CodeWars (5 kyu) Pete, the baker - Javascript
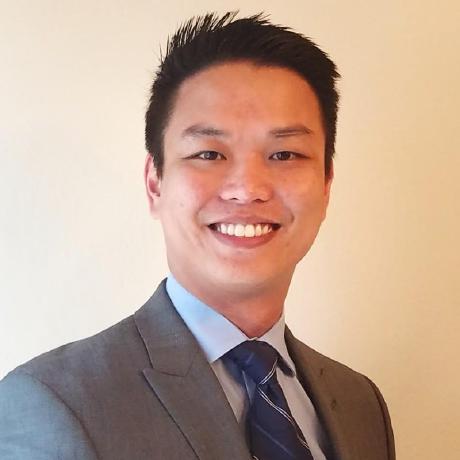
Description:
Pete likes to bake some cakes. He has some recipes and ingredients. Unfortunately he is not good in maths. Can you help him to find out, how many cakes he could bake considering his recipes?
Write a function cakes()
, which takes the recipe (object) and the available ingredients (also an object) and returns the maximum number of cakes Pete can bake (integer). For simplicity there are no units for the amounts (e.g. 1 lb of flour or 200 g of sugar are simply 1 or 200). Ingredients that are not present in the objects, can be considered as 0.
Examples:
// must return 2
cakes({flour: 500, sugar: 200, eggs: 1}, {flour: 1200, sugar: 1200, eggs: 5, milk: 200});
// must return 0
cakes({apples: 3, flour: 300, sugar: 150, milk: 100, oil: 100}, {sugar: 500, flour: 2000, milk: 2000});
Sample Tests
const {assert} = require('chai');
describe('description example', function() {
it('pass example tests', function() {
let recipe = {flour: 500, sugar: 200, eggs: 1};
let available = {flour: 1200, sugar: 1200, eggs: 5, milk: 200};
assert.equal(cakes(recipe, available), 2);
recipe = {apples: 3, flour: 300, sugar: 150, milk: 100, oil: 100};
available = {sugar: 500, flour: 2000, milk: 2000};
assert.equal(cakes(recipe, available), 0);
});
});
Solution
To solve this kata, we'll be taking in recipe,
which contains the required ingredients and their respective amounts stored within an object. Additionally, we have another object available
that tells us what ingredients we have on hand.
We'll need to see what ingredients the recipe
calls for and figure out how many cakes we can make with the ingredients we have in available
. If recipe
calls for an ingredient that does not exist in available
, we'll need to return 0.
First, let's work through the pseudocode:
function cakes(recipe, available) {
// Iterate over recipe object to get each ingredient
// Confirm we have the ingredient available
// Calculate the maximum amount we can make per each ingredient
// Return the smallest amount we can make
}
Now that we have an idea of how to proceed, let's start working through the coding solution.
function cakes(recipe, available) {
// Need a variable to keep track of how many possible cakes can be made per ingredient
let counterArr = []
// Iterate over `recipe` object to get each ingredient
for (let ingredient in recipe){
// Confirm we have the ingredient available
// Calculate the maximum amount we can make per each ingredient
available[ingredient] ?
counterArr.push(Math.floor(available[ingredient] / recipe[ingredient])) : counterArr.push(0)
}
// Return the smallest amount we can make
return Math.min(...counterArr)
}
So there are a few pieces in the code we should make note of:
We'll need one additional variable
counterArr
to keep track of how many possible cakes we can make per ingredientWe'll be iterating over the
recipe
object by usingfor...in
We check if we have the
recipe's
required ingredientsavailable
If available, we push the maximum, integer amount of cakes we can make per ingredient
If not available, then we can't make any cakes and push
0
We return the smallest number in our
counterArr
by combiningMath.min()
with the spread operator...
Subscribe to my newsletter
Read articles from Nam Nguyen directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by
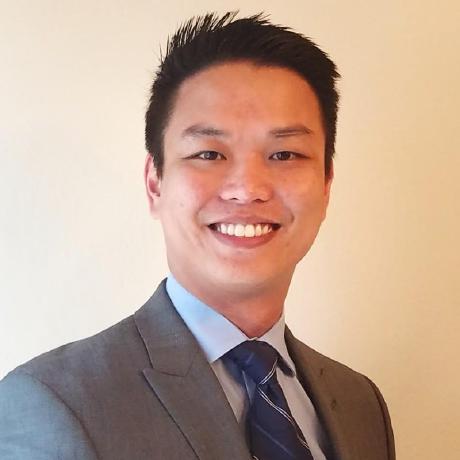
Nam Nguyen
Nam Nguyen
A passionate software engineer providing elegant, innovative, and accessible solutions. When I’m not click-clacking away at my keyboard, you’ll probably find me lost in a book, trying a new recipe/restaurant, or having a blast with my beautiful wife and precocious daughter. I have 10+ years of IT experience and look forward to discussing ways we can join hands for our next adventure!