Deep Clone(Javascript structuredClone)
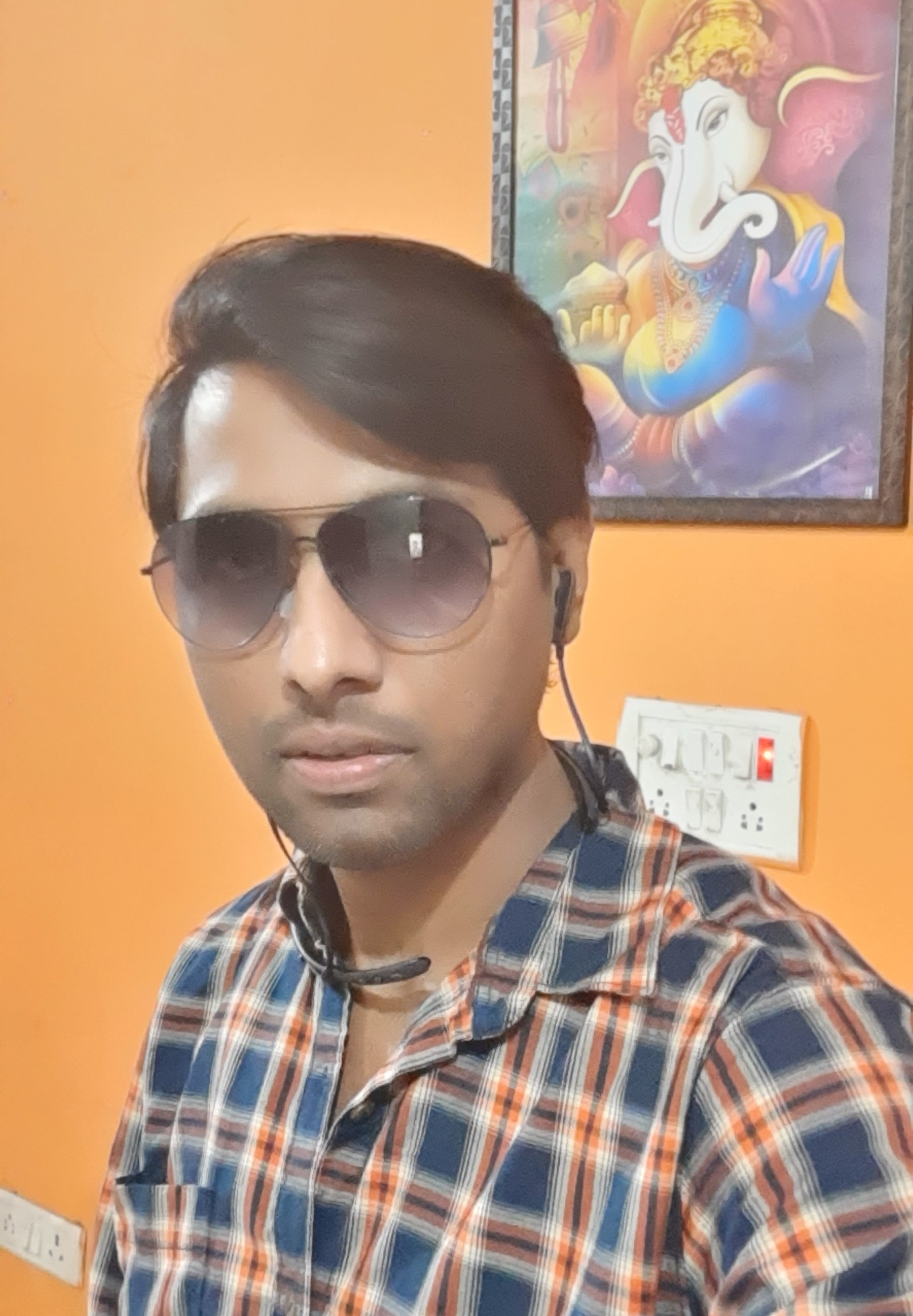
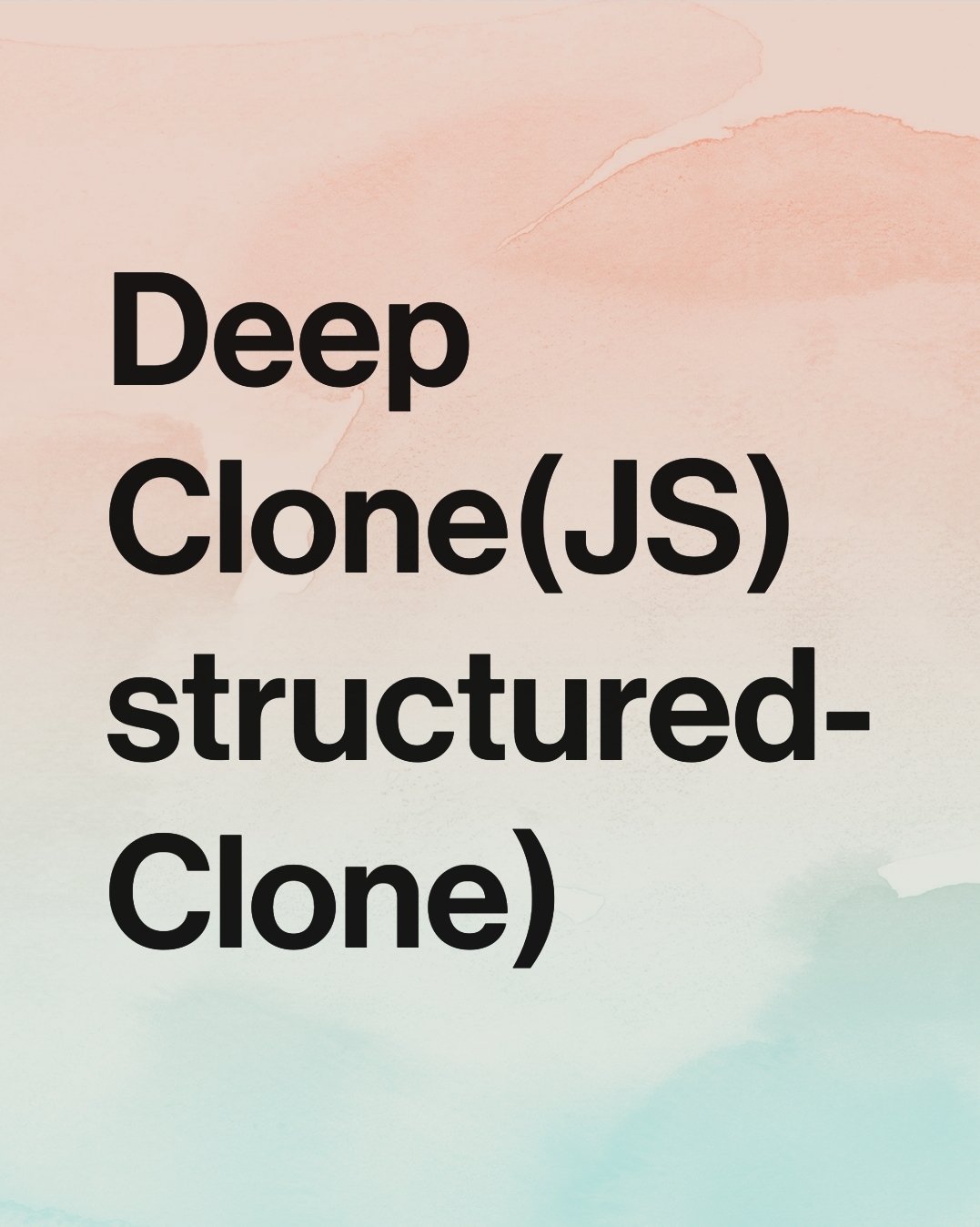
Problem- Deep Clone nested objects in javascript
Solution 1
JSON. parse(JSON. stringify())
Solution 2 - using any third-party lib like (lodash-_-clone deep-method)
_.cloneDeep( value )
Solution 3 - Write your own function/Code
Solution 4 โ The structured clone algorithm(Very Popular)
The structured clone algorithm copies complex JavaScript objects. It is used internally when invoking structuredClone()
The returned value is a deep copy of the original value.
//with structuredClone
const person = {
name: 'Anil',
skills: ['FullStack'],
joiningDate: new Date('2023-10-25')
}
const person2 = structuredClone(person);
person2.name = 'Anil Verma';
console.log(person.name); //Anil
console.log(person2.name); //Anil Verma
//With spread operator
const person = {
name: 'Anil',
skills: ['FullStack'],
joiningDate: new Date('2023-10-25')
}
const person2 = {
...person
}
Note- skills is an array altering person2.skills will alter person.skills
//With stringify
const person = {
name: 'Anil',
skills: ['FullStack'],
joiningDate: new Date('2023-10-25')
}
person2 = JSON.parse(JSON.stringify(person))
Note- joiningDate is a Date object it will become string value
Things that donโt work with the structured clone
Function objects cannot be duplicated by the structured clone algorithm; attempting to throws a DataCloneError exception.
