The Spread Syntax
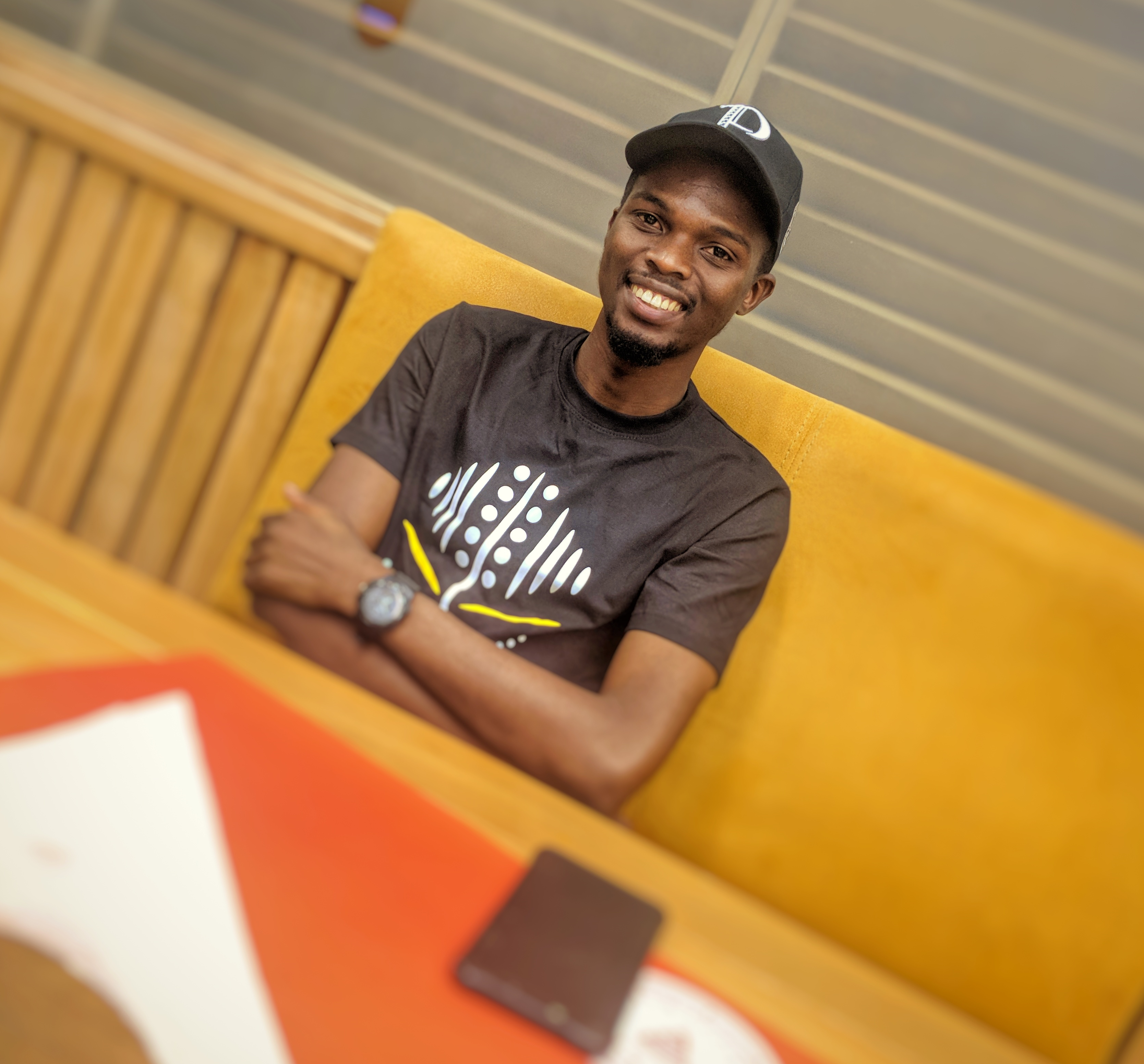
Table of contents
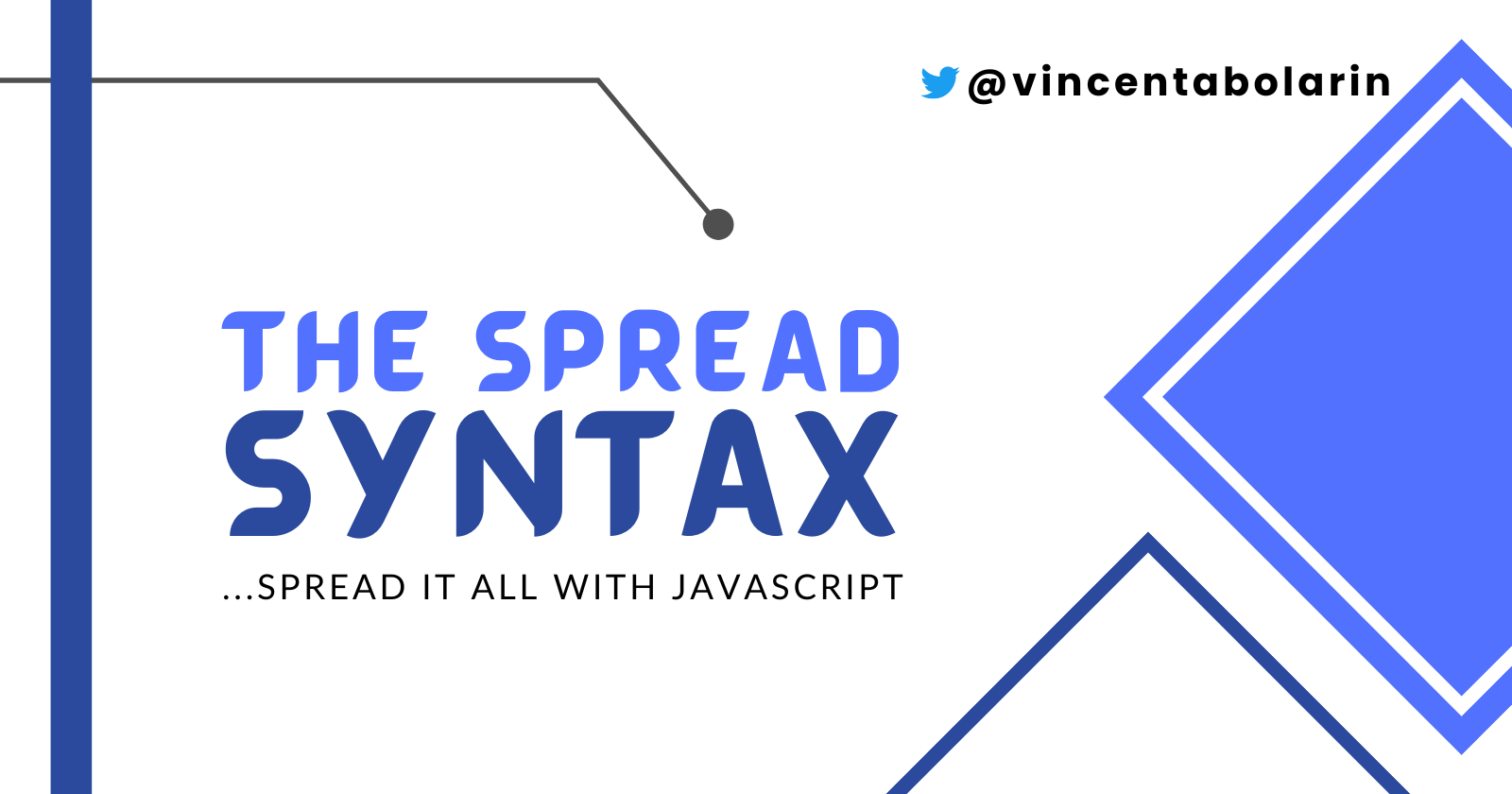
The spread syntax (...
) in JavaScript does not get as much recognition as it should. This is because when it comes to arrays (where this syntax is very important), a lot of people simply focus on array methods such as filter, map, and others. However, when you start building complex applications, you will begin to see how important the spread syntax is in JavaScript.
Now, if you have seen the codebase for large applications, there is a good chance that you have seen the spread syntax in use. You probably simply overlooked it because you did not know its use.
What is the Spread Syntax?
According to MDN Web Docs:
The spread (
...
) syntax allows an iterable, such as an array or string, to be expanded in places where zero or more arguments (for function calls) or elements (for array literals) are expected. In an object literal, the spread syntax enumerates the properties of an object and adds the key-value pairs to the object being created.
What this means in essence is that the spread syntax allows us to quickly copy all or part of an existing array or object into another array or object.
Uses of the Spread Syntax
To create a copy of an array.
const array1 = [1, 2, 3, 4, 5]; const array2 = [...array1]; // creates a copy of array1 console.log(array1); // Output: [1, 2, 3, 4, 5] console.log(array2); // Output: [1, 2, 3, 4, 5]
