CodeWars (6 kyu) Consecutive strings - Javascript
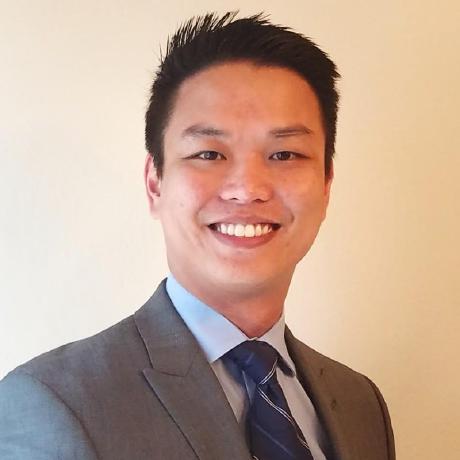
Description:
You are given an array(list) strarr
of strings and an integer k
. Your task is to return the first longest string consisting of k consecutive strings taken in the array.
Examples:
strarr = ["tree", "foling", "trashy", "blue", "abcdef", "uvwxyz"], k = 2
Concatenate the consecutive strings of strarr by 2, we get:
treefoling (length 10) concatenation of strarr[0] and strarr[1]
folingtrashy (" 12) concatenation of strarr[1] and strarr[2]
trashyblue (" 10) concatenation of strarr[2] and strarr[3]
blueabcdef (" 10) concatenation of strarr[3] and strarr[4]
abcdefuvwxyz (" 12) concatenation of strarr[4] and strarr[5]
Two strings are the longest: "folingtrashy" and "abcdefuvwxyz".
The first that came is "folingtrashy" so
longest_consec(strarr, 2) should return "folingtrashy".
In the same way:
longest_consec(["zone", "abigail", "theta", "form", "libe", "zas", "theta", "abigail"], 2) --> "abigailtheta"
n being the length of the string array, if n = 0
or k > n
or k <= 0
return "" (return Nothing
in Elm, "nothing" in Erlang).
Note
consecutive strings : follow one after another without an interruption
Sample Tests
const { assert } = require('chai');
describe("longestConsec",function() {
it("Basic tests",function() {
assert.strictEqual(longestConsec(["zone", "abigail", "theta", "form", "libe", "zas"], 2), "abigailtheta")
assert.strictEqual(longestConsec(["ejjjjmmtthh", "zxxuueeg", "aanlljrrrxx", "dqqqaaabbb", "oocccffuucccjjjkkkjyyyeehh"], 1), "oocccffuucccjjjkkkjyyyeehh")
assert.strictEqual(longestConsec([], 3), "")
assert.strictEqual(longestConsec(["itvayloxrp","wkppqsztdkmvcuwvereiupccauycnjutlv","vweqilsfytihvrzlaodfixoyxvyuyvgpck"], 2), "wkppqsztdkmvcuwvereiupccauycnjutlvvweqilsfytihvrzlaodfixoyxvyuyvgpck")
assert.strictEqual(longestConsec(["wlwsasphmxx","owiaxujylentrklctozmymu","wpgozvxxiu"], 2), "wlwsasphmxxowiaxujylentrklctozmymu")
assert.strictEqual(longestConsec(["zone", "abigail", "theta", "form", "libe", "zas"], -2), "")
assert.strictEqual(longestConsec(["it","wkppv","ixoyx", "3452", "zzzzzzzzzzzz"], 3), "ixoyx3452zzzzzzzzzzzz")
assert.strictEqual(longestConsec(["it","wkppv","ixoyx", "3452", "zzzzzzzzzzzz"], 15), "")
assert.strictEqual(longestConsec(["it","wkppv","ixoyx", "3452", "zzzzzzzzzzzz"], 0), "")
})
})
Solution
To solve this kata, we'll be taking in an array of strings strarr
and an integer k
. It is possible that n = 0
or k > n
or k <= 0
, in which case we'll want to return ""
. Otherwise, we'll want to return the first, longest string consisting of k
consecutive strings.
First, let's work through the pseudocode:
function longestConsec(strarr, k) {
// Iterate over the array of strings
// Concatenate k amount of elements together
// Determine which concatenated string has the greatest length
// If there are multiple concatenated strings with the same max length, return the first instance
// If n = 0 or k > n or k <= 0, return ""
}
Now that we have an idea of how to proceed, let's start working through the coding solution.
function longestConsec(strarr, k) {
// Need a variable to keep track of the longest, concatenated string we've run into
let longest = ""
// Iterate over the array of strings
for (let i = 0; i <= strarr.length-k; i++){
// Concatenate `k` amount of elements together
let tempStr = strarr.slice(i, i+k).join("")
// Determine which concatenated string has the greatest length
// If there are multiple concatenated strings with the same max length, return the first instance
if (tempStr.length > longest.length){
longest = tempStr
}
}
// If n = 0 or k > n or k <= 0, return ""
return k > 0 ? longest : ""
}
So there are a few pieces in the code we should make note of:
We'll need two additional variables.
a.
longest
which will hold the longest string we've seenb.
tempStr
which will hold the current concatenated string within our loopOur for loop will start at index
0
and will only run while it is less than or equal to the array length minusk
. This ensures our for loop does not perform additional operations on the remaining elements in the array that would not havek
amount of successors.We are forming the current concatenated string by using
slice
.slice
allows us to return a portion of the array, with astart
andend
index (end
not included). Therefore, we'll slice from our current index in the loopi
up toi + k.
Because of the way we set up our
if
statement to compare string lengths, we will only return the first instance of the greatest concatenated lengthLastly, we'll want to remember what we should do if
k<=0
(which is toreturn ''
).
Subscribe to my newsletter
Read articles from Nam Nguyen directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by
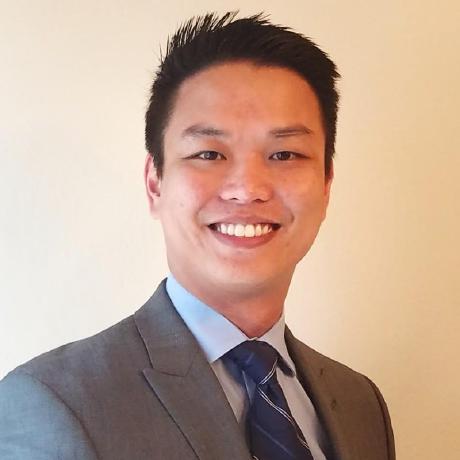
Nam Nguyen
Nam Nguyen
A passionate software engineer providing elegant, innovative, and accessible solutions. When I’m not click-clacking away at my keyboard, you’ll probably find me lost in a book, trying a new recipe/restaurant, or having a blast with my beautiful wife and precocious daughter. I have 10+ years of IT experience and look forward to discussing ways we can join hands for our next adventure!