Maps in JavaScript
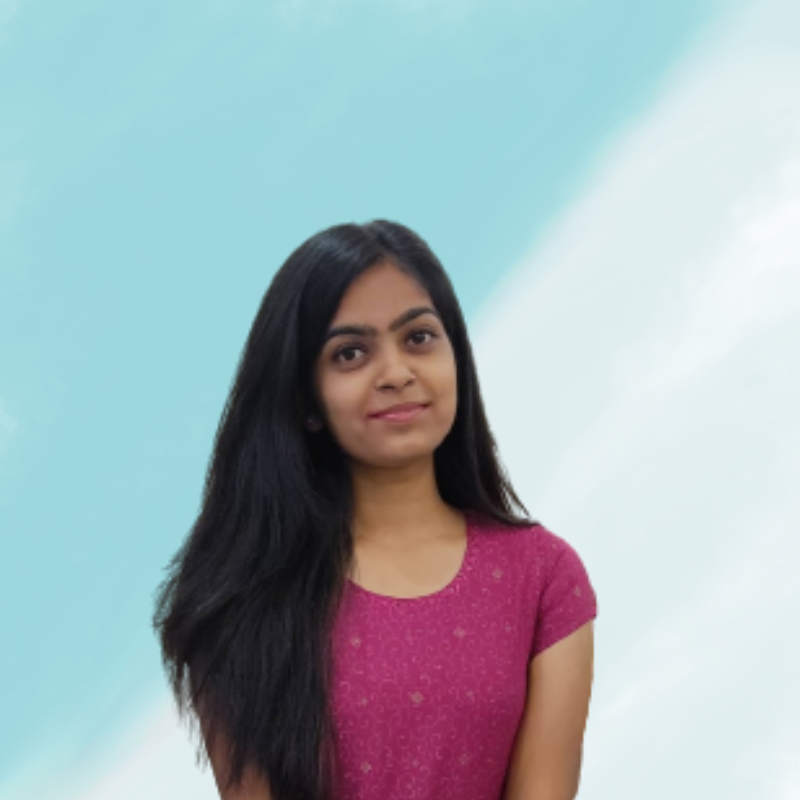
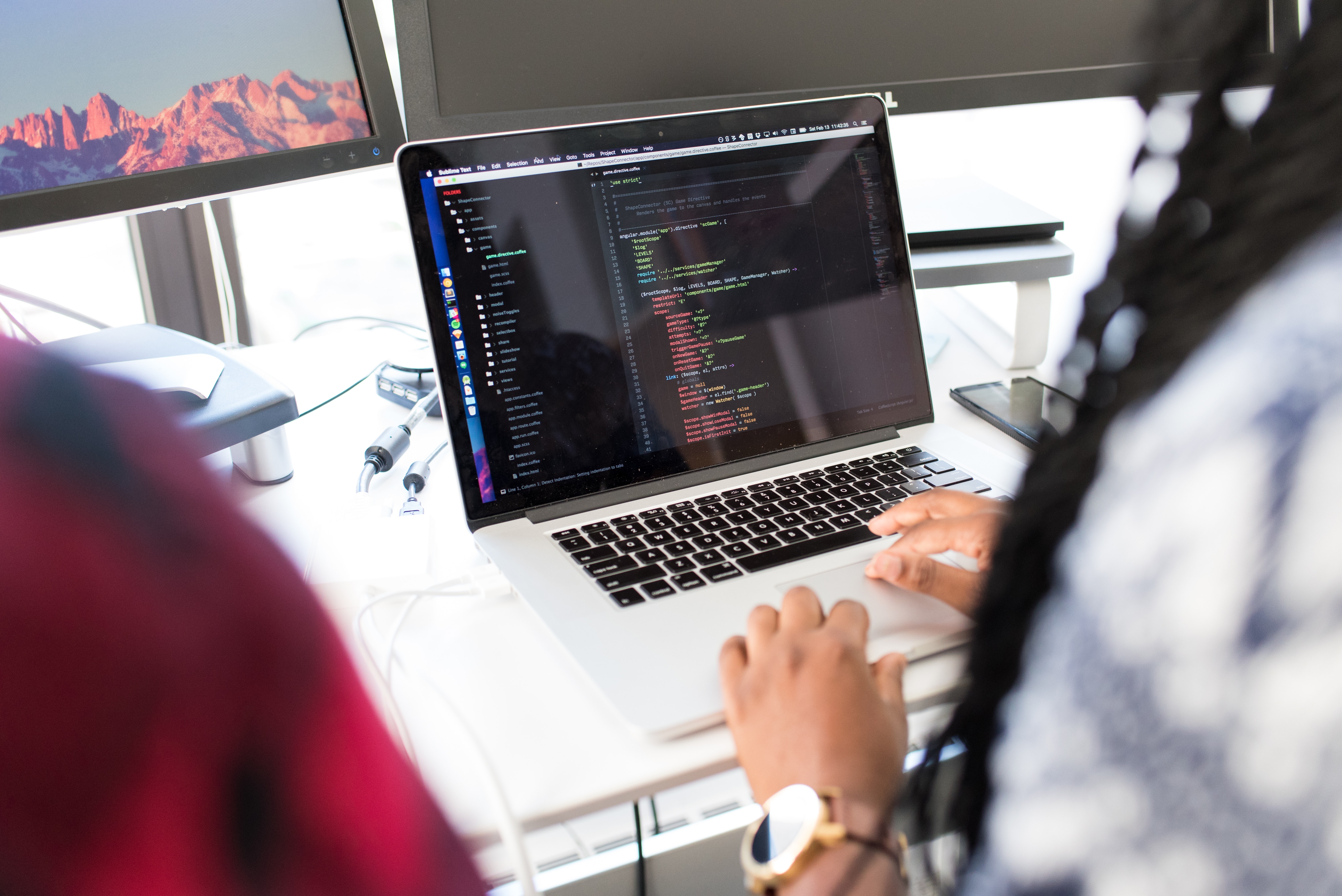
A map is a collection of elements where each element is stored as a Key, value pair. It remembers the original insertion order of the keys. A key in the Map
may only occur once; it is unique in the Map
's collection. Iteration happens in insertion order, which corresponds to the order in which each key-value pair was first inserted into the map by the set()
method (that is, there wasn't a key with the same value already in the map when set()
was called). It is similar to an object but with a few key differences. Some of them are explained below.
