Python Lists

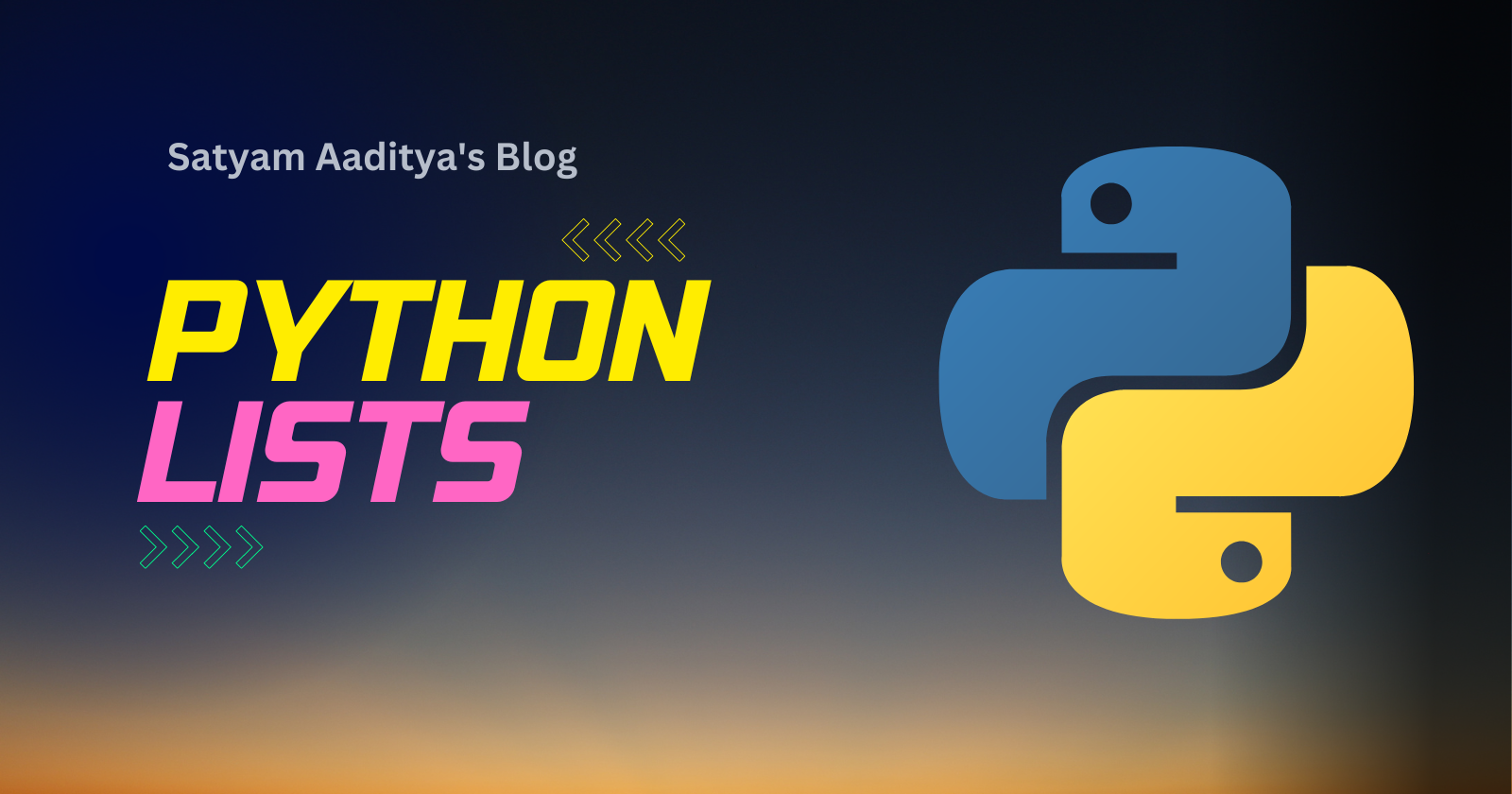
Python lists are one of the most versatile and commonly used data structures in Python. They are used to store a collection of items that can be of different data types, such as integers, strings, and even other lists. In this article, we will discuss lists in Python, including how to create, access, and modify them.
Creating a List
You can create a list in Python by enclosing a sequence of items in square brackets, separated by commas.
Example:
my_list = [1, 2, 3, "four", 5.6]
This creates a list called my_list
that contains integers, a string, and a float.
Accessing List Items
You can access individual items in a list by using their index, which starts at 0 for the first item in the list.
Example:
my_list = [1, 2, 3, "four", 5.6]
print(my_list[0]) # Output: 1
print(my_list[3]) # Output: four
You can also access a range of items in a list using slicing.
Example:
my_list = [1, 2, 3, "four", 5.6]
print(my_list[1:4]) # Output: [2, 3, "four"]
Modifying List Items
You can modify items in a list by using their index and assigning a new value to them.
Example:
my_list = [1, 2, 3, "four", 5.6]
my_list[0] = "one"
print(my_list) # Output: ["one", 2, 3, "four", 5.6]
Adding and Removing List Items
You can add items to a list using the append()
method, which adds a single item to the end of the list, or the extend()
method, which adds multiple items to the end of the list.
Example:
my_list = [1, 2, 3, "four", 5.6]
my_list.append("six")
print(my_list) # Output: [1, 2, 3, "four", 5.6, "six"]
my_list.extend([7, 8, 9])
print(my_list) # Output: [1, 2, 3, "four", 5.6, "six", 7, 8, 9]
You can remove items from a list using the remove()
method, which removes the first occurrence of an item in the list, or the pop()
method, which removes an item at a specific index and returns its value.
Example:
my_list = [1, 2, 3, "four", 5.6]
my_list.remove("four")
print(my_list) # Output: [1, 2, 3, 5.6]
value = my_list.pop(1)
print(value) # Output: 2
print(my_list) # Output: [1, 3, 5.6]
List Methods
Python provides several built-in methods that you can use to manipulate lists. Some of the commonly used list methods are:
1. len(): Returns the number of items in a list.
Example:
my_list = [1, 2, 3, "four", 5.6]
print(len(my_list)) # Output: 5
2. sort(): Sorts the items in a list in ascending order.
Example:
my_list = [4, 2, 6, 1, 5]
my_list.sort()
print(my_list) # Output: [1, 2, 4, 5, 6]
3. reverse(): Reverses the order of items in a list.
Example:
my_list = [1, 2, 3, "four", 5.6]
my_list.reverse()
print(my_list) # Output: [5.6, "four", 3, 2, 1]
4. index(): Returns the index of the first occurrence of an item in a list.
Example:
my_list = [1, 2, 3, "four", 5.6]
print(my_list.index(3)) # Output: 2
5. count(): Returns the number of times an item appears in a list.
Example:
my_list = [1, 2, 3, "four", 5.6, 2, 3]
print(my_list.count(2)) # Output: 2
Conclusion
In this article, we have covered the basics of Python lists, including how to create, access, and modify them. We have also looked at some of the commonly used list methods in Python. Lists are a powerful and versatile data structure in Python that can be used to store and manipulate large collections of data. With the knowledge gained from this article, you should now be able to use lists effectively in your Python programs.
Subscribe to my newsletter
Read articles from Satyam Aaditya directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by
