Simple Steps for Including Custom Fields in Wasp Google Auth

Table of contents
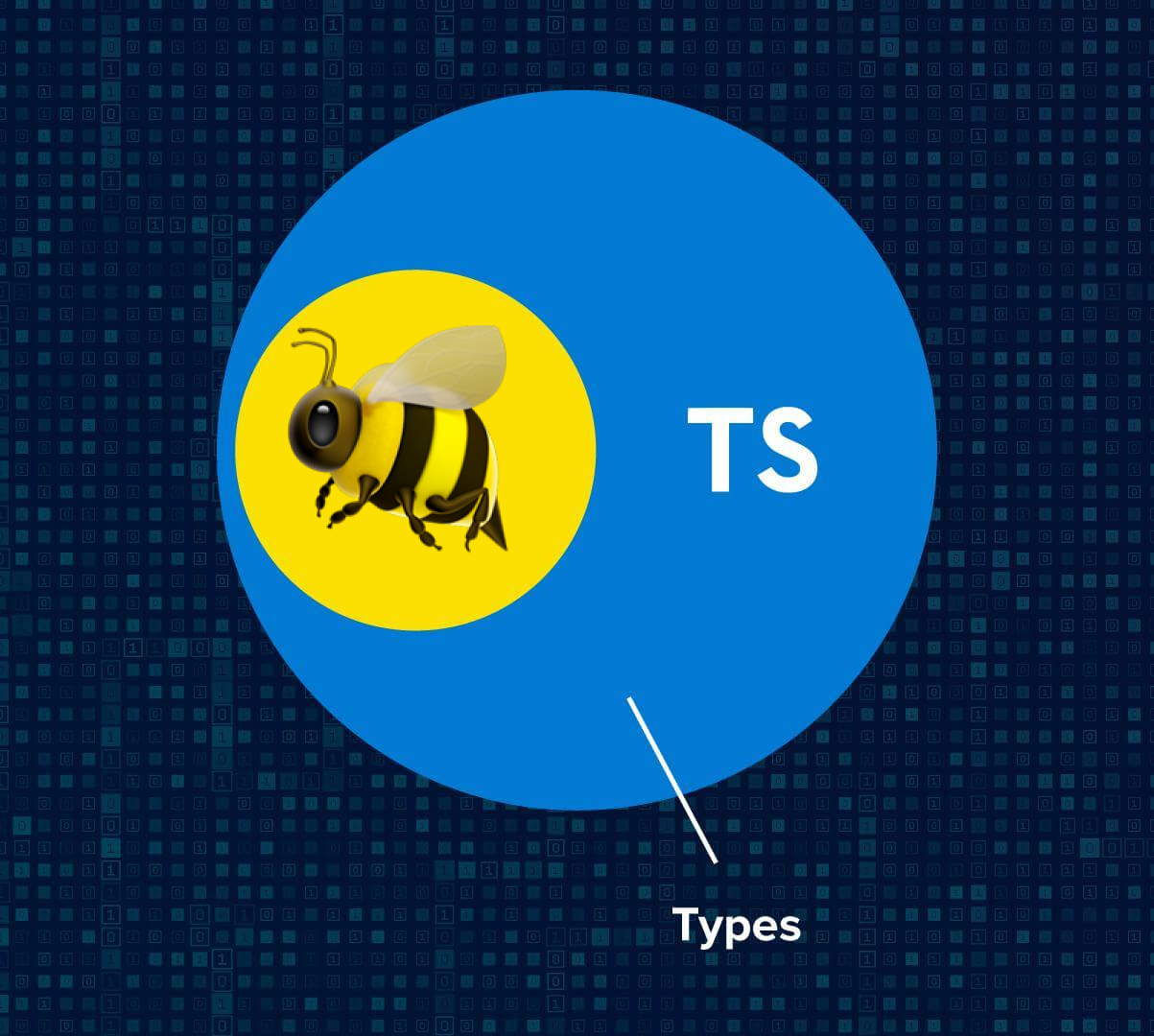
The problem statement
When using Google OAuth in Wasp project, you might notice that behind the scenes, whenever you are logging in the user, creates the user for you, if it doesn't exist. It does so with very minimal user fields, but what if you need additional user information, such as profile image
or full name
?
Let's take care of that and learn a thing or two about Wasp lang.
The solution
- Make sure your database has the correct columns, in the User table. In my case, I added 2 more properties to the User entity, in my
main.wasp
file:
entity User {=psl
id Int @id @default(autoincrement())
email String? @unique
password String?
isEmailVerified Boolean @default(false)
emailVerificationSentAt DateTime?
passwordResetSentAt DateTime?
profileImage String?
fullName String?
stripeId String?
checkoutSessionId String?
hasPaid Boolean @default(false)
sendEmail Boolean @default(false)
datePaid DateTime?
credits Int @default(3)
relatedObject RelatedObject[]
externalAuthAssociations SocialLogin[]
psl=}
Once added, run wasp db migrate-dev
so Wasp takes care of all the db migrations, and relaunch your server.
- In
main.wasp
add a new dependency@types/passport-google-oauth20
. At the time of writing this article, the actual version is2.0.11
but go ahead and install the latest one.
("@types/passport-google-oauth20", "2.0.11")
The reason why you install @types/passport-google-oauth20
is because Wasp is using passport
to handle authentication strategy etc behind the scenes, so that's exactly the types that are used in the backend and that you can expect to be returned.
Create a file under
src/server/auth/google.ts
Add new function
getUserFields
alongside with type import:
import { Profile } from "passport-google-oauth20";
export async function getUserFields(
_: unknown,
args: { profile: Profile }
) {
const userFields = {
email: args.profile._json.email,
profileImage: args.profile._json.picture,
fullName: args.profile._json.name,
};
return userFields;
}
- Declare your
getUserFields
function inmain.wasp
file, by addinggetUserFieldsFn
property toauth.methods.google
object, like so:
auth: {
userEntity: User,
externalAuthEntity: SocialLogin,
methods: {
google: {
getUserFieldsFn: import { getUserFields } from "@server/auth/google.js", // <-- add `getUserFieldsFn` property
configFn: import { config } from "@server/auth/google.js",
},
},
onAuthFailedRedirectTo: "/",
},
Function getUserFields
is a special function, that Wasp is using to retrieve any user fields that will be added to the database. The second argument of this function is to receive an object, that contains profile
of type Profile
. This is what is being "passed" to you by Wasp , so that you can "cherry-pick" what you want to be saved into your User record when one will be created.
Whatever object you will return from this function, this is what will be stored in the User table, under the new row. As you can see, I am retrieving profileImage
and fullName
properties from args.profile._json.
object.
You can read more about getUserFields
in the linked article, but it's only covering JavaScript
file, not TypeScript, so hoping this article helps you to annotate. your codebase better, until Wasp provides you with a type export by itself.
Follow me here on ๐ Hashnode: https://blog.oleggulevskyy.dev/
Follow me on ๐ฆ Twitter: https://twitter.com/preacher_rourke
I am sharing all the cool stuff I find, daily.
Subscribe to my newsletter
Read articles from Oleg Gulevskyy directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by

Oleg Gulevskyy
Oleg Gulevskyy
Full stack aficionado, passionate about making tech simpler, faster, and enjoyable. Sharing the love with open source and spreading the knowledge ! ๐๐๐๐