Simplifying Prop Management in React: How to Use the Provider Pattern for Clean and Scalable Code
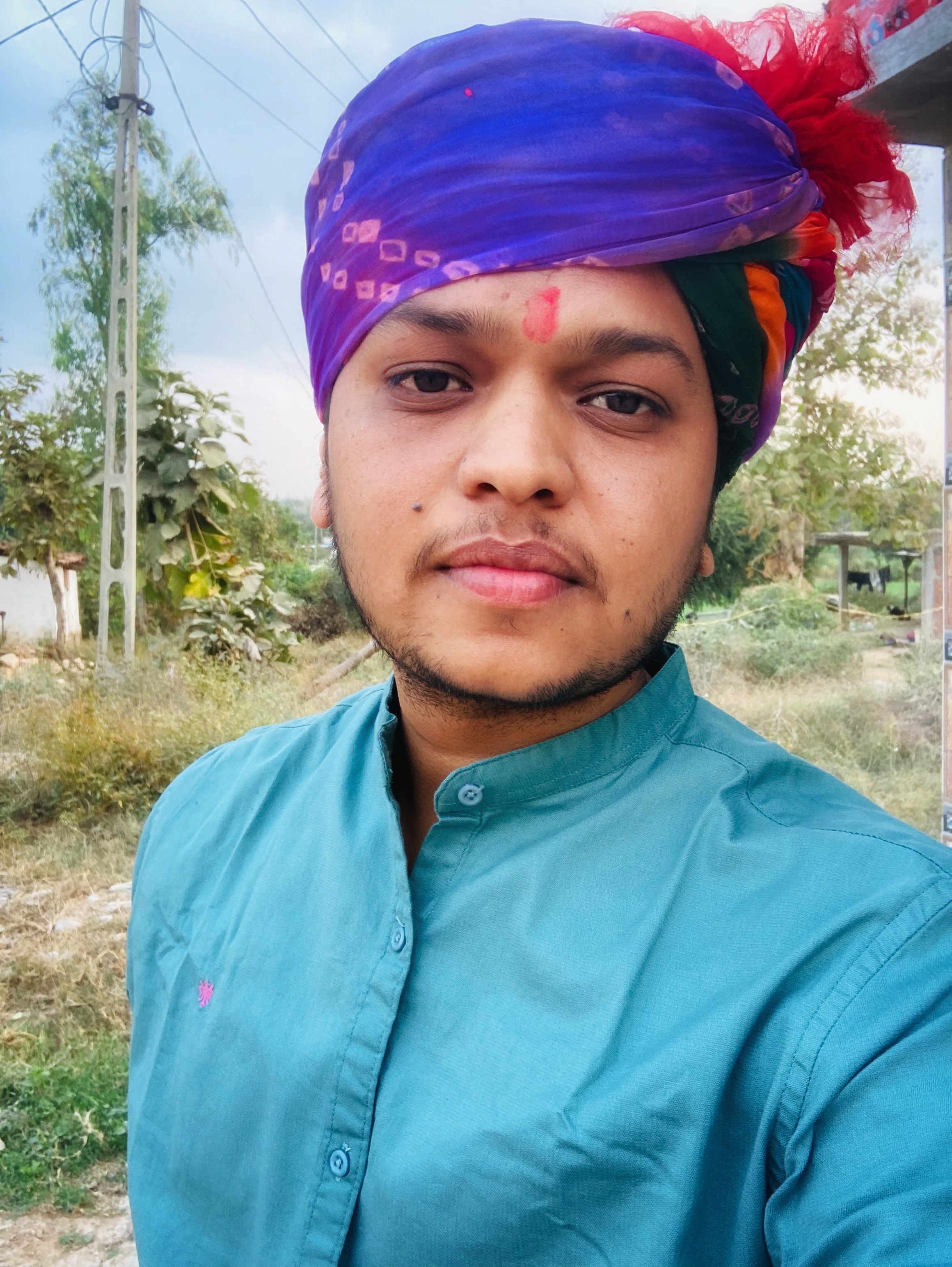
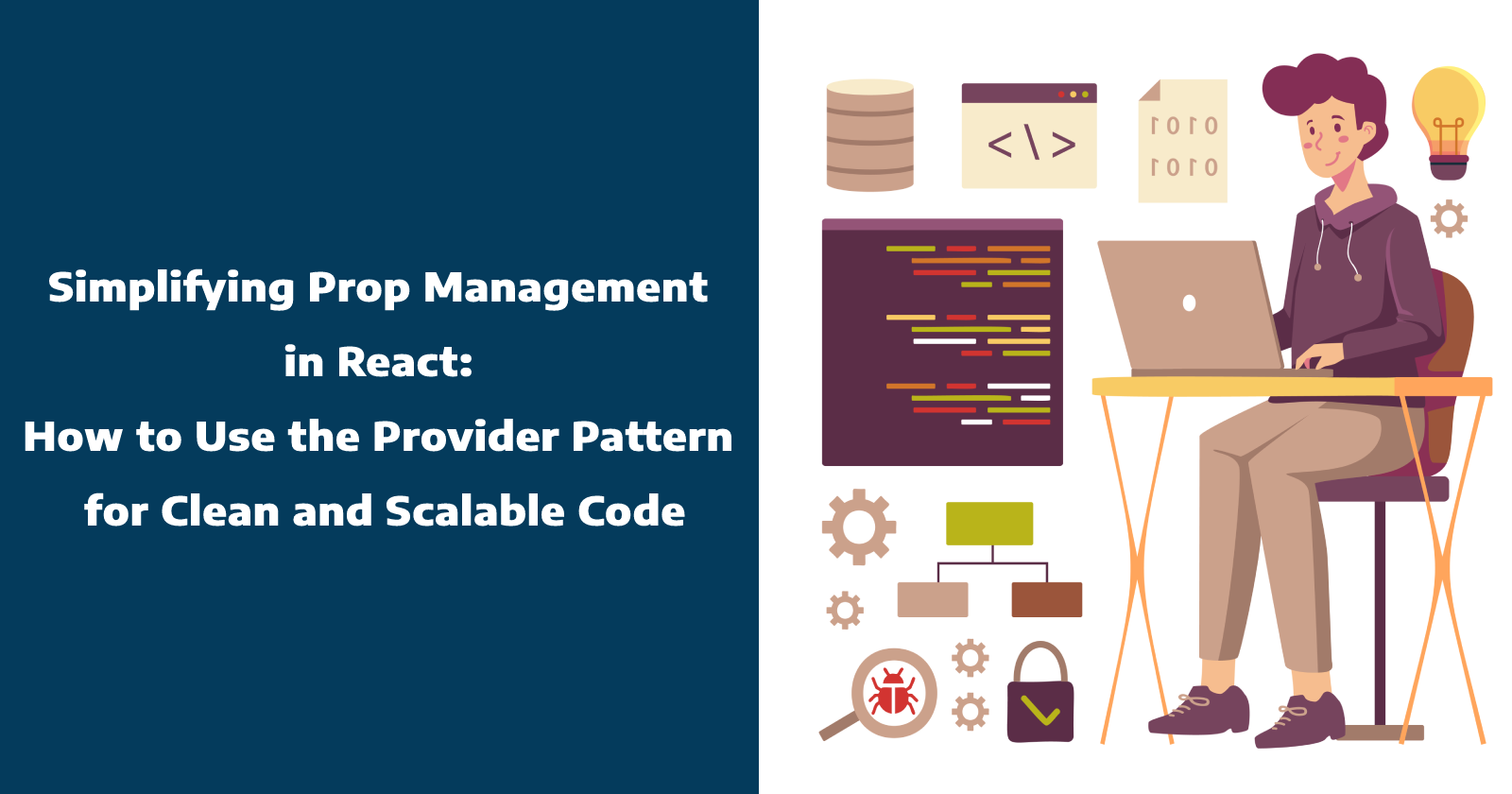
Introduction
In this article, we will explore and comprehend the Provider pattern. Design patterns offer solutions to frequently recurring problems in software design. It assists developers in creating software that is more flexible, extensible, and maintainable by offering time-tested solutions to common issues.
