Exception handling in JavaScript
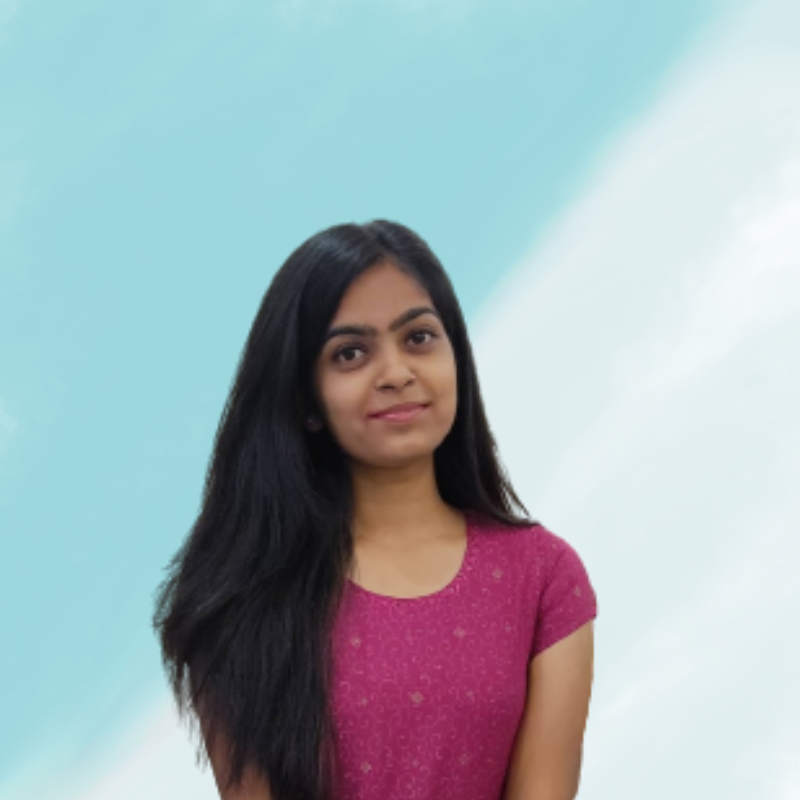
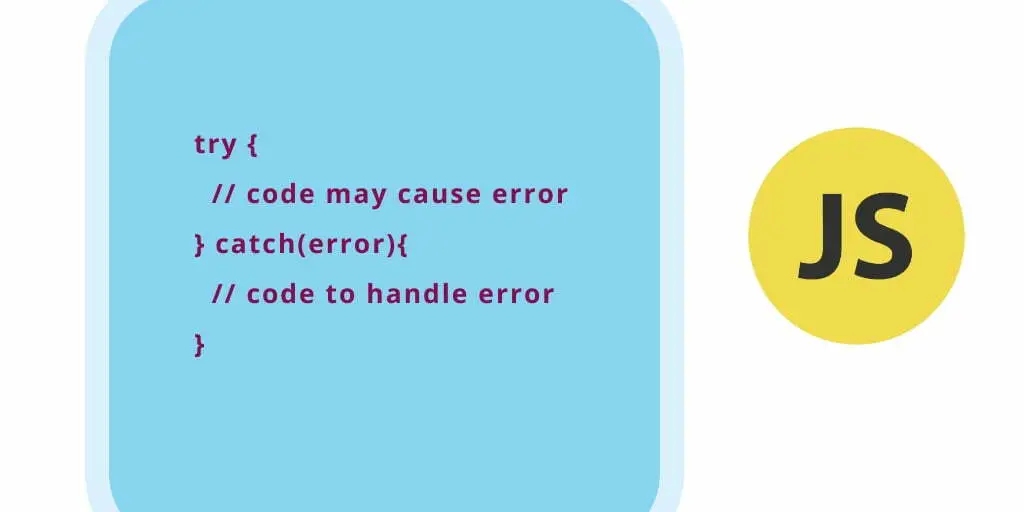
There are two main types of errors in JavaScript:
Syntax errors: occur when the code syntax is not proper and JavaScript interpreter can't understand it. This error can't be handled with exception handling.
Runtime errors: occur while the code is executing. For ex, accessing an undefined variable or when a function is not found. This error can be handled using exception handling.
Introduction to try-catch
The try statement is used for implementing exception handling. It is a piece of code that needs to be tested during the execution of code. The block of code is checked if it has any errors or not. If any errors are encountered, then the try{} statement passes it to the catch{} statement block. Once the control is handed over to the catch block the code block under catch{} will be executed. The syntax of a try
statement is as follows:
The catch statement defines a block of code that gets executed when any errors are encountered within the try block. The catch block gets executed only when there is any error present in the try block and the error needs to be addressed. Otherwise, the catch block gets skipped. The catch block gets executed only after the execution of the try block.
