Conditional Statement. if, else, and else if and switch.

Table of contents
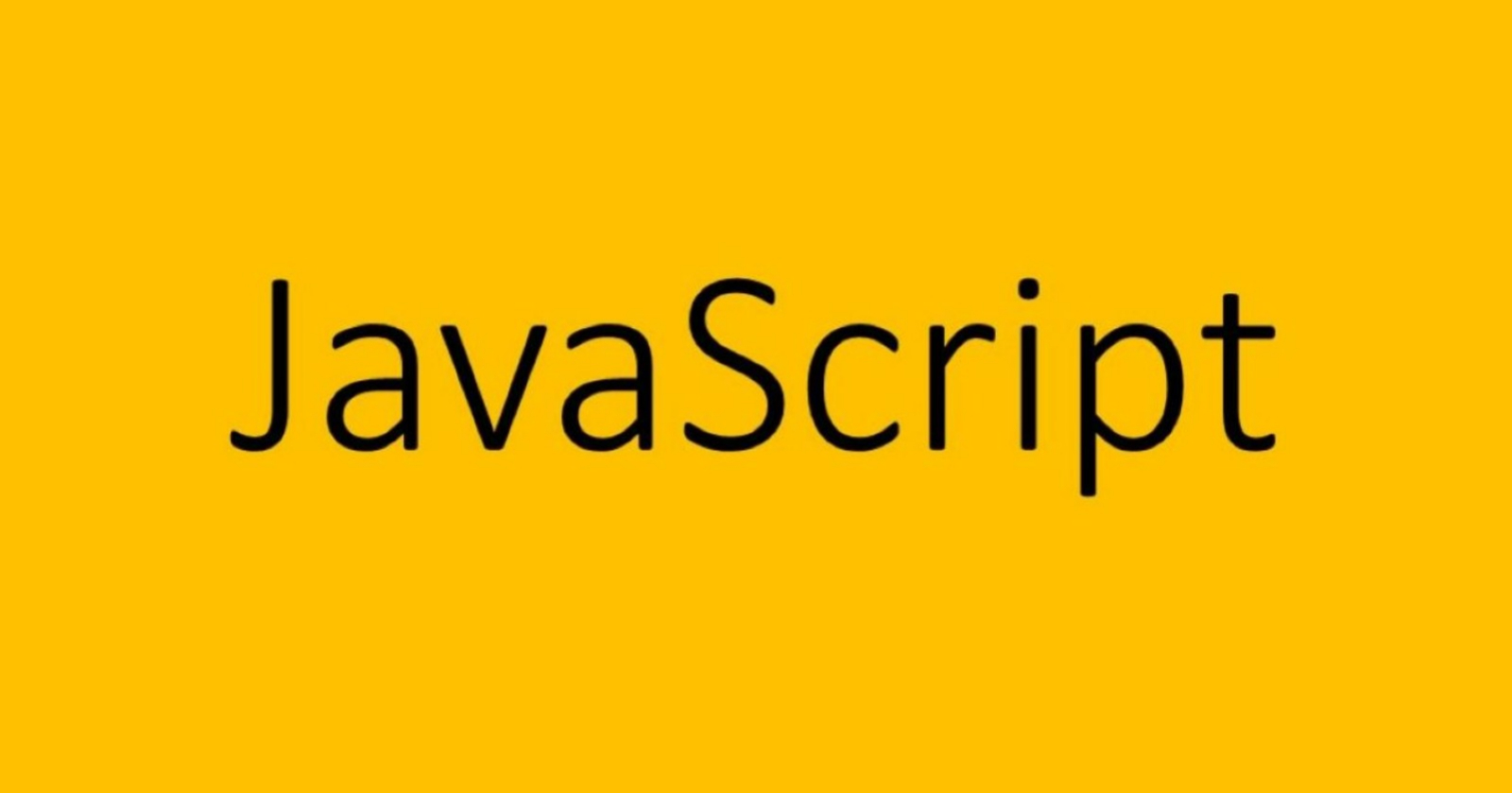
Condition statements are used to perform different actions based on different conditions. When you write code you want to perform different actions for different missions. In JavaScript, a conditional statement is a programming structure that allows you to make decisions based on certain conditions.
The most common type of conditional statement is the if
statement, which checks if a certain condition is true or false, and then executes some code if the condition is true.
if statement
let age = 15;
if (age >= 18) {
console.log("You are old enough to vote!");
} else {
console.log("You are not old enough to vote yet.");
}
we're using an if
statement to check if the value of the age
variable is greater than or equal to 18. If it is, the program will execute the code inside the curly braces following the if
statement, which will print "You are old enough to vote!" to the console. If the condition is false, the program will execute the code inside the curly braces following the else
statement, which will print "You are not old enough to vote yet." to the console.
else statement
let age = 15;
if (age >= 18) {
console.log("You are old enough to vote!");
} else {
console.log("You are not old enough to vote yet.");
}
we are checking whether the value of the variable age
is greater than or equal to 18 using an if
statement.
If the condition is true, the program will execute the code block inside the curly braces immediately following the if
statement, which in this case logs "You are old enough to vote!" to the console.
If the condition is false, however, the program will execute the code block inside the curly braces following the else
keyword, which in this case logs "You are not old enough to vote yet." to the console.
The value of age
is 15 and not greater than or equal to 18, the program executes the code block following the else
keyword and logs "You are not old enough to vote yet." so the else statement executes.
else if statement
The else if statement chain multiple conditions together.
let grade = 85;
if (grade >= 90) {
console.log("You got an A!");
} else if (grade >= 80) {
console.log("You got a B!");
} else if (grade >= 70) {
console.log("You got a C!");
} else if (grade >= 60) {
console.log("You got a D!");
} else {
console.log("You failed the class.");
}
we're using an if
statement to check the value of the grade
variable. If it's greater than or equal to 90, the program will log "You got an A!" to the console. If it's not, the program will move on to the next condition, which is an else-if
statement that checks if the grade is greater than or equal to 80. If this condition is true, the program will log "You got a B!" to the console.
If this condition is also false, the program will move on to the next else if
statement, which checks if the grade is greater than or equal to 70, and so on.
If none of the conditions are true, the program will execute the code block inside the else
statement and log "You failed the class." to the console.
In this example, since the value of the grade
is 85, the program executes the code block following the else if
statement that checks if the grade is greater than or equal to 80, and logs "You got a B!" to the console.
So let's move to the switch statement.
Switch statement
The switch
statement is useful when you have multiple conditions to test, and can be a more efficient and organized way to handle multiple conditions than a series of if
/else if
statements.
Example of the switch statement.
let country = "India";
switch (country) {
case "USA":
console.log("Welcome to Usa!");
break;
case "UK":
console.log("Welcome to Uk.");
break;
case "India":
console.log("Welcome to India!");
break;
case "Japan":
console.log("Welcome to Japan!");
break;
case "China":
console.log("Welcome to China!");
break;
default:
console.log("Ok Welcome to Earth!");
break;
}
we're using a switch
statement to check the value of the country
variable. The case
statements provide different values to test against, and the code block following each the case
statement is executed if the value of country
matches that case.
If none of the case
statements match the value of the country
, the default
case is executed. In this example, the default case logs "Ok Welcome to Earth!" to the console.
So in this example, since the value of a country
is "India", the program executes the code block following the first case
statement, which logs "Welcome to India" to the console.
Thank you
Subscribe to my newsletter
Read articles from Aditya Pandey directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by
