Hyperparameter Tuning and Cross-Validation: An In-Depth Guide
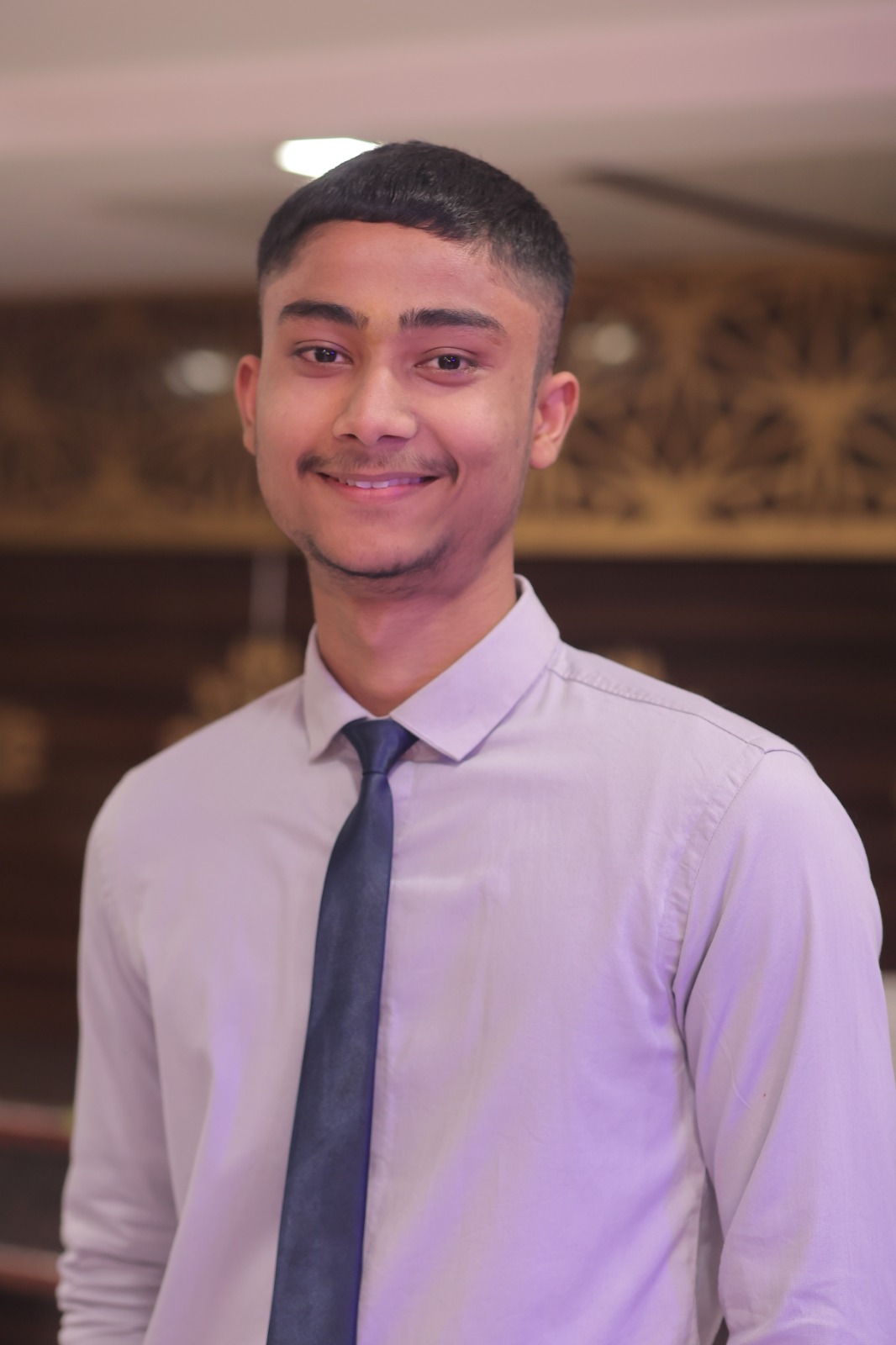
In machine learning, we often need to tune the parameters of our models to get the best performance. Hyperparameter tuning and cross-validation are two powerful techniques that can help us find the optimal set of parameters for a given model.
In this blog post, we will explore hyperparameter tuning and cross-validation in-depth, including their importance, practical implementation, and use cases.
Hyperparameter Tuning
Hyperparameters are parameters that are set before training a model, such as the learning rate, regularization coefficient, or the number of hidden layers in a neural network. The performance of a model depends heavily on these hyperparameters, and finding the optimal set of hyperparameters can make a significant difference in the model's accuracy.