Flow chart
Use of try-catch in real-world application
In this example, the try block contains code that may throw an error, such as calling a function that doesn't exist (someFunction()). If an error is thrown within the try block, the code in the corresponding catch block will be executed.
In the catch block, we can handle the error in whatever way makes sense for our application. In this case, we're simply logging an error message to the console with console.error().
Note that the catch block only runs if an error is thrown in the corresponding try block. If no error is thrown, the catch block is skipped.
Also, the error parameter in the catch block is an object that contains information about the error that was thrown. You can access the error message with error.message. Other properties of the error object include name (the name of the error type), stack (a stack trace of the error), and more.
try-catch-finally
In addition to the catch statement, we can also use the finally statement, which defines a block of code that will be executed regardless of whether an exception was thrown or not. This can be used to perform other actions after the try-and-catch statements have been executed.
Here's an example of using the try-catch-finally statement in JavaScript:
In the above example, the divide function attempts to divide num1 by num2. If an error occurs during the division operation (such as dividing by zero), the catch block is executed, logging an error message to the console and returning 0 as the result. The finally block is also executed, regardless of whether an error was thrown or not, and logs a message to the console indicating that the division operation is completed.
In the first example where the divide function is called with valid inputs, the output is 5. In the second example where it's called with invalid input, the output is 0 and an error message is logged to the console, indicating that the division by zero caused the error. The finally block is still executed in both cases, logging a message indicating that the operation is complete.
Conclusion
Exception handling is an important aspect of writing robust and reliable JavaScript code. By using try-catch-finally statements, you can catch and handle exceptions that may occur during the execution of your code.
In this article, we covered the basics of exception handling in JavaScript, including how to catch and handle different types of exceptions using the try-catch-finally statement. We also provided some real-life examples of how try-catch statements can be used to handle errors in various scenarios, such as handling user input errors, network errors, and unexpected errors.
By following these best practices and using try-catch statements effectively, you can write more reliable and robust JavaScript code that can handle unexpected errors and ensure that your application remains stable and user-friendly.
See you all in the next one!! Byee :)
Subscribe to my newsletter
Read articles from Anushka Singh Bhardwaj directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by
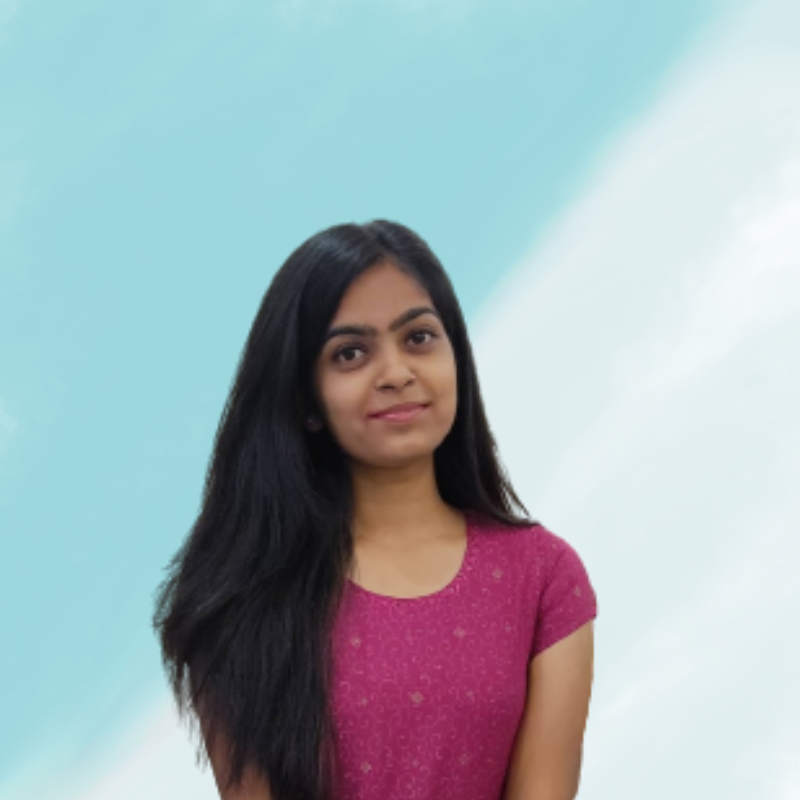
Anushka Singh Bhardwaj
Anushka Singh Bhardwaj
Frontend developer with expertise in HTML, CSS, JavaScript and experience in creating responsive, accessible and cross-browser compatible websites.