Python Tuples

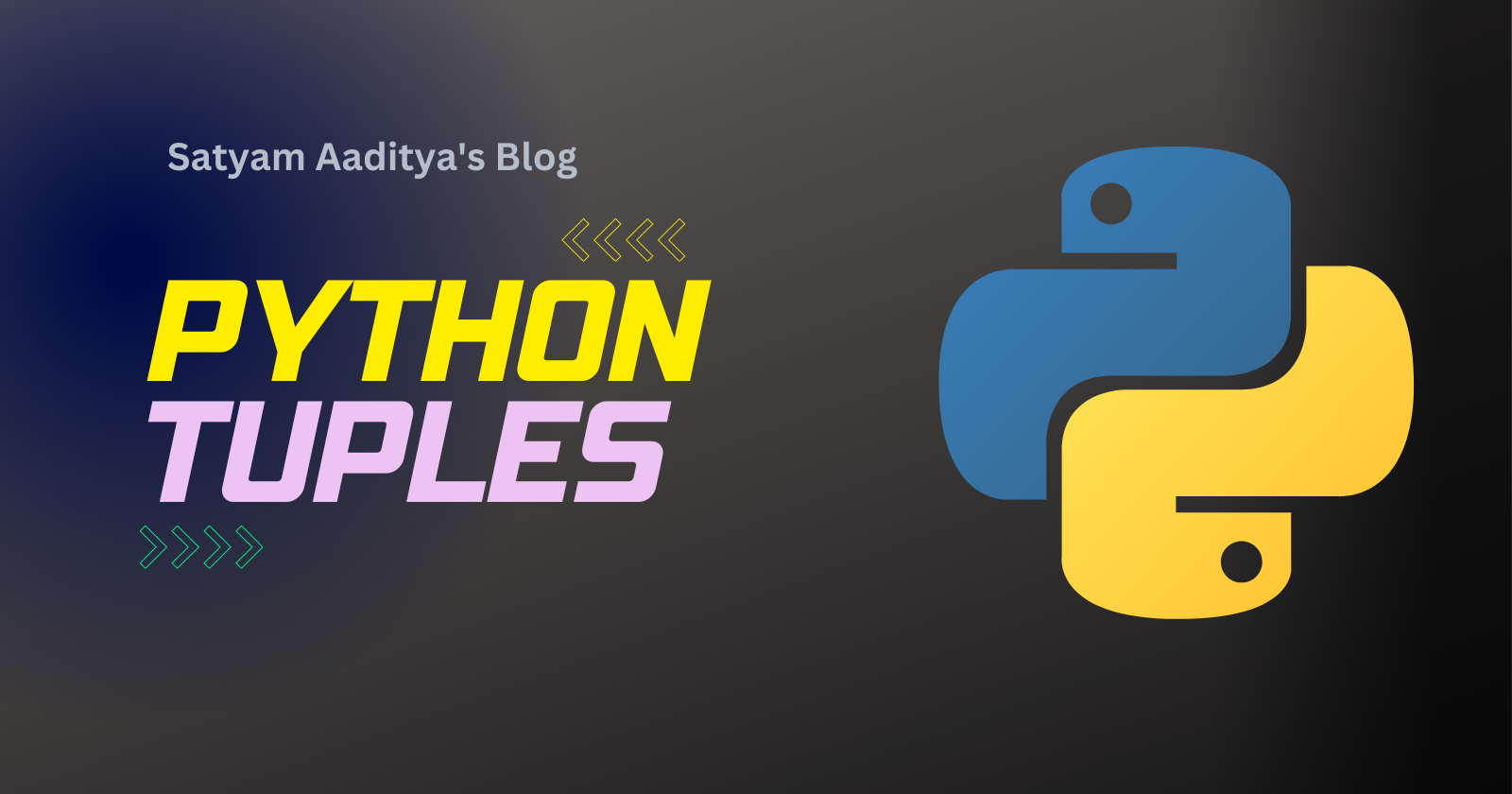
In Python, a tuple is a collection of immutable objects, which means that once a tuple is created, it cannot be modified. A tuple can contain objects of different data types, including integers, floats, strings, and other tuples.
Creating Tuples
To create a tuple, you can use parentheses () or the built-in tuple() function. Here are some examples:
# Creating a tuple using parentheses
my_tuple = (1, 2, 3, 'four')
# Creating a tuple using the tuple() function
another_tuple = tuple(['apple', 'banana', 'orange'])
Accessing Tuple Elements
You can access elements of a tuple using the indexing operator []. The index of the first element in a tuple is 0, and the index of the last element is len(my_tuple) - 1. Here's an example:
my_tuple = (1, 2, 3, 'four')
print(my_tuple[0]) # Output: 1
print(my_tuple[-1]) # Output: 'four'
Tuple Slicing
You can also use slicing to access a range of elements in a tuple. The syntax for slicing is similar to that for lists. Here's an example:
my_tuple = (1, 2, 3, 'four', 'five', 'six')
print(my_tuple[1:4]) # Output: (2, 3, 'four')
print(my_tuple[:3]) # Output: (1, 2, 3)
print(my_tuple[3:]) # Output: ('four', 'five', 'six')
Tuple Methods
Although tuples are immutable, they have a few built-in methods that you can use to manipulate them.
1. count(): Returns the number of times a specified element appears in a tuple.
my_tuple = (1, 2, 3, 2, 4, 2, 'five')
print(my_tuple.count(2)) # Output: 3
2. index(): Returns the index of the first occurrence of a specified element in a tuple.
my_tuple = (1, 2, 3, 2, 4, 2, 'five')
print(my_tuple.index(4)) # Output: 4
Advantages of Tuples
Tuples are immutable, so they cannot be modified accidentally, making them a safe option when you need to store data that shouldn't be changed.
Tuples are faster than lists in terms of performance, especially when it comes to indexing and iterating through elements.
Tuples can be used as keys in dictionaries, whereas lists cannot, since keys must be immutable.
Conclusion
In conclusion, tuples are an important data type in Python that can be used to store a collection of immutable objects. They have many advantages over lists, including better performance and immutability. Understanding how to create and manipulate tuples will be valuable when working with complex data structures in Python.
Subscribe to my newsletter
Read articles from Satyam Aaditya directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by