Hyperparameter tuning is the process of finding the optimal set of hyperparameters for a given model. There are several techniques for hyperparameter tuning, including grid search, random search, and Bayesian optimization.
Grid search is a brute-force method of hyperparameter tuning that involves evaluating the model's performance for every possible combination of hyperparameters in a predefined range. While it is simple and easy to implement, it can be computationally expensive and time-consuming, especially for models with many hyperparameters.
Random search is another method of hyperparameter tuning that randomly samples hyperparameters from a predefined range. This technique is more efficient than grid search for models with many hyperparameters, as it explores a more diverse range of hyperparameters.
Bayesian optimization is a more advanced method of hyperparameter tuning that uses probabilistic models to predict the performance of a model for a given set of hyperparameters. This technique can be more efficient than grid search and random search, as it can exploit the structure of the search space and learn from previous evaluations.
Overall, hyperparameter tuning is a critical step in machine learning that can significantly improve a model's accuracy. By finding the optimal set of hyperparameters, we can ensure that our model performs well on unseen data.
Cross-Validation
Cross-validation is a technique used to evaluate a model's performance on unseen data. It involves splitting the data into several subsets, training the model on some subsets, and evaluating it on the remaining subsets. This process is repeated several times, and the results are averaged to get an estimate of the model's performance on unseen data.
The most common type of cross-validation is k-fold cross-validation, where the data is divided into k equally sized subsets. The model is trained k times, each time using a different subset as the validation set, and the results are averaged.
Cross-validation is important because it provides a more accurate estimate of a model's performance on unseen data than a simple train/test split. By evaluating the model on several subsets of the data, we can get a better sense of how well it can generalize to new, unseen data.
Practical Implementation
Hyperparameter tuning and cross-validation can be easily implemented in Python using popular machine-learning libraries such as Scikit-Learn.
Here is an example of hyperparameter tuning using grid search in Scikit-Learn:
from sklearn.model_selection import GridSearchCV
from sklearn.ensemble import RandomForestClassifier
params = {
'n_estimators': [100, 200, 300],
'max_depth': [5, 10, 15]
}
model = RandomForestClassifier()
grid_search = GridSearchCV(model, params, cv=5)
grid_search.fit(X_train, y_train)
best_params = grid_search.best_params_
In this example, we use a random forest classifier and grid search to find the optimal set of hyperparameters for the model. We define a range of values for the number of trees (n_estimators) and the maximum depth of the trees (max_depth). We then use GridSearchCV to perform a grid search over these hyperparameters, with a cross-validation of 5. After fitting the model, we can obtain the best set of hyperparameters using the best_params_ attribute.
Here is an example of k-fold cross-validation in Scikit-Learn:
from sklearn.model_selection import KFold
from sklearn.ensemble import RandomForestClassifier
from sklearn.metrics import accuracy_score
kf = KFold(n_splits=5)
model = RandomForestClassifier(n_estimators=100, max_depth=10)
for train_index, val_index in kf.split(X):
X_train, X_val = X[train_index], X[val_index]
y_train, y_val = y[train_index], y[val_index]
model.fit(X_train, y_train)
y_pred = model.predict(X_val)
accuracy = accuracy_score(y_val, y_pred)
print(f'Accuracy: {accuracy}')
In this example, we use k-fold cross-validation to evaluate the performance of a random forest classifier with 100 trees and a maximum depth of 10. We define a KFold object with 5 splits and loop over the splits to train and evaluate the model on each split. We compute the accuracy of the model on each validation set using the accuracy_score function from Scikit-Learn.
Use Cases
Hyperparameter tuning and cross-validation are used extensively in machine learning, particularly in deep learning and computer vision.
For example, in image classification tasks, hyperparameter tuning can be used to find the optimal learning rate, batch size, and number of epochs for training a convolutional neural network. Cross-validation can be used to evaluate the performance of the model on different subsets of the data, and to ensure that the model can generalize to new, unseen images.
In natural language processing tasks, hyperparameter tuning can be used to find the optimal size of the embedding layer, the number of hidden layers in a recurrent neural network, and the dropout rate. Cross-validation can be used to evaluate the performance of the model on different subsets of the data, and to ensure that the model can generalize to new, unseen text data.
Conclusion
Hyperparameter tuning and cross-validation are powerful techniques that can help us find the optimal set of hyperparameters for a given model, and evaluate its performance on unseen data. By implementing these techniques in Python using popular machine learning libraries such as Scikit-Learn, we can improve the accuracy of our models and ensure that they can generalize to new, unseen data. Hope you got value out of this article. Subscribe to the newsletter to get more such updates. Also, any feedback on the article is appreciated.