Cloning DOM nodes likewise throws a DataCloneError exception.
Certain object properties are not preserved:
The lastIndex property of RegExp objects is not preserved.
Property descriptors, setters, getters, and similar metadata-like features are not duplicated.
The prototype chain is not walked or duplicated.
Source: MDN
Happy learningโฆ. ๐๐๐๐
Subscribe to my newsletter
Read articles from Anil Verma directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by
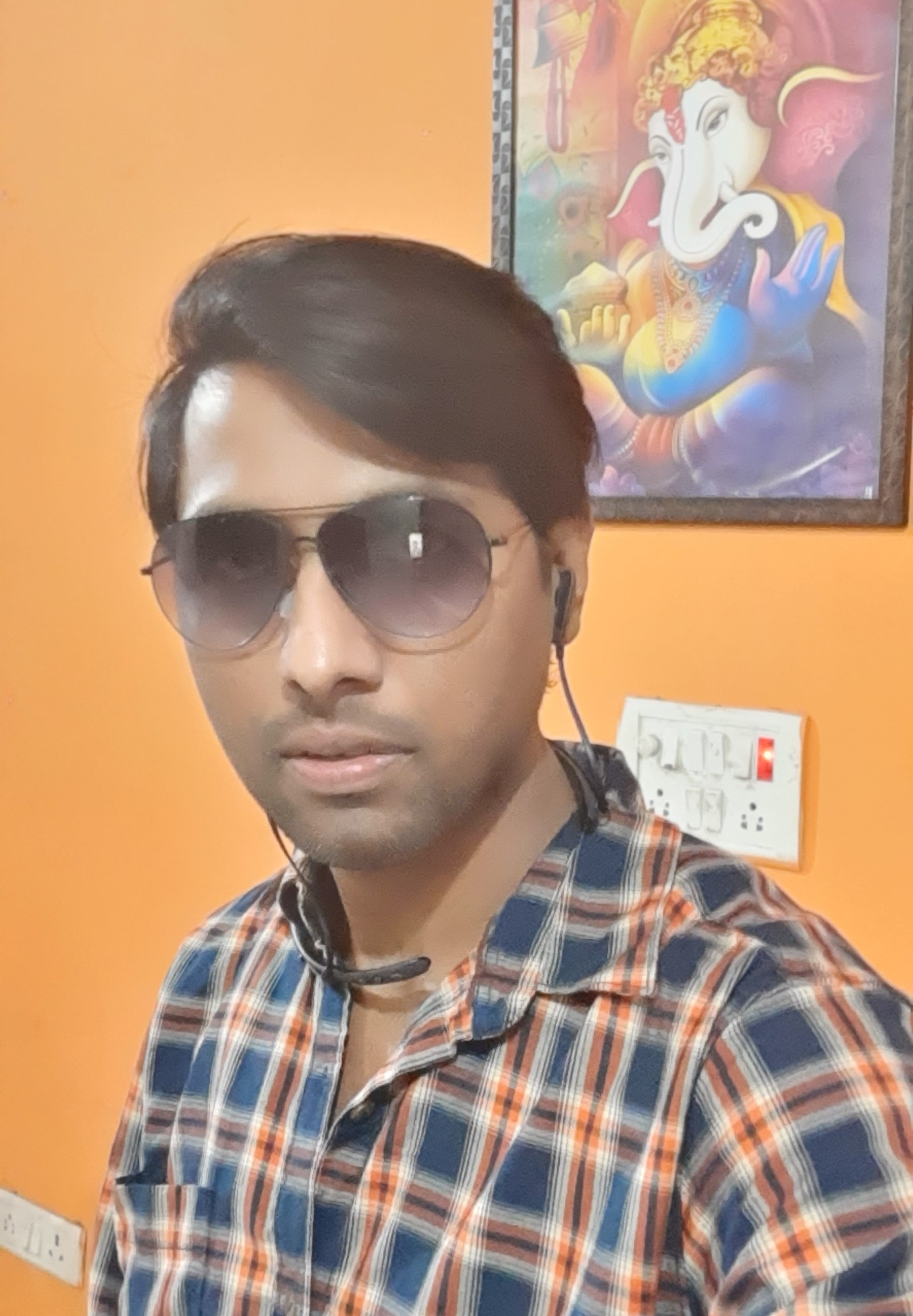
Anil Verma
Anil Verma
Hi there ๐, I am Anil Verma I am a passionate Full Stack Web Developer who is fascinated by complex engineering problems.