How to use a webcam in the Nextjs application
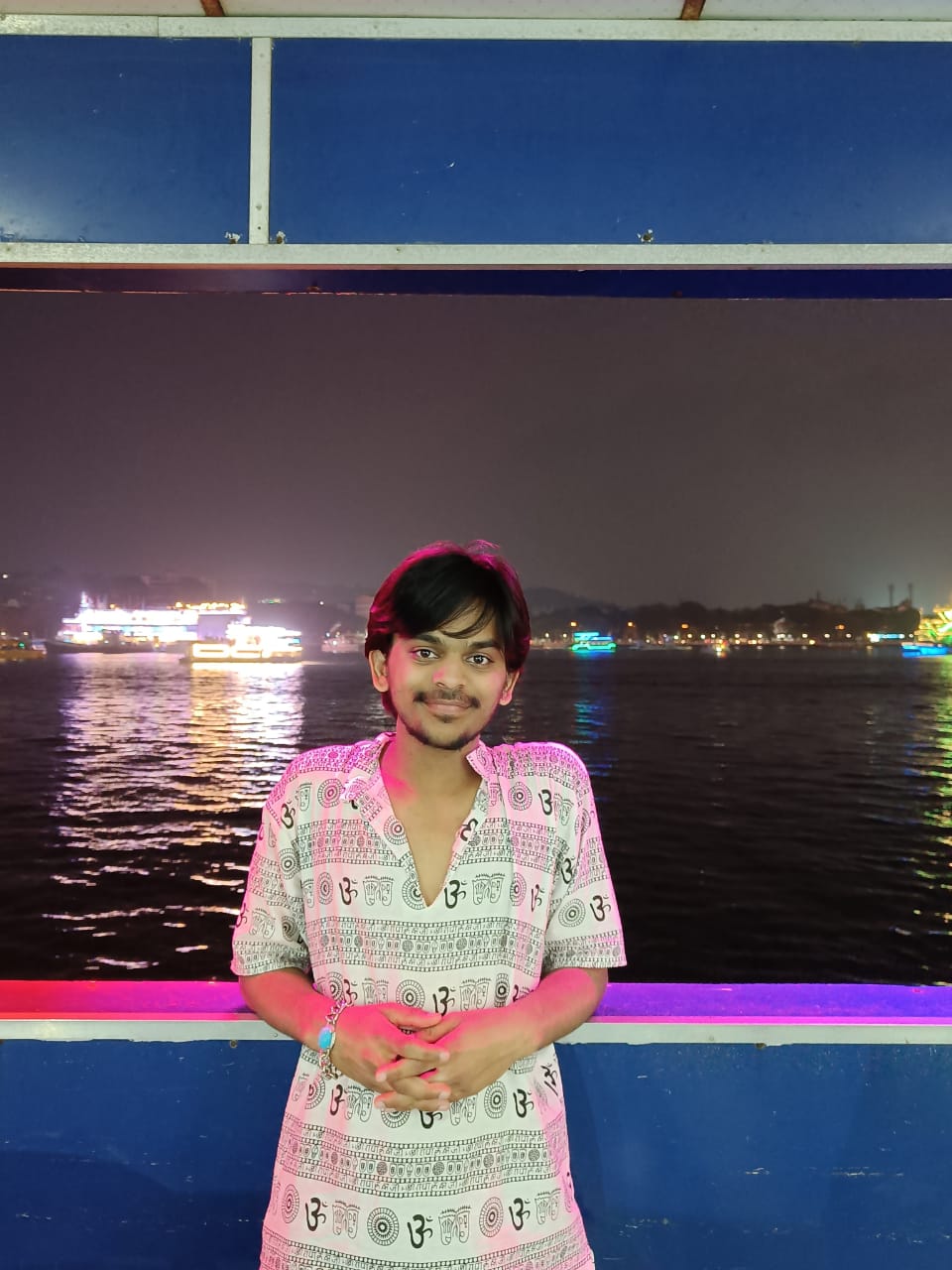
hey folks, In this blog I wanted to share my latest experience of building a video streaming platform for my client.
if you are looking to build a video streaming platform you would need to use a webcam for your user and here is how you can do this.
In your React Component, import 3 hooks.
import React, { useRef, useState, useEffect } from 'react';
In your React Component, initialize two variables.
import React, { useRef, useState, useEffect } from 'react'; const CamScreen = () => { const videoRef = useRef<any>(null); const [mediaStream, setMediaStream] = useState<MediaStream | null>(null); const sendVideoData = (data: string) => { socket.emit("videoData", data); }; return ( <div>continue reading it....</div> ) } export default CamScreen;
Now first, we would need to ask permission from the user to open the webcam and for this, we are going to use javascript MediaDevices.
a basic idea,
The
MediaDevices
.getUserMedia()
method prompts the user for permission to use a media input which produces aMediaStream
with tracks containing the requested types of media.you can do like this.
