While Loops In Java

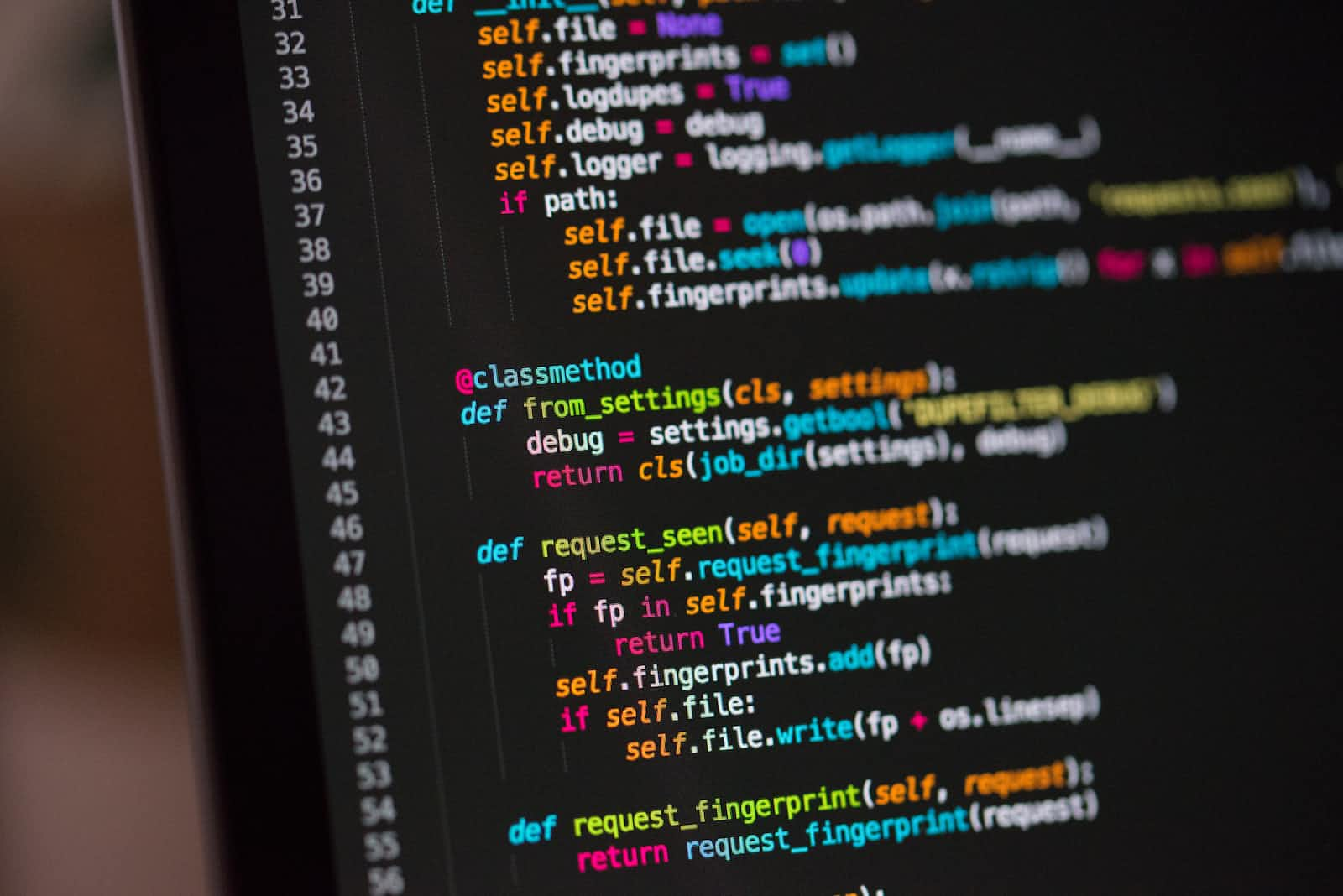
So while loop is used to repeat a specific code again and again and again.
While loop consists of a condition. And while the loop will keep repeating itself until or unless the condition written in parenthesis is true.
Like the condition can be Example -> 3>2 (It is an infinite condition.)
Syntax :
while( condition ){
// Your Block Of Code
}
Example 1 :
Write a code to print "Hello world ".
public class PrintHelloWorld100Times {
public static void main(String[] args) {
int count = 1;
while(true) {
System.out.println(count+". Hello World");
count++;
}
}
}
Example 2 :
Write a code to print numbers from 1 to 10.
public class PrintNumberFrom1to10 {
public static void main(String[] args) {
int num = 1;
while(num < 11) {
System.out.println(num);
num++;
}
}
}
Example 3 :
Write a code to print numbers from 1 to n.
So, this code requires input from the user. For this, we will use the Scanner class.
This class is used to give user input.
Scanner Class Example :
// Scanner Class Example
Scanner sc = new Scanner(System.in);
Now the real code.
public class PrintFrom1toN {
public static void main(String[] args) {
Scanner sc = new Scanner(System.in); // Scanner Class
System.out.println("Enter the last number ");
int fstnum = 1 , lstnum = sc.nextInt();
// sc.nextInt(); For inputing the last number.
while(fstnum <= lstnum) {
System.out.println(fstnum);
fstnum++; // Unary Operator
}
}
}
Example 4 :
Write a Code for Sum of n Natural Numbers.
So the key here is to remember what is the difference between natural numbers and whole numbers.
So the whole numbers are the number that starts from 0, 1, 2, 3, ............ to infinite.
So the natural numbers are the number that starts from 1, 2, 3, 4, ............ to infinite.
public class SumofFirstnNaturalNumbers {
public static void main(String[] args) {
int num = 1,lstnum,sum = 0;
Scanner sc = new Scanner(System.in);
lstnum = sc.nextInt();
while(num<=lstnum) { // num is also called iterator
sum += num; // Assignment Operator
num++; // Unary Operator
}
System.out.println("Sum is : "+sum);
}
}
More Facts or Insights about While Infinite Loops
An infinite loop is a loop that is running infinite times on your computer. It usually happens when we have a never-ending true condition and it will just run and runs.
So, this is the end of while loops in Java.
I hope you liked it.
Subscribe to my newsletter
Read articles from Aditya Deshwal directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by