Prerequisites
Any programming language.
Basic programming concepts.
Object-oriented concepts.
Design pattern basics. (Good to know but, Not required)
What is the Provider Pattern?
Suppose we are implementing a Theme Toggle Switch, where users can switch themes between Dark Mode and Light Mode. Here, we want to make the toggle data available to all other components. We can pass data to all the children and grandchildren components using props, but this can be challenging if all components need this data to change the theme.
We often end up doing something called prop drilling, which means we pass the data from parent to child to grandchild to great-grandchild all the way down the component tree. This can impact the app's performance, and refactoring the code or changing prop names can be difficult.
This is where the Provider Pattern comes in. By using the Provider Pattern, we can make data available to multiple components. Instead of passing the data far down to components, we can wrap all the components in a Provider.
A Provider is a higher-order component provided to us by the Context
object. We can create a Context
object, using the createContext
method that React provides for us.
What kind of problem does the Provider Pattern solve?
With the Provider Pattern, we no longer need to pass data down to components through prop drilling. Instead, components can optionally consume the data, allowing for the distribution of data to multiple components without resorting to prop drilling.
This pattern also aids in managing the state in a scalable and efficient manner. It enables the centralization of shared data or state management within a single provider component, which can then supply that data to any component in the component tree, eliminating the need for prop drilling.
A real-world example of the Provider pattern in React might involve internationalization (i18n) and localization (l10n) within a multilingual application. In this scenario, the Provider pattern simplifies the management of language settings and translation data, removing this complexity from individual components.
This approach offers a clean and efficient way to handle i18n and l10n in a multilingual React application.
Now, let's examine the code aspect of how the Provider pattern functions.
Code Example Using React.js
import React from 'react'
import ReactDOM from 'react-dom'
import { Provider } from 'react-redux'
import store from './store'
import App from './App'
const otherElements = document.getElementById('root')
ReactDOM.render(
<Provider store={store}>
<App />
</Provider>,
<otherElements/>
)
You can refer to this simple app that demonstrates the use of the Provider component.
Here are some examples of frameworks or libraries that incorporate the Provider pattern:
React Context
MobX
Redux
Pros and Cons
Let's discuss the pros and cons of the Provider Pattern.
Pros
Provider Pattern reduces risk in state sharing and allows easy renaming and component reuse as the app grows.
Eliminates prop-drilling
The Provider pattern centralizes data management, ensuring consistent and reliable shared data across components.
Cons
Provider Pattern eliminates prop-drilling and centralizes data management, but may cause performance issues due to context-consuming components re-rendering on value changes.
The Provider pattern can be overused, causing unnecessary complexity.
Conclusion
In conclusion, the Provider pattern is a useful design pattern for managing shared data or state in a single location and making it available to multiple components without prop drilling.
It offers a scalable and efficient way to manage state and helps maintain consistency and integrity of shared data across components. While there are some potential performance issues and the risk of overusing the pattern, the benefits outweigh the drawbacks.
Overall, the Provider pattern is a valuable tool for creating flexible, extensible, and maintainable react apps.
Thank you for reading my article. Iām going to share more tech content soon. So, Please Follow Me, Feel free to comment and share your suggestions/thoughts.