1. Accidental Keys: A Map
does not contain any keys by default. It only contains what is explicitly put into it whereas an object contains default keys that could collide with your keys if you're not careful.
- Key types: A map's key can be of any value but object's key must be either a String or a Symbol.
The correct usage for storing data in the Map is through the set(key, value)
method. Here's an example of how to create and use a map in JavaScript:
Map() constructor
Map()
is a higher-order array method that creates a new array by calling a provided function on each element of the original array. The map()
method does not modify the original array. If you want to modify the original array, you can use forEach()
instead.
The basic syntax for map()
is as follows:
array.map(callback(element[, index[, array]])[, thisArg])
Here's an example of how to use map()
to create a new array of squared numbers:
In this example, we first define an array of numbers. We then use the map()
method to create a new array of squared numbers. The map()
method takes a callback function as its argument, which is executed on each element of the original array. The result of the callback function is added to a new array, which is returned by the map()
method.
The callback function can take up to three arguments: the current element being processed, its index, and the original array. In the example above, we only need the first argument, which is the current element.
In addition to transforming data, map()
can also be used to filter data by returning a new array containing only elements that pass a certain condition. The returned array can also be of a different length than the original array. However, keep in mind that map()
creates a new array every time it is called, which can have performance implications when working with large arrays. As with any other array method, it's important to understand how map()
works and how to use it effectively to write clean and efficient code.
Subscribe to my newsletter
Read articles from Anushka Singh Bhardwaj directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by
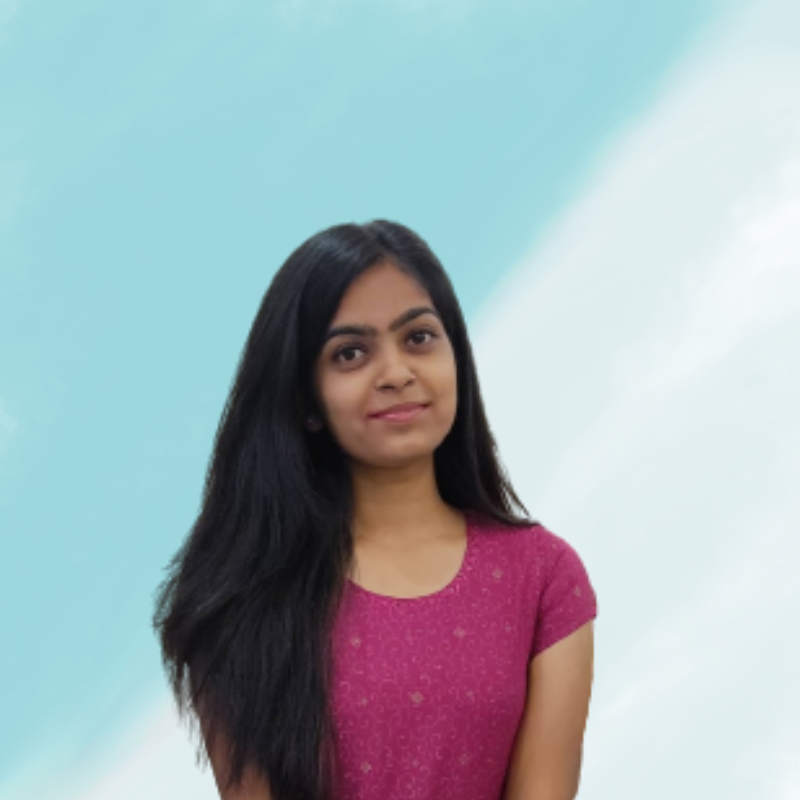
Anushka Singh Bhardwaj
Anushka Singh Bhardwaj
Frontend developer with expertise in HTML, CSS, JavaScript and experience in creating responsive, accessible and cross-browser compatible websites.