Thanks :)
Subscribe to my newsletter
Read articles from Rhythm Rawat directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by
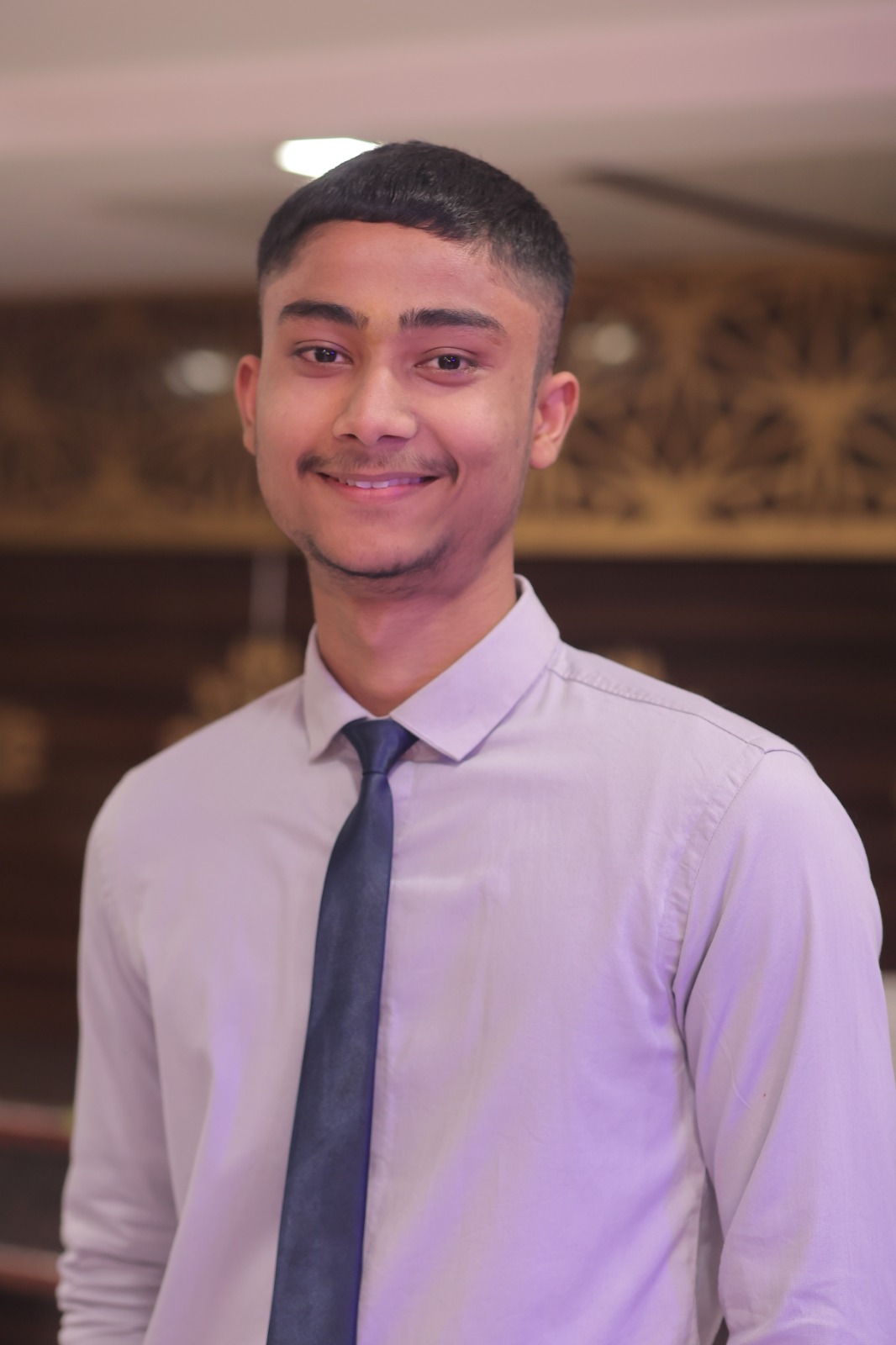
Rhythm Rawat
Rhythm Rawat
Machine learning enthusiast with a strong emphasis on computer vision and deep learning. Skilled in using well-known machine learning frameworks like TensorFlow , scikit-learn and PyTorch for effective model development. Familiarity with transfer learning and fine-tuning pre-trained models to achieve better results with limited data. Proficient in Python, Machine Learning, SQL, and Flask.