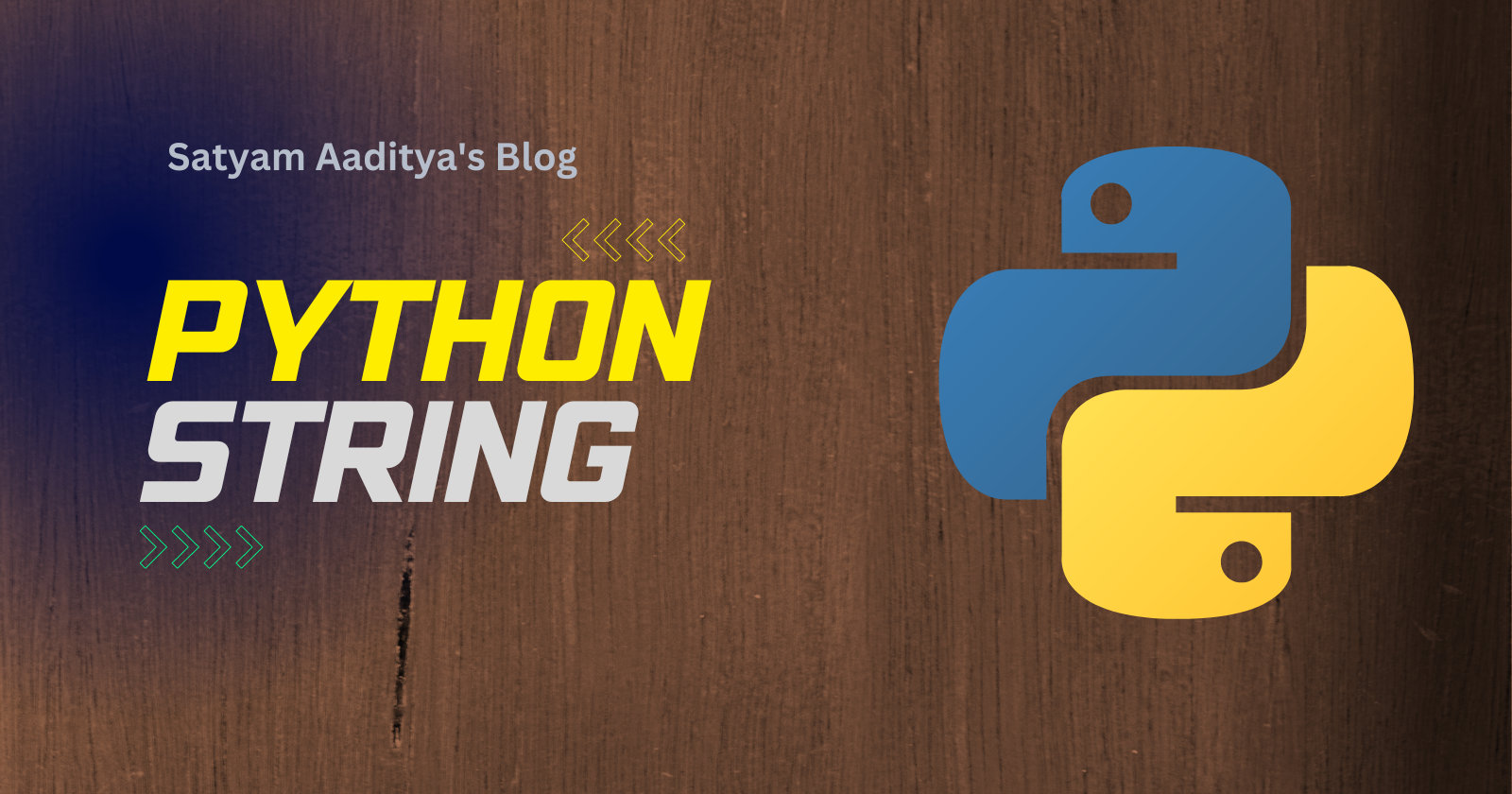
Strings are an essential part of Python programming. A string is a sequence of characters enclosed in single or double quotes. In this article, we will explore Python strings in detail.
Creating a String
You can create a string by enclosing a sequence of characters in single or double quotes.
Example:
# single quotes
string1 = 'Hello World!'
# double quotes
string2 = "Hello World!"
Strings can also span multiple lines using triple quotes.
Example:
string3 = """Hello
World!"""
Accessing Characters in a String
You can access individual characters in a string using indexing. In Python, indexing starts at 0, which means the first character of a string is at index 0.
Example:
string = "Hello World!"
print(string[0]) # Output: H
print(string[6]) # Output: W
You can also access a range of characters in a string using slicing.
Example:
string = "Hello World!"
print(string[0:5]) # Output: Hello
String Methods
Python provides several built-in methods that you can use to manipulate strings. Some of the commonly used string methods are:
a. len(): Returns the length of a string.
Example:
string = "Hello World!"
print(len(string)) # Output: 12
b. strip(): Removes whitespace characters from the beginning and end of a string.
Example:
string = " Hello World! "
print(string.strip()) # Output: Hello World!
c. lower(): Converts all characters in a string to lowercase.
Example:
string = "Hello World!"
print(string.lower()) # Output: hello world!
d. upper(): Converts all characters in a string to uppercase.
Example:
string = "Hello World!"
print(string.upper()) # Output: HELLO WORLD!
e. replace(): Replaces a substring in a string with another substring.
Example:
string = "Hello World!"
print(string.replace("World", "Python")) # Output: Hello Python!
String Concatenation
You can concatenate two or more strings using the + operator.
Example:
string1 = "Hello"
string2 = "World"
string3 = string1 + " " + string2
print(string3) # Output: Hello World
String Formatting
String formatting allows you to insert values into a string. You can use the % operator or the format() method for string formatting.
Example using the % operator:
name = "Alice"
age = 25
print("My name is %s and I am %d years old." % (name, age))
Output:
My name is Alice and I am 25 years old.
Example using the format() method:
name = "Alice"
age = 25
print("My name is {} and I am {} years old.".format(name, age))
Output:
My name is Alice and I am 25 years old.
In conclusion, Python strings are a powerful and versatile data type. With the help of string methods, concatenation, and formatting, you can manipulate and process strings in a variety of ways.
Subscribe to my newsletter
Read articles from Satyam Aaditya directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by