To concatenate two or more arrays.
const array1 = [1, 2, 3]; const array2 = [4, 5]; const array3 = [...array1, ...array2]; // concatenates array1 and array2 console.log(array3); // Output: [1, 2, 3, 4, 5]
To pass the items of an array as individual arguments to a function.
const numbers = [1, 2, 3, 4, 5]; const sum = (a, b, c, d, e) => a + b + c + d + e; const result = sum(...numbers); // passes the items in the numbers array as individual arguments console.log(result); // Output: 15
To convert a nodeList to an array. The number of items in the array depends on the number of items in the nodeList object.
const nodeList = document.querySelectorAll('p'); // returns a nodeList const nodeListArray = [...nodeList]; //converts the nodeList object into an array console.log(nodeListArray); // Output: [p, p, p, p, p]
To combine two objects
const someDetails = { firstName: "Vincent", lastName: "Abolarin", }; const moreDetails = { occupation: "Software Engineer" }; const allDetails = { ...someDetails, ...moreDetails } console.log(allDetails); // Output: { // firstName: 'Vincent', // lastName: 'Abolarin', // occupation: 'Software Engineer' // }
Of course, there are very many other use cases of the spread syntax. The use cases are not limited to these. However, when you understand the basics of how it works, you will be able to apply it to whatever use case comes up.
Also, it's pretty clear that the use of the spread syntax is not limited to arrays; you can also use this syntax on functions. It makes a lot of things easier, and when the need arises to use it, you will be glad that you know how to.
You can check out my Twitter thread on this same topic:
Subscribe to my newsletter
Read articles from Vincent Abolarin directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by
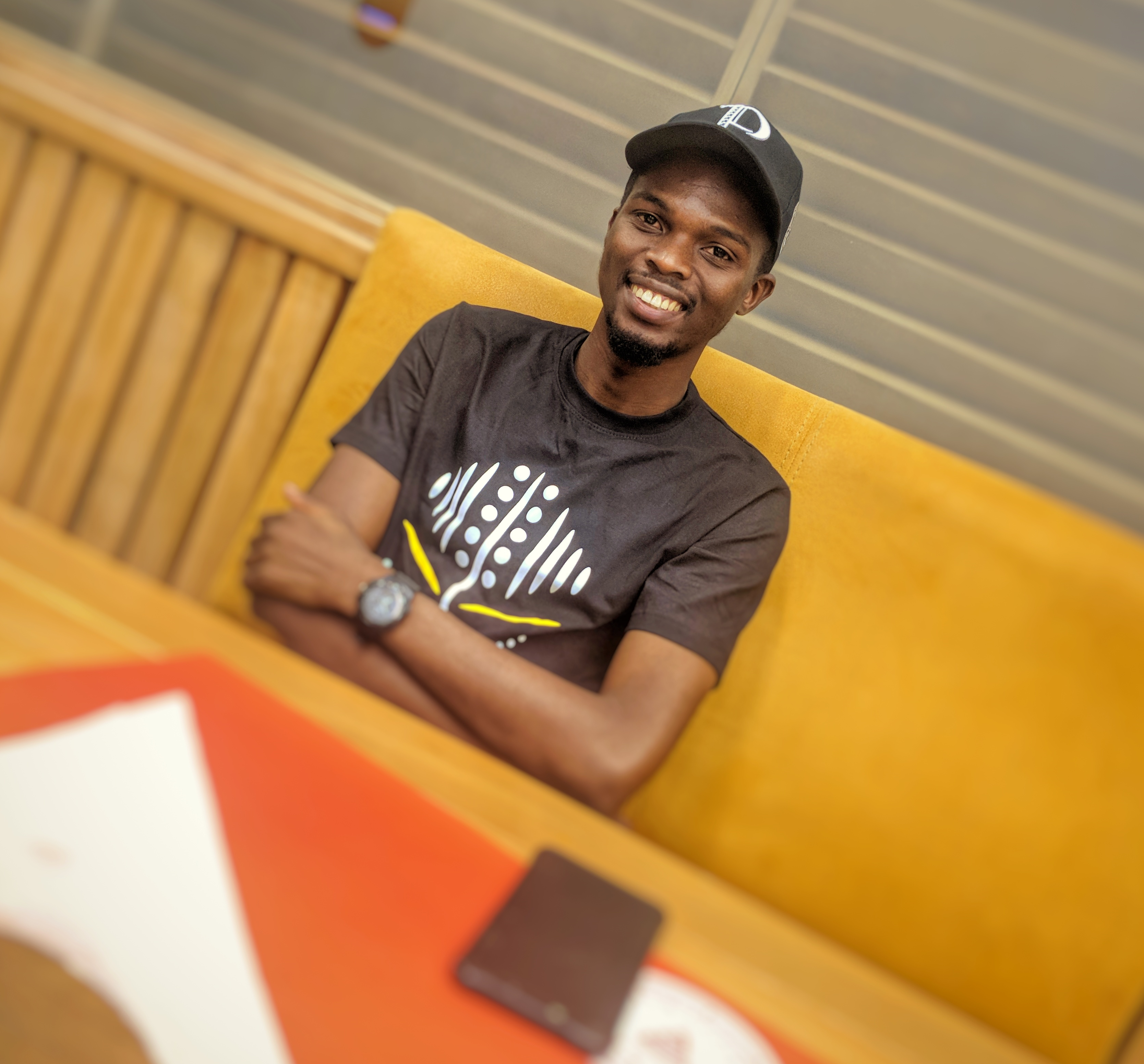