import React, { useRef, useState, useEffect } from 'react'; const CamScreen = () => { const videoRef = useRef<any>(null); const [mediaStream, setMediaStream] = useState<MediaStream | null>(null); const sendVideoData = (data: string) => { socket.emit("videoData", data); }; useEffect(() => { const enableVideoStream = async () => { try { const stream = await navigator.mediaDevices.getUserMedia({ video: true }); setMediaStream(stream); } catch (error) { console.error('Error accessing webcam', error); } }; enableVideoStream(); }, []); return ( <div>continue reading it....</div> ) } export default CamScreen;
Next, we would need to update the variables and store the media stream on it,
import React, { useRef, useState, useEffect } from 'react'; const CamScreen = () => { const videoRef = useRef<any>(null); const [mediaStream, setMediaStream] = useState<MediaStream | null>(null); const sendVideoData = (data: string) => { socket.emit("videoData", data); }; useEffect(() => { const enableVideoStream = async () => { try { const stream = await navigator.mediaDevices.getUserMedia({ video: true }); setMediaStream(stream); } catch (error) { console.error('Error accessing webcam', error); } }; enableVideoStream(); }, []); useEffect(() => { if (videoRef.current && mediaStream) { videoRef.current.srcObject = mediaStream; } }, [videoRef, mediaStream]); useEffect(() => { return () => { if (mediaStream) { mediaStream.getTracks().forEach((track) => { track.stop(); }); } }; }, [mediaStream]); return ( <div>continue reading it....</div> ) } export default CamScreen;
and finally, you can present the video on screen,
import React, { useRef, useState, useEffect } from 'react'; const CamScreen = () => { const videoRef = useRef<any>(null); const [mediaStream, setMediaStream] = useState<MediaStream | null>(null); const sendVideoData = (data: string) => { socket.emit("videoData", data); }; useEffect(() => { const enableVideoStream = async () => { try { const stream = await navigator.mediaDevices.getUserMedia({ video: true }); setMediaStream(stream); } catch (error) { console.error('Error accessing webcam', error); } }; enableVideoStream(); }, []); useEffect(() => { if (videoRef.current && mediaStream) { videoRef.current.srcObject = mediaStream; } }, [videoRef, mediaStream]); useEffect(() => { return () => { if (mediaStream) { mediaStream.getTracks().forEach((track) => { track.stop(); }); } }; }, [mediaStream]); return ( <div> <video ref={videoRef} autoPlay={true} /> </div> ) } export default CamScreen;
Thanks for Reading.
Subscribe to my newsletter
Read articles from Abhi Jain directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by
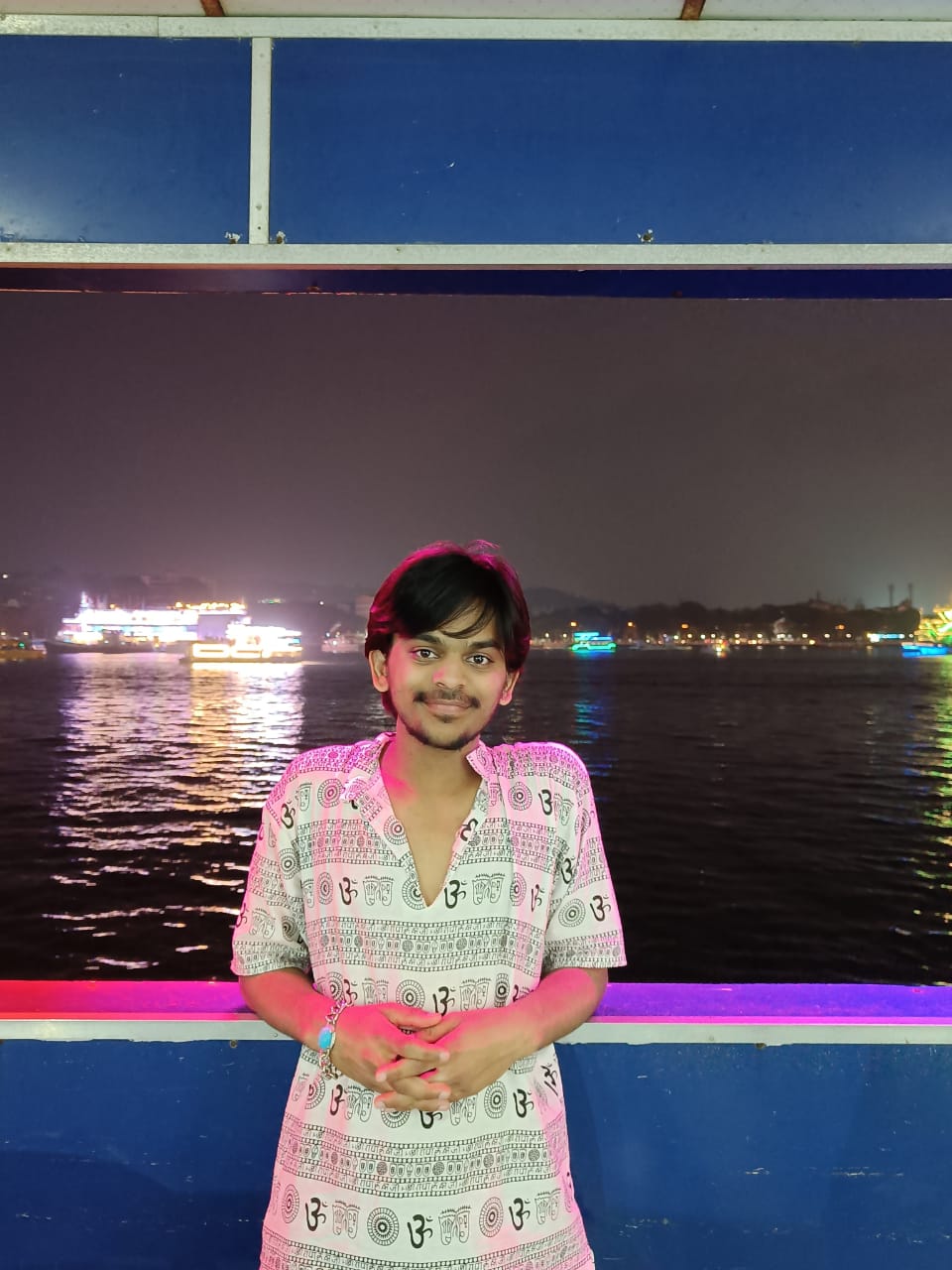
Abhi Jain
Abhi Jain
I am a full-stack web and app developer from India. Love building and collaborating software which solves problem.