Also, Follow me on Twitter @asenseofpradhyu. I share daily Cloud, System Design, Code, and many more tech stuff š
Resources
Subscribe to my newsletter
Read articles from Pradhumansinh Padhiyar directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by
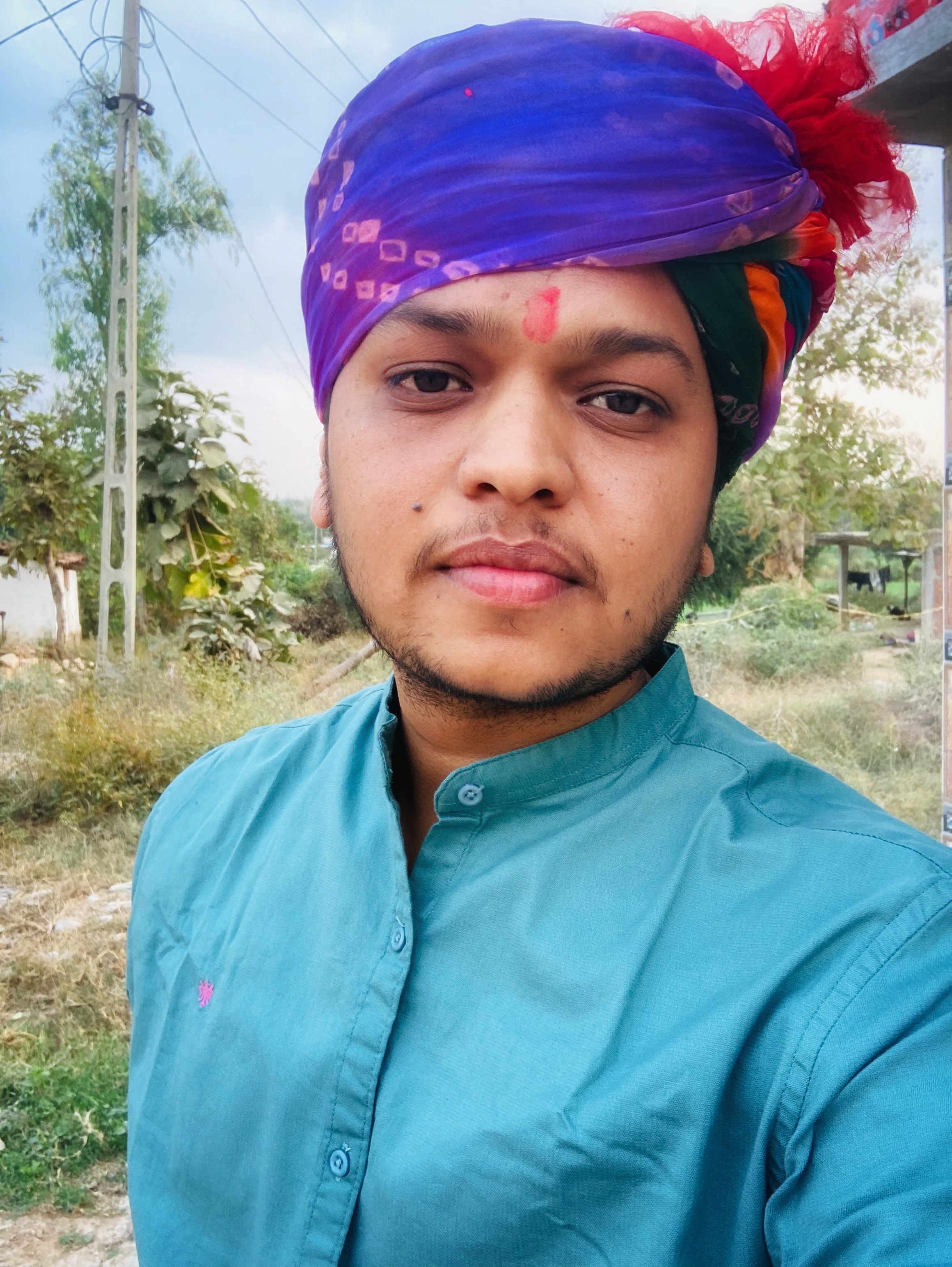
Pradhumansinh Padhiyar
Pradhumansinh Padhiyar
Sharing what I learn about Cloud, System design, and Code ⢠On my journey to becoming a certified AWS Dev ⢠Writing ebook on